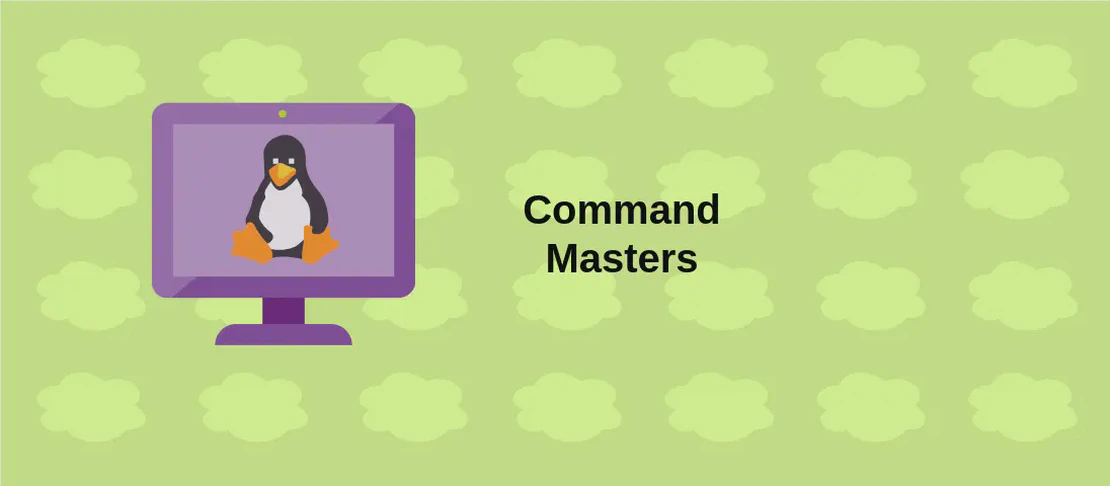
How to Use the 'if' Command in Batch Scripts (with examples)
- Windows
- December 17, 2024
Batch scripting in Windows often relies on conditional statements to determine the flow of execution. One of the core commands used for this purpose is if
, which allows scripts to perform different actions based on conditions. This command is crucial for creating more dynamic and responsive scripts. Here, we explore various use cases of the if
command with examples to illustrate its versatility in handling conditions.
Use Case 1: Execute the specified commands if the condition is true
Code:
if condition (echo Condition is true)
Motivation:
This is one of the fundamental uses of the if
command. It’s particularly useful when you need to perform an action only when a specific condition holds true. For instance, in automated deployment scripts, you might need to check if a service is running before attempting to start it, thus avoiding unnecessary operations.
Explanation:
if
is the command that initiates the conditional check.condition
represents the logical condition that needs evaluation (e.g., checking the state of a file or variable).(echo Condition is true)
specifies the command that runs if the condition evaluates to true. In this example, the script outputs a confirmation message.
Example Output:
Condition is true
Use Case 2: Execute the specified commands if the condition is false
Code:
if not condition (echo Condition is true)
Motivation:
This use case is critical when you need to ensure that an operation occurs only when a condition is false. Such checks are common in troubleshooting scripts where you want to know if a service is not running and require starting.
Explanation:
if not
indicates that the subsequent command block should run if the condition evaluates to false.condition
is the logical check, similar to the first example.(echo Condition is true)
executes if the condition is false, typically used to handle the opposite logic of what is expected.
Example Output:
Condition is true
Use Case 3: Execute the first specified commands if the condition is true otherwise execute the second specified commands
Code:
if condition (echo Condition is true) else (echo Condition is false)
Motivation:
This approach is beneficial when you need dual pathways within your script, effectively allowing you to handle both true and false conditions explicitly. This duality is often required in decision-making scripts, such as installing updates only if they are not present, or logging errors otherwise.
Explanation:
if condition
initiates the primary condition check.(echo Condition is true)
specifies the command executed if the condition is true.else
provides an alternative pathway if the condition is not met.(echo Condition is false)
executes if the initial condition is false, offering different outputs for both scenarios.
Example Output:
Condition is true
or
Condition is false
Use Case 4: Check whether %errorlevel%
is greater than or equal to the specified exit code
Code:
if errorlevel 2 (echo Condition is true)
Motivation:
%errorlevel%
is a pre-defined variable that stores the exit code of the last executed command. This check is vital for handling errors and taking corrective actions in scripts, such as retrying a failed download or stopping further execution unless a success code is returned.
Explanation:
if errorlevel 2
checks if the last executed command’s exit code is 2 or greater.(echo Condition is true)
is the command block that executes if the condition holds, often used for logging or error handling.
Example Output:
Condition is true
Use Case 5: Check whether two strings are equal
Code:
if %variable% == string (echo Condition is true)
Motivation:
String comparison is a common task in scripting, particularly for validation purposes like verifying user input or checking the output of a function. This use case enables scripts to proceed based on exact string matches.
Explanation:
if %variable% == string
compares the content of%variable%
to the specifiedstring
.(echo Condition is true)
runs if both strings are equivalent, used for validation checks.
Example Output:
Condition is true
Use Case 6: Check whether two strings are equal without respecting letter case
Code:
if /i %variable% == string (echo Condition is true)
Motivation:
In many situations, case-insensitivity is desired, such as user-defined settings or arguments passed to a script. This option avoids unnecessary failure due to case discrepancies, making scripts more robust.
Explanation:
if /i
enables case-insensitive string comparison.%variable% == string
functions as in the prior example, performing a case-insensitive comparison.(echo Condition is true)
executes upon a match, accommodating different capitalization.
Example Output:
Condition is true
Use Case 7: Check whether a file exists
Code:
if exist path\to\file (echo Condition is true)
Motivation:
Existence checks are a cornerstone of file management in scripts. Before performing actions like reading from, writing to, or deleting a file, confirming its presence prevents errors and ensures the reliability of script operations.
Explanation:
if exist
verifies if a specified file is present in the directory.path\to\file
defines the file path relative to the current directory or an absolute file path.(echo Condition is true)
is executed if the file exists, commonly used to confirm prerequisites or dependencies.
Example Output:
Condition is true
Conclusion:
The if
command is an indispensable tool in Windows batch scripting, serving as the foundation for constructing logic-driven workflows. By learning and utilizing its various forms and intricacies, users can create sophisticated scripts that adapt to different situations, enhancing automation and error handling capabilities.