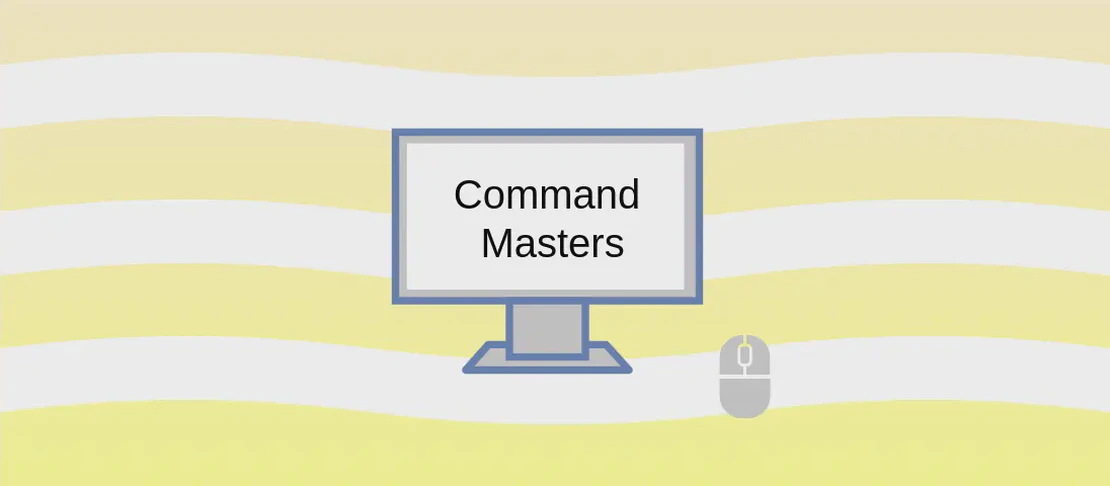
How to Use the Command 'indent' (with Examples)
- Osx
- December 17, 2024
The indent
command is a utility that formats and beautifies C and C++ source code files by adjusting whitespace. Its main function is to make code easier to read and to adhere to a particular coding style or standard, enhancing code uniformity across projects. The indent
command can alter a source file to fit various coding styles, such as Berkeley, Linux, or Kernighan & Ritchie, by adding or removing spaces and aligning text in a consistent manner.
Use Case 1: Format C/C++ Source According to the Berkeley Style
Code:
indent path/to/source_file.c path/to/indented_file.c -nbad -nbap -bc -br -c33 -cd33 -cdb -ce -ci4 -cli0 -di16 -fc1 -fcb -i4 -ip -l75 -lp -npcs -nprs -psl -sc -nsob -ts8
Motivation:
Adhering to a consistent code style is crucial for maintainability and readability, especially when collaborating with different team members on a project. The Berkeley style is known for its structured and visually appealing layout, which helps in easily navigating through complex codebases. Formatting code in this style can contribute significantly to reducing cognitive load and improving focus for developers.
Explanation:
-nbad
: Disables placing a newline after an assignment operator, keeping related elements on the same line.-nbap
: Disables blank lines after procedure bodies, maintaining code compactness.-bc
: Forces newline before a closing brace, promoting block visibility.-br
: Places braces ({
and}
) on the line following the statement, enhancing clarity.-c33
: Sets comment column width to 33, aligning comments neatly.-cd33
: Sets column offset for block comments to 33, creating an aligned structure.-cdb
: Places comment delimiters on blank lines, improving separation.-ce
: Moves C comments to the end of the line, maintaining focus on code.-ci4
: Indents continuation lines by four spaces, aligning multi-line code smoothly.-cli0
: Sets case label indentation to none, aligning switch-case constructs directly under the switch statement.-di16
: Sets variable declarations indentation to 16 spaces.-fc1
: Enables a newline at the start of functions (function newline
).-fcb
: Puts a newline after an opening brace, making the block start distinct.-i4
: Uses an indentation size of four spaces, which is common and readable.-ip
: Indents parameter declarations in function definitions, making them clearer.-l75
: Limits line length to 75 characters, enhancing readability.-lp
: Packs function calls, reducing multiple lines into fewer.-npcs
: Disables placing a space after a cast, keeping it visually tight.-nprs
: Disables placing a space around assignment operators.-psl
: Places comments on the line above the indented code.-sc
: Uses standard handle comments by default, standardizing their appearance.-nsob
: Disables swallowing optional blanks, retaining intended spacing.-ts8
: Sets tab stops every 8 spaces, standard for alignment with editors.
Example Output:
After applying these settings, the code will have a neat appearance with consistent indentation and well-aligned comments. Braces will follow the Berkeley style with clear separations for different code blocks, reducing visual clutter and improving overall code clarity.
Use Case 2: Format C/C++ Source According to the Style of Kernighan & Ritchie (K&R)
Code:
indent path/to/source_file.c path/to/indented_file.c -nbad -bap -nbc -br -c33 -cd33 -ncdb -ce -ci4 -cli0 -cs -d0 -di1 -nfc1 -nfcb -i4 -nip -l75 -lp -npcs -nprs -npsl -nsc -nsob
Motivation:
The Kernighan & Ritchie (K&R) style is one of the most widely respected and followed coding styles. It originated from the authors of the C programming language themselves and is known for its readability and minimalist approach. Utilizing the indent
command to format the code in K&R style ensures that codebases maintain a clean and professional look, aiding in better understanding and reducing confusion among developers.
Explanation:
-nbad
: Avoids breaking after the assignment, keeping variable assignments concise.-bap
: Inserts blank lines after procedure bodies, distinguishing different code sections.-nbc
: Disables breaking complex declarations, keeping them more succinct.-br
: Moves braces to a new line, standard for K&R style.-c33
: Positions comments at column 33, aligning text for easy readability.-cd33
: Moves block comments to column 33, following general code alignment.-ncdb
: Avoids blank lines for comment delimiters, making inline comments tighter.-ce
: Aligns comments at the end of the code line for direct reference.-ci4
: Indents continuation lines by four spaces for clearer multiple-line statements.-cli0
: Does not further indent case labels from the switch.-cs
: Includes space after cast operators which is a common practice for readability.-d0
: Sets a default value for indentation of declarations improving clarity.-di1
: Indentation of variable declarations by one space enhances clear grouping.-nfc1
: Avoids newline at function start, maintaining a condensed layout.-nfcb
: Does not force a newline after a function block, promoting a compact style.-i4
: Uses a four-space tab width facilitating consistency across different environments.-nip
: Avoids parameter indentation, preferring inline parameter listings.-l75
: Restricts line length to 75 characters for easier reading.-lp
: Packs lines, maintaining concise code.-npcs
: Avoids spaces around casts, keeping casts visually tighter.-nprs
: Neglects placing spaces around assignment operators.-npsl
: Positions comments beside code improving inline understanding.-nsc
: Skips standard comment formatting, relying on code clarity.-nsob
: Does not condense blank lines, maintaining developer’s intended spacing.
Example Output:
Applying K&R formatting results in a streamlined, easily navigable code structure where functions and statements are well-aligned yet moderately compact. This can facilitate faster comprehension and edits by developers familiar with this classic format.
Conclusion:
The indent
command serves as a powerful tool for enforcing coding styles and enhancing the readability of C/C++ source codes. Whether adopting the Berkeley style for its neatness or the K&R style for its simplicity and historical significance, indent
supports the maintainable development practices necessary for complex and collaborative projects. With these examples and explanations, developers can easily apply uniform coding styles, greatly improving their code’s quality and readability.