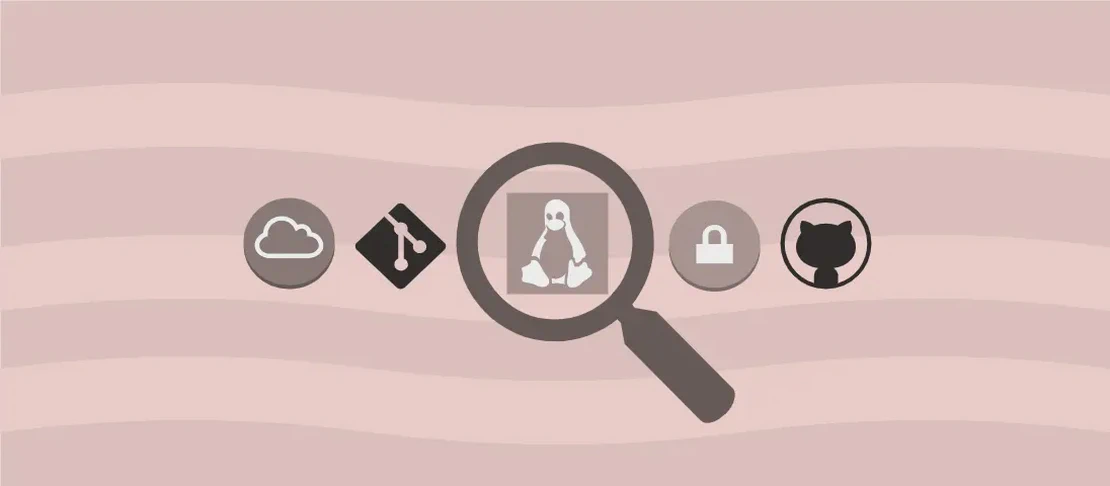
How to use the command 'if' (with examples)
The ‘if’ command is used to perform conditional processing in shell scripts. It allows you to execute a set of commands based on the outcome of a condition command. The ‘if’ command is often used in conjunction with the ’test’ command or its alias ‘[’ to evaluate conditions. By using the ‘if’ command, you can control the flow of your script and make decisions based on certain conditions.
Use case 1: Execute the specified commands if the condition command’s exit status is zero
Code:
if condition_command; then
echo "Condition is true"
fi
Motivation: This use case enables you to execute a set of commands only if the condition command’s exit status is zero. It is useful when you want to perform certain actions based on the success of a previous command or operation.
Explanation:
- ‘if’ is the keyword that indicates the start of the conditional execution block.
- ‘condition_command’ is the command that is being evaluated. If its exit status is zero (success), the specified commands within the ’then’ block will be executed.
- ’then’ indicates the start of the block of commands to be executed if the condition is true.
- ’echo’ is the command used to print the message “Condition is true”.
- ‘fi’ denotes the end of the conditional execution block.
Example output: If the condition command’s exit status is zero (success), the message “Condition is true” will be printed.
Use case 2: Execute the specified commands if the condition command’s exit status is not zero
Code:
if ! condition_command; then
echo "Condition is true"
fi
Motivation: This use case allows you to execute a set of commands only if the condition command’s exit status is not zero. It can be used when you want to handle any errors or failures indicated by a non-zero exit status.
Explanation:
- In this case, the ‘!’ operator negates the exit status of the ‘condition_command’. If the condition command’s exit status is not zero, meaning it indicates failure, the specified commands within the ’then’ block will be executed.
- The rest of the structure is the same as explained in the previous use case.
Example output: If the condition command’s exit status is not zero (failure), the message “Condition is true” will be printed.
Use case 3: Execute the first specified commands if the condition command’s exit status is zero, otherwise execute the second specified commands
Code:
if condition_command; then
echo "Condition is true"
else
echo "Condition is false"
fi
Motivation: This use case allows you to handle different outcomes based on the condition command’s exit status. It provides a way to execute one set of commands when the condition is true and another set of commands when the condition is false.
Explanation:
- In this case, the ‘if’ block is extended to include an ’else’ block. If the condition command’s exit status is zero (success), the specified commands within the ’then’ block will be executed. If the condition command’s exit status is not zero (failure), the specified commands within the ’else’ block will be executed.
- The message “Condition is true” is printed when the condition is true, and “Condition is false” is printed when the condition is false.
- ’else’ denotes the start of the block of commands to be executed if the condition is false.
- ‘fi’ denotes the end of the conditional execution block.
Example output: If the condition command’s exit status is zero (success), the message “Condition is true” will be printed. If the condition command’s exit status is not zero (failure), the message “Condition is false” will be printed.
Use case 4: Check whether a file exists
Code:
if [[ -f path/to/file ]]; then
echo "Condition is true"
fi
Motivation: This use case allows you to check the existence of a file before performing further actions. It is particularly useful when you want to conditionally execute commands based on the presence or absence of a specific file.
Explanation:
- In this case, ‘-f’ is the file test operator used within the ‘[[’ and ‘]]’ brackets to test if the given path is a regular file.
- When the condition is true (the file exists), the specified commands within the ’then’ block will be executed.
- The rest of the structure is the same as explained in the first use case.
Example output: If the file exists at the specified path, the message “Condition is true” will be printed.
Use case 5: Check whether a directory exists
Code:
if [[ -d path/to/directory ]]; then
echo "Condition is true"
fi
Motivation: This use case allows you to check the existence of a directory before proceeding with specific operations. It helps in conditionally executing commands based on the presence or absence of a particular directory.
Explanation:
- In this case, ‘-d’ is the directory test operator used within the ‘[[’ and ‘]]’ brackets to test if the given path is a directory.
- When the condition is true (the directory exists), the specified commands within the ’then’ block will be executed.
- The rest of the structure is the same as explained in the first use case.
Example output: If the directory exists at the specified path, the message “Condition is true” will be printed.
Use case 6: Check whether a file or directory exists
Code:
if [[ -e path/to/file_or_directory ]]; then
echo "Condition is true"
fi
Motivation: This use case allows you to check the existence of a file or directory using a single condition. It is useful when you want to conditionally execute commands based on the presence or absence of a file or directory.
Explanation:
- In this case, ‘-e’ is the existence test operator used within the ‘[[’ and ‘]]’ brackets to test if the given path exists.
- When the condition is true (the file or directory exists), the specified commands within the ’then’ block will be executed.
- The rest of the structure is the same as explained in the first use case.
Example output: If the file or directory exists at the specified path, the message “Condition is true” will be printed.
Use case 7: Check whether a variable is defined
Code:
if [[ -n "$variable" ]]; then
echo "Condition is true"
fi
Motivation: This use case allows you to check whether a variable is defined before using it in further operations. It is useful when you want to conditionally execute commands based on the presence or absence of a variable’s value.
Explanation:
- In this case, ‘-n’ is the non-empty test operator used within the ‘[[’ and ‘]]’ brackets to test if the given variable has a non-empty value.
- When the condition is true (the variable is defined and has a non-empty value), the specified commands within the ’then’ block will be executed.
- The rest of the structure is the same as explained in the first use case.
Example output: If the variable is defined and has a non-empty value, the message “Condition is true” will be printed.
Use case 8: List all possible conditions
Code:
man [
Motivation: This use case allows you to explore all the possible conditions that can be used with the ‘if’ command. By referring to the manual page of the ’test’ command, which is the same as ‘[’, you can find a comprehensive list of available conditions and their usage.
Explanation:
- Running ‘man [’ in the terminal will open the manual page for the ’test’ command, which is an alias to ‘[’.
- The manual page provides detailed documentation on various test operators and their syntax. It explains how to use them to evaluate conditions in the ‘if’ command.
Example output: The manual page of the ’test’ command, listing all possible conditions and their usage, will be displayed in the terminal.
Conclusion:
The ‘if’ command is a powerful tool for performing conditional processing in shell scripts. It allows you to control the flow of your script and make decisions based on conditions. By mastering the various use cases of the ‘if’ command and understanding the different conditions that can be tested, you can write more robust and flexible shell scripts.