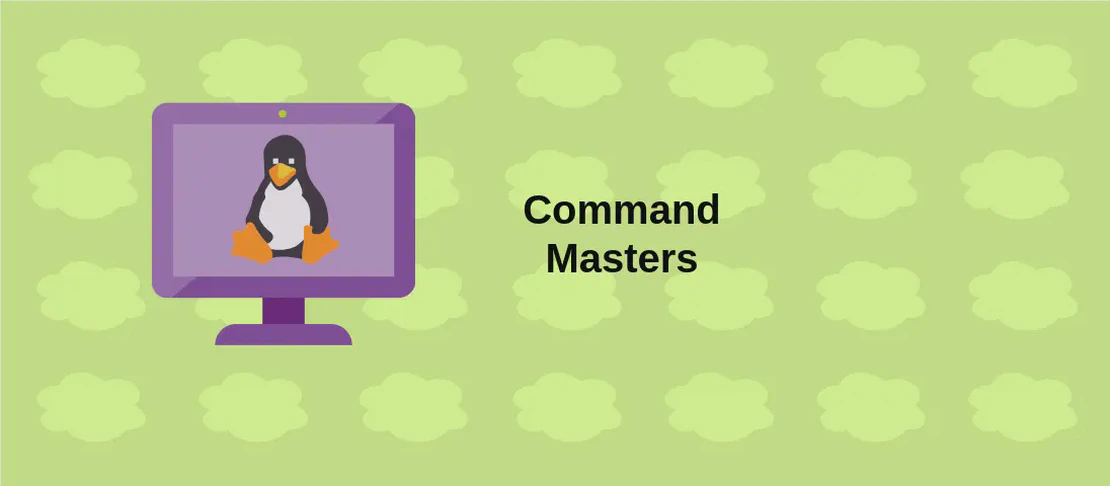
Mastering HTTPie Command-line Tool (with examples)
HTTPie is a user-friendly and powerful command-line HTTP client designed for testing, debugging, and interacting with web APIs and HTTP servers. It simplifies sending HTTP requests and offers an intuitive syntax. With a human-readable output, HTTPie helps developers quickly diagnose issues and seamlessly interact with their applications’ REST APIs.
Use case 1: Make a Simple GET Request
Code:
http https://example.com
Motivation: At its core, the simplest way to use the HTTPie tool is to perform a GET request to check if a specific server is reachable and to view the response it provides. This can be particularly useful when testing new endpoints, ensuring that a service is up, or when verifying the structure and content of the data returned from a server.
Explanation:
http
: The command that initiates an HTTP request using HTTPie.https://example.com
: The URL to which the GET request is sent. In this case,https://example.com
is used as a placeholder for any server or service you wish to reach.
Example Output: The output will show the HTTP response headers followed by the response body of the specified URL, allowing you to visually confirm both the metadata and content delivered by the server.
Use case 2: Print Specific Parts of the Content
Code:
http --print Hh https://example.com
Motivation: In many cases, developers or testers might not need the complete HTTP response but are only interested in specific components, such as headers or just the body. This command allows detailed customization of what parts of the request and response are displayed, improving readability and relevance of the output.
Explanation:
--print
: An option to specify which parts of the HTTP exchange should be printed.Hh
: Indicates that both the request headers and response headers should be printed.
Example Output: This output will include only the request and response headers, making it ideal if you need to troubleshoot header-related issues.
Use case 3: Specify the HTTP Method and Use a Proxy
Code:
http POST --proxy http:http://localhost:8080 https://example.com
Motivation: There are scenarios when developers need to test endpoints with different HTTP methods, like POST or PUT, rather than the default GET. Also, adding a proxy is essential when requests need to be monitored for debugging or routed through a specific proxy server for compliance with network security policies.
Explanation:
POST
: The HTTP method used to send data to the server.--proxy http:http://localhost:8080
: Specifies the use of an HTTP proxy server located atlocalhost
port8080
.
Example Output: The response will be indicative of a POST request. If successful, it often results in a status code like 201 (Created), and the request will have passed through the defined proxy.
Use case 4: Follow Redirects and Specify Additional Headers
Code:
http --follow https://example.com 'User-Agent: Mozilla/5.0' 'Accept-Encoding: gzip'
Motivation: Web developers frequently encounter redirects, especially when testing connections to endpoints that have moved or are under maintenance. The ability to follow these automatically and add custom headers (like User-Agent) is critical when tailoring requests to mimic specific conditions, like rendering different content based on the request headers.
Explanation:
--follow
: Allows the request to automatically follow any redirects (3xx status codes).'User-Agent: Mozilla/5.0'
: Sets the User-Agent header to mimic a request coming from a Mozilla Firefox browser.'Accept-Encoding: gzip'
: Requests a compressed response to save bandwidth.
Example Output: This will display the final response after following any redirects and applying the specified headers, showing the content designed for clients like Firefox.
Use case 5: Authenticate to a Server Using Different Authentication Methods
Code:
http --auth username:password --auth-type basic GET https://example.com/auth
Motivation: Authentication is crucial when interacting with restricted APIs or protected resources. Whether using basic, digest, or token-based authentication, ensuring your requests include the necessary credentials is key to accessing and manipulating the desired data securely.
Explanation:
--auth username:password
: Supplies the necessary credentials for authentication with the server.--auth-type basic
: Specifies the use of the basic authentication method.
Example Output: The response will be a successful message if the provided credentials are valid, often accompanied by a welcome or authentication-confirmed message in the body.
Use case 6: Construct a Request without Sending It
Code:
http --offline GET https://example.com
Motivation: Creating a request without sending is extremely useful for verifying request syntax, ensuring header correctness, or prepping reusable requests without risking interaction with live systems. This dry-run ability aids in thorough testing and development processes.
Explanation:
--offline
: Constructs and prints the request without actually sending it.GET
: Specifies the HTTP method for the simulated request.
Example Output: The output will include the full request as it would be sent, allowing you to review it for any errors before execution.
Use case 7: Use Named Sessions for Persistent Custom Headers, Auth Credentials, and Cookies
Code:
http --session my_session --auth username:password https://example.com/auth API-KEY:xxx
Motivation: Session persistence is vital when working with APIs requiring repeated authentication or interaction within a timeframe, eliminating redundancy by storing and re-using session data. This promotes efficiency, especially when needing to maintain a state across multiple requests.
Explanation:
--session my_session
: Initiates or continues using a named session calledmy_session
.--auth username:password
: Provides authentication credentials that are stored in the session.API-KEY:xxx
: Sets a persistent custom header, perhaps needed for ongoing communication with an API.
Example Output: The request will initiate a session, and the output will reflect a session-authenticated interaction, demonstrating that repeated setup processes can be avoided in subsequent requests.
Use case 8: Upload a File to a Form
Code:
http --form POST https://example.com/upload cv@path/to/file
Motivation: File uploads are a staple in web applications, from form submissions to media sharing. Testing this capability using the HTTPie tool allows developers to simulate and validate the upload process without needing a full client application, increasing the flexibility of integration tests.
Explanation:
--form
: Indicates that form-encoded data is being sent.POST
: The HTTP method used typically for submission of data including file uploads.cv@path/to/file
: Associates the file located atpath/to/file
with a form field namedcv
.
Example Output: A successful result would indicate that the file has been accepted by the server, possibly returning status 200 OK along with any message programmed to confirm receipt.
Conclusion:
The HTTPie command-line tool offers rich functionality for interacting with HTTP servers and APIs right from the terminal. Whether testing endpoints, ensuring proper authentication protocols, simulating proxy usage, or managing session persistence, HTTPie enhances workflow efficacy and debugging potential throughout a developer’s toolbox. With the diverse use cases described above, developers can confidently apply these techniques in various scenarios, streamlining and enhancing their API interactions.