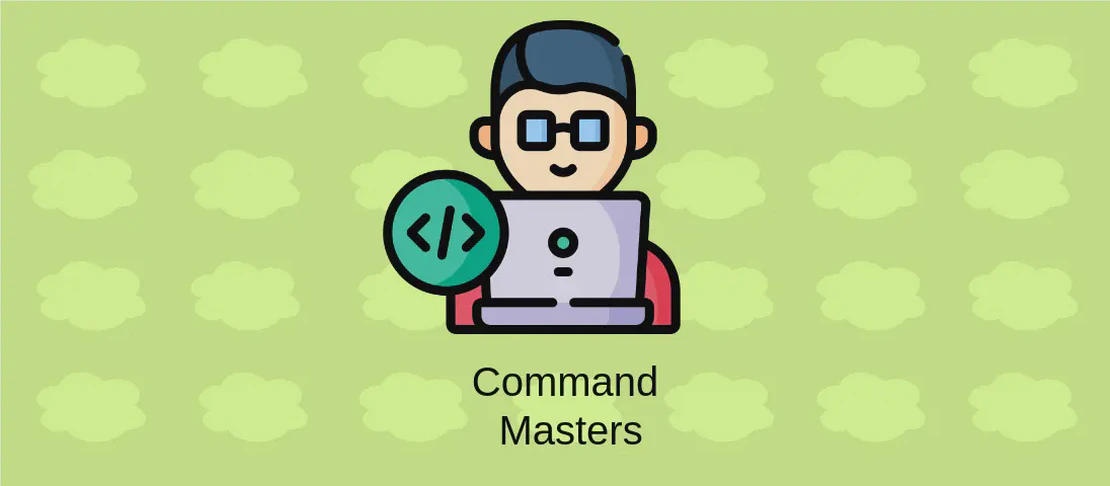
How to use the command "httpie" (with examples)
- Linux
- November 5, 2023
HTTPie is a user-friendly command-line tool used for sending HTTP requests. It simplifies the process of interacting with APIs and web services by providing a more user-friendly interface compared to cURL or other similar tools. In this article, we will explore several different use cases of the HTTPie command, along with code examples, motivations, explanations, and example outputs for each use case.
Sending a GET request
The most basic use case of HTTPie is sending a GET request to a URL. In this example, we will send a GET request to https://example.com
:
http https://example.com
Motivation: This use case is useful when we want to retrieve information from a server using a simple HTTP GET request. It can be used to check the status of a website, fetch API data, or access any publicly accessible resource.
Explanation: The http
command is followed by the URL we want to send the request to. In this case, we specify https://example.com
. HTTPie automatically assumes it’s a GET request since no other request method is specified.
Example output:
HTTP/1.1 200 OK
Content-Type: text/html
Date: Sat, 01 Jan 2022 12:00:00 GMT
Server: ExampleServer
<!doctype html>
<html>
<head>
<title>Example Domain</title>
...
</head>
<body>
<div>
<h1>This is an example website</h1>
...
</div>
</body>
</html>
The response includes the HTTP status code, headers, and the response body.
Sending a POST request
Another common use case of HTTPie is sending a POST request with request data. In this example, we will send a POST request to https://example.com
with a request data parameter called hello
set to the value “World”:
http https://example.com hello=World
Motivation: This use case is useful when we need to send data to a server using an HTTP POST request. It can be used for submitting forms, creating new resources, or sending JSON data to APIs.
Explanation: Similar to the previous example, we provide the URL as the argument to the http
command. Additionally, we include the request data as key-value pairs, separated by a =
sign.
Example output:
HTTP/1.1 200 OK
Content-Type: text/html
Date: Sat, 01 Jan 2022 12:00:00 GMT
Server: ExampleServer
<!doctype html>
<html>
<head>
<title>Example Domain</title>
...
</head>
<body>
<div>
<h1>Hello World!</h1>
...
</div>
</body>
</html>
The response will include the HTTP status code, headers, and the response body.
Sending a POST request with redirected input
With HTTPie, we can send a POST request with redirected input from a file. This can be useful when we have request data stored in a separate file, such as a JSON payload. In this example, we will send a POST request to https://example.com
with the contents of file.json
as the request payload:
http https://example.com < file.json
Motivation: This use case is helpful when we have a large request payload or multiple parameters that would be difficult to include on the command line. By redirecting the input from a file, we can easily send the contents of the file as the request payload.
Explanation: The <
operator is used to redirect the input from the file file.json
to the http
command. The contents of the file will be sent as the request payload.
Example output:
HTTP/1.1 200 OK
Content-Type: application/json
Date: Sat, 01 Jan 2022 12:00:00 GMT
Server: ExampleServer
{
"message": "Request processed successfully.",
...
}
The response will include the HTTP status code, headers, and the response body, which, in this case, is a JSON object.
Sending a PUT request with a given JSON body
HTTPie also supports sending PUT requests with a JSON body. This is useful when we need to update an existing resource on a server. In this example, we will send a PUT request to https://example.com/todos/7
with a JSON body containing a parameter called hello
set to the value “world”:
http PUT https://example.com/todos/7 hello=world
Motivation: This use case is applicable when we want to update an existing resource on a server. PUT requests are commonly used for updating API data or modifying specific items in a system.
Explanation: The http
command is followed by the HTTP method PUT
and the URL https://example.com/todos/7
. We also include the request data as key-value pairs, separated by a =
sign.
Example output:
HTTP/1.1 204 No Content
Content-Type: text/html; charset=utf-8
Date: Sat, 01 Jan 2022 12:00:00 GMT
Server: ExampleServer
...
The response will include the HTTP status code and headers. In this case, there is no response body (No Content
).
Sending a DELETE request with a given request header
HTTPie allows us to send DELETE requests with custom request headers. This can be useful when we need to provide additional information in the request, such as an API key or authentication token. In this example, we will send a DELETE request to https://example.com/todos/7
with a request header API-Key
set to “foo”:
http DELETE https://example.com/todos/7 API-Key:foo
Motivation: This use case is relevant when we want to delete a resource on a server. DELETE requests are commonly used for removing data from APIs or deleting records in a system.
Explanation: The http
command is followed by the HTTP method DELETE
and the URL https://example.com/todos/7
. Additionally, we include the request header API-Key
with the value “foo” using the format HeaderName:HeaderValue
.
Example output:
HTTP/1.1 204 No Content
Content-Type: text/html; charset=utf-8
Date: Sat, 01 Jan 2022 12:00:00 GMT
Server: ExampleServer
...
The response will include the HTTP status code and headers. In this case, there is no response body (No Content
).
Show the whole HTTP exchange
HTTPie allows us to display the complete HTTP exchange, including both the request and response. This can be useful for debugging or understanding the underlying HTTP communication. In this example, we will use the -v
option to show the whole HTTP exchange when sending a GET request to https://example.com
:
http -v https://example.com
Motivation: This use case is valuable when we need to inspect the complete HTTP exchange to troubleshoot issues, analyze network traffic, or understand the details of the request and response.
Explanation: The http
command is followed by the -v
option, which enables verbose mode. This mode displays the complete HTTP exchange, including request and response headers.
Example output:
GET / HTTP/1.1
Accept: */*
Accept-Encoding: gzip, deflate
Connection: keep-alive
Host: example.com
HTTP/1.1 200 OK
Cache-Control: max-age=604800
Content-Type: text/html
Date: Sat, 01 Jan 2022 12:00:00 GMT
...
<!doctype html>
<html>
<head>
<title>Example Domain</title>
...
</head>
<body>
<div>
<h1>This is an example website</h1>
...
</div>
</body>
</html>
The output includes the complete raw HTTP request and response, including headers and the response body.
Download a file
HTTPie allows us to easily download files from a URL. This is useful when we want to retrieve a file from a server or save it locally. In this example, we will download a file from https://example.com
:
http --download https://example.com
Motivation: This use case is handy when we need to retrieve a file from a server without relying on a full-fledged download manager or web browser. It provides a simple command-line option to download a file directly.
Explanation: The --download
option is appended to the http
command, followed by the URL. This option instructs HTTPie to download the file rather than displaying the response contents.
Example output: The downloaded file will be saved in the current working directory with the same name as the original file. If the original file is example.html
, it will be saved as example.html
locally.
Follow redirects and show intermediary requests and responses
HTTPie allows us to follow redirects and display intermediary requests and responses. This is useful when we want to inspect the entire redirect chain and understand how the request is being processed. In this example, we will use the --follow
and --all
options when sending a GET request to https://example.com
:
http --follow --all https://example.com
Motivation: This use case is valuable when we need to observe the complete chain of redirects during a request. It provides a way to understand how the server processes the initial request and handle redirects.
Explanation: The --follow
option enables following redirects, while the --all
option instructs HTTPie to display intermediary requests and responses. These options combine to show the entire redirect chain.
Example output: The output will include multiple sets of request and response information, each representing a step in the redirect chain, starting from the initial request and ending with the final response.
Request 1/3: GET / HTTP/1.1
...
Response 1/3: 301 Moved Permanently
...
Request 2/3: GET /new-page HTTP/1.1
...
Response 2/3: 302 Found
...
Request 3/3: GET /final-page HTTP/1.1
...
Response 3/3: 200 OK
...
The output will include details for each request and response, including HTTP status codes, headers, and response bodies.
Conclusion
HTTPie is a versatile command-line tool for sending HTTP requests. It provides an intuitive and user-friendly interface that simplifies the process of interacting with APIs and web services. By exploring the different use cases and code examples in this article, you can gain a better understanding of how to use HTTPie effectively in your own projects. Whether you need to send GET, POST, PUT, or DELETE requests, follow redirects, download files, or inspect the complete HTTP exchange, HTTPie has got you covered. Try it out and see how it can streamline your HTTP request workflows.