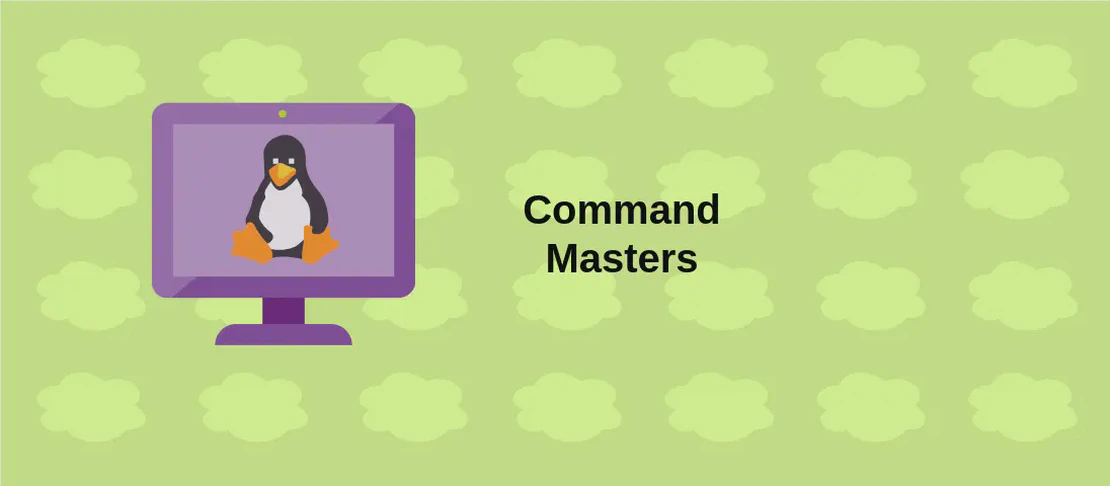
How to Use the Command 'Install-Module' (with Examples)
- Windows
- December 17, 2024
The Install-Module
command in PowerShell is a versatile and powerful command used extensively for managing PowerShell modules. PowerShell modules are packages that contain PowerShell scripts, cmdlets, functions, and workflows to be used in automating various system tasks. The Install-Module
command allows users to not only install modules from the PowerShell Gallery, NuGet, and other repositories, but also update them, specify version constraints, choose their installation scope, and many other advanced functionalities.
Install a Module or Update to the Latest Available Version
Code:
Install-Module module
Motivation for Using the Example:
This use case is crucial for users who want to ensure they have the latest features, security updates, and bug fixes provided by the module authors. By simply running this command without additional parameters, users can ensure their modules are up-to-date, which is important for maintaining system performance and security.
Explanation:
Install-Module
: This is the base command used to install or update a module. It retrieves the module from the default repository and installs it.module
: This placeholder should be replaced with the actual name of the module you wish to install or update.
Example Output:
Installing module...
Module 'module' installed successfully.
Install a Module with a Specific Version
Code:
Install-Module module -RequiredVersion version
Motivation for Using the Example:
Sometimes, a specific version of a module is required to maintain compatibility with other scripts or systems. By specifying a particular version, users can maintain a stable environment and avoid breaking changes that might occur in later versions.
Explanation:
module
: Name of the module to be installed.-RequiredVersion
: This parameter allows specifying the exact version of the module you wish to install, ensuring that no other version will be installed.version
: Represents the version number of the module required.
Example Output:
Installing module version...
Module 'module vX.X.X' installed successfully.
Install a Module No Earlier Than a Specific Version
Code:
Install-Module module -MinimumVersion version
Motivation for Using the Example:
In some scenarios, users need at least a particular version to access new features or to meet specific dependencies. Using -MinimumVersion
ensures that any version older than the specified version will not be installed.
Explanation:
module
: The name of the module desired for installation.-MinimumVersion
: Sets a baseline version, meaning any version equal to or newer than this can be installed.version
: The minimum acceptable version number.
Example Output:
Checking available versions...
Module 'module' version 'vX.X.X and above' installed successfully.
Specify a Range of Supported Versions (Inclusive) of the Required Module
Code:
Install-Module module -MinimumVersion minimum_version -MaximumVersion maximum_version
Motivation for Using the Example:
Users sometimes need to ensure a module is within a specific range to maintain compatibility with other system components or requirements. This range flexibility allows users to tailor the module’s version for tailored functionality while preventing unwanted changes introduced in newer versions.
Explanation:
module
: Module name to be installed.-MinimumVersion
: Specifies the minimum version acceptable.minimum_version
: The lower bound version number.-MaximumVersion
: This defines the upper limit version allowable.maximum_version
: The upper bound version number.
Example Output:
Evaluating module versions for compatibility...
Module 'module' version within specified range installed successfully.
Install Module from a Specific Repository
Code:
Install-Module module -Repository repository
Motivation for Using the Example:
Organizations often use specific repositories other than the default PowerShell Gallery for internally tested or custom modules. This use case allows users to target these repositories directly, ensuring that they pull a module from the correct or trusted source.
Explanation:
module
: The module you want to install.-Repository
: This parameter specifies the name of the repository from which the module should be installed.repository
: The name of the desired repository.
Example Output:
Locating 'module' in 'repository'...
Module 'module' installed successfully from 'repository'.
Install Module from Specific Repositories
Code:
Install-Module module -Repository repository1 , repository2 , ...
Motivation for Using the Example:
When modules may reside in multiple repositories or when multiple repositories are authorized to provide the module, this use case lets users specify one or more repositories, broadening the potential source pool while maintaining control.
Explanation:
module
: Target module name required for installation.-Repository
: Instructs PowerShell to consider the named repositories.repository1, repository2, ...
: A list of repositories to be searched for the desired module.
Example Output:
Searching for 'module' in multiple repositories...
Module 'module' installed successfully from one of the specified repositories.
Install the Module for All/Current User
Code:
Install-Module module -Scope AllUsers|CurrentUser
Motivation for Using the Example:
Deciding whether to install a module for a single user or for all users on a machine is crucial in multi-user environments. This option provides flexibility in managing user-specific versus system-wide configurations.
Explanation:
module
: The module intended for installation.-Scope
: Determines the scope of the installation, allowing personal (CurrentUser) or system-wide (AllUsers) installations.AllUsers|CurrentUser
: Targets the scope choice: “AllUsers” for system-wide and “CurrentUser” for the individual user.
Example Output:
Installing 'module' for all users...
Module 'module' successfully installed with AllUsers scope.
Perform a Dry Run to Determine Which Modules Will be Installed, Upgraded, or Removed
Code:
Install-Module module -WhatIf
Motivation for Using the Example:
Before making changes to a system, understanding the consequences can be critical. The WhatIf
parameter provides a safe way to preview what changes would occur without executing them, helping prevent disruptions due to unexpected installations or upgrades.
Explanation:
module
: Module name used in a simulated action.-WhatIf
: Triggers a simulation that displays what actions the command would perform without making any changes.
Example Output:
WhatIf: Performing the operation "Install-Module" on target "Version X of module".
WhatIf: Installing module...
Conclusion
The Install-Module
command is a fundamental tool within the PowerShell ecosystem, providing the ability to install, update, and manage modules efficiently. Each use case and its functionalities highlight the command’s flexibility, ensuring that users can maintain their environments in precise manners tailored to their specific requirements. Mastering these use cases will enhance your control over module management, ultimately leading to more secure, efficient, and reliable system operations.