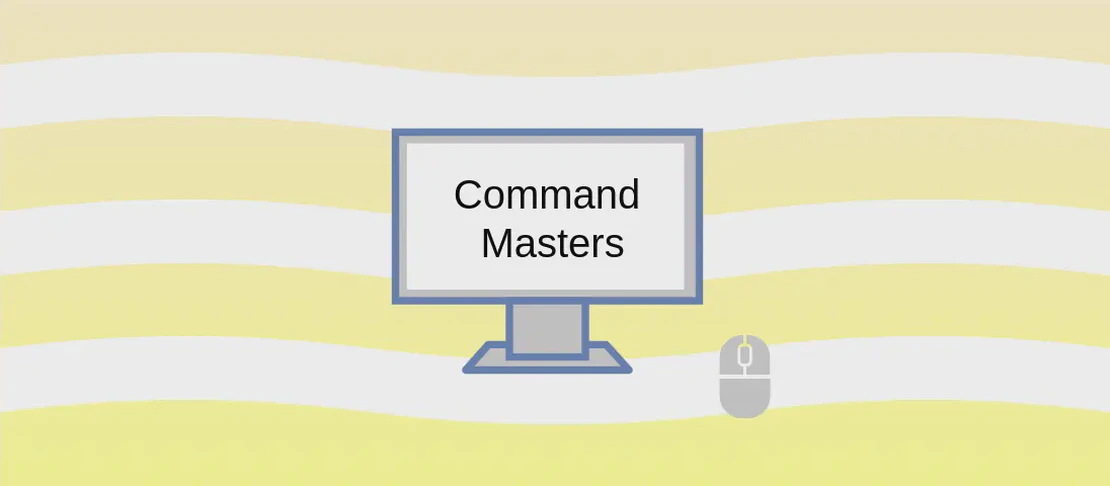
Using PowerShell's `Invoke-Item` Command (with examples)
- Windows
- December 17, 2024
Invoke-Item
is a powerful command available in PowerShell that allows users to open files using their respective default programs. This command is particularly useful for quickly launching a variety of files without needing to manually navigate through explorers or find the appropriate application for each file. Whether you’re managing a directory full of documents, images, or any other file types, Invoke-Item
provides a streamlined and efficient workflow for opening them directly from the command line.
Use case 1: Open a file in its default program
Code:
Invoke-Item -Path path\to\file
Motivation:
You have a document or file you need to inspect or edit, and you wish to open it quickly without hassle. Instead of manually finding the file through File Explorer and double-clicking it, you can use Invoke-Item
from within PowerShell, speeding up your process and allowing you to continue working seamlessly in command-line environments.
Explanation:
-Path
: The-Path
argument specifies the path to the file you want to open. Replacepath\to\file
with the actual file path.- The simplicity of this command enables quick access to files directly from the console.
Example Output:
Upon execution, the specified file will open in its default program, such as opening a .docx
file in Microsoft Word or a .pdf
file in Adobe Reader.
Use case 2: Open all files inside a directory
Code:
Invoke-Item -Path path\to\directory\*
Motivation:
You’re working with all files in a specific directory, such as preparing for a presentation where you need multiple documents, images, and datasets open simultaneously. Using Invoke-Item
allows you to open all these files at once, saving the time and tedium of opening each individually.
Explanation:
-Path
: Specifies the path to the directory containing files. The asterisk*
at the end of the path indicates that all items in the directory should be opened.- This method is perfect for bulk operations where multitasking and workflow efficiency are critical.
Example Output: All files contained within the specified directory will open in their respective default applications simultaneously.
Use case 3: Open all PNGs inside a directory
Code:
Invoke-Item -Path path\to\directory\*.png
Motivation:
Designers or photographers often need to review, edit, or showcase images. Using this command, you can open all .png
files within a directory at once, which is incredibly useful during a creative review process or while comparing variants of image files.
Explanation:
-Path
: The path is set to the directory containing the PNG files, with an asterisk and extension.png
*.png
to specify only the opening of PNG files.
Example Output: All PNG files in the specified directory will open, possibly in your image viewer application, allowing for rapid review and editing.
Use case 4: Open all files inside a directory containing a specific keyword
Code:
Invoke-Item -Path path\to\directory\* -Include *keyword*
Motivation: When dealing with large directories, it’s common to need only specific files that contain a certain keyword. For example, when compiling project materials that include a standard naming convention or tag. This command helps open only relevant files quickly without manual searching.
Explanation:
-Path
: Specifies the target directory.-Include
: Filters files by including only those containingkeyword
. Replacekeyword
with your specific term.- This specialized filter capability simplifies opening content based on specific naming patterns.
Example Output: Only files containing the specified keyword in their names will open, effectively reducing clutter and helping organize your workspace according to your needs.
Use case 5: Open all files inside a directory except those containing a specific keyword
Code:
Invoke-Item -Path path\to\directory\* -Exclude *keyword*
Motivation: Consider a scenario where most files are relevant, but a few with specific keywords are not needed. This method lets you open everything except the irrelevant, automatically eliminating files that don’t require your attention.
Explanation:
-Path
: Directs to the location of the files.-Exclude
: Works oppositely compared to-Include
, opening all files while excluding those with thekeyword
.`
Example Output: Files that do not contain the specified keyword are opened, helping to keep unnecessary files out of your workspace.
Use case 6: Perform a dry run to determine which files will be opened inside a directory through Invoke-Item
Code:
Invoke-Item -Path path\to\directory\* -WhatIf
Motivation:
Perhaps you’re uncertain about which files will be targeted by your Invoke-Item
command using either -Include
or -Exclude
. This dry run feature helps you verify file selections first, making sure you only open what is intended before executing potentially time-consuming actions.
Explanation:
-Path
: Sets the directory path to check.-WhatIf
: Performs a simulation of the command, displaying which items would open without actually opening them.- This safety net prevents mistakes in bulk operations by allowing pre-execution verification.
Example Output:
The PowerShell console displays a list of files that would be opened by the command if executed without -WhatIf
, giving you a preview of the outcome.
Conclusion:
The Invoke-Item
command is a versatile tool in PowerShell, suitable for a range of file management tasks, particularly when dealing with numerous files or needing a precise selection based on file names or types. This command enhances productivity by streamlining the process of opening files, tailored perfectly for users who require efficiency alongside command-line operations. Through practical examples, this article has demonstrated the applicability and flexibility that Invoke-Item
provides in a variety of scenarios.