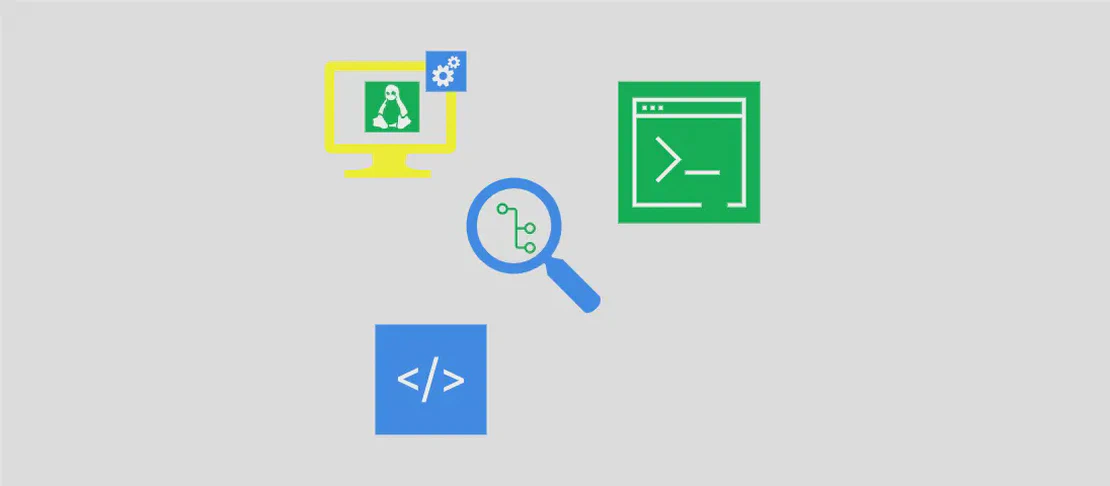
How to Send Input Events to an Android Device Using ADB (with examples)
- Android
- December 17, 2024
The input
command is a powerful tool that allows developers and testers to simulate user interactions with an Android device from the command line. It is part of Android Debug Bridge (ADB), which is a versatile command-line tool that lets you communicate with a device. The input
command can be used for a variety of purposes, such as testing app functionality, automating repetitive tasks, or simulating real user interactions for demonstration purposes. The command must be executed through adb shell
, which provides direct access to the device’s operating system.
Send an event code for a single character to an Android device
Code:
adb shell input keyevent event_code
Motivation:
One of the primary reasons to use this command is to simulate a key press on an Android device. This can be useful in testing scenarios where you need to replicate user actions without physically interacting with the device. For instance, if you’re testing an app’s response to hardware button presses (like the volume or home button), this command lets you simulate that interaction directly from your computer.
Explanation:
adb shell
: This part of the command opens a communication shell on the Android device.input
: This is the command used to simulate input events.keyevent
: This specifies the type of event you’re sending, which in this case is a key event.event_code
: Here, you would replaceevent_code
with the specific KeyEvent constant that represents the key you want to simulate. For example,KEYCODE_HOME
for the home button orKEYCODE_VOLUME_UP
for the volume up button.
Example Output:
Upon execution, the device will register the appropriate keypress, similar to physically pressing the key. There won’t be any direct output in the terminal, but the device’s response will be visible (such as returning to the home screen or increasing the volume).
Send a text to an Android device
Code:
adb shell input text "Hello%20World"
Motivation:
Sending text directly to a device can be exceptionally useful for testing apps that require text input. Rather than manually typing out strings for input fields or search queries, this command automates the process, saving significant time and reducing human error.
Explanation:
adb shell
: Initiates the shell environment on the Android device.input
: Specifies that you’re sending some form of input.text
: Indicates that the input type is text."Hello%20World"
: The text string you wish to input. Note that spaces within the input must be represented by%20
.
Example Output:
Once executed, the text “Hello World” will appear in the active text field on the Android device, as if typed by the user. There will be no terminal output, but the result will be visible on the device screen.
Send a single tap to an Android device
Code:
adb shell input tap x_position y_position
Motivation:
Simulating taps on an Android device can be crucial for testing app functionality where user taps trigger actions. This can be especially helpful for automated testing scripts that need to interact with the user interface to validate behaviors or reproduce issues.
Explanation:
adb shell
: Opens a shell session with the Android device.input
: Used to initialize an input event.tap
: Specifies that the input event is a tap/single touch.x_position y_position
: These are the screen coordinates where the tap will occur. You need to replace these placeholders with actual numbers to simulate a tap at the desired location on the screen.
Example Output:
After executing this command, the device will behave as though the screen was tapped at the provided coordinates. You may notice an app launch, button press, or other responses typically triggered by a user tap.
Send a swipe gesture to an Android device
Code:
adb shell input swipe x_start y_start x_end y_end duration_in_ms
Motivation:
Swipe gestures are a common interaction on touch-screen devices, and being able to automate them is invaluable for testing user interface behaviors such as scrolling through lists, navigating between app pages, or verifying gesture-based features like dragging.
Explanation:
adb shell
: Accesses the device’s command-line interface.input
: Initiates the input sequence.swipe
: Determines that the input will be a swipe gesture.x_start y_start
: These define the starting coordinates of the swipe.x_end y_end
: These are the ending coordinates for the swipe gesture.duration_in_ms
: Specifies how long the swipe should take in milliseconds, making it possible to simulate both fast and slow swipes.
Example Output:
Executing this command will result in the simulated swipe on the device. This might scroll a list, slide between screens, or interact with any swipe-sensitive component. The impact will be observed on the device’s UI but not in the terminal.
Send a long press to an Android device using a swipe gesture
Code:
adb shell input swipe x_position y_position x_position y_position duration_in_ms
Motivation:
Long presses are often used to reveal additional options or trigger context menus in Android applications. Being able to simulate long presses allows for thorough testing of all app functionalities, ensuring that context menus and other long-press triggered features are working properly.
Explanation:
adb shell
: Initiates a connection to the device’s shell.input
: Begins the input process on the device.swipe
: Even though a tap or press is desired, swipe is used with identical start and end coordinates to simulate a press.x_position y_position
: The coordinates where the long press should occur.duration_in_ms
: The duration of the press in milliseconds. A longer duration in this command simulates a long press; for example, 1000ms.
Example Output:
Upon execution, this command will make the device perform a long press at the specified coordinates. This can lead to a different UI behavior, such as showing a context menu, although there won’t be direct feedback in the terminal.
Conclusion
The input
command in Android Debug Bridge (ADB) provides an efficient and robust means to simulate a variety of user actions on an Android device. By understanding and utilizing each function—whether it’s tapping, typing text, swiping, or long-pressing—developers and testers can automate comprehensive tests and demonstrate app behavior. These interactions mimic real user input and provide an excellent way to identify potential issues or verify features without the need for manual intervention.