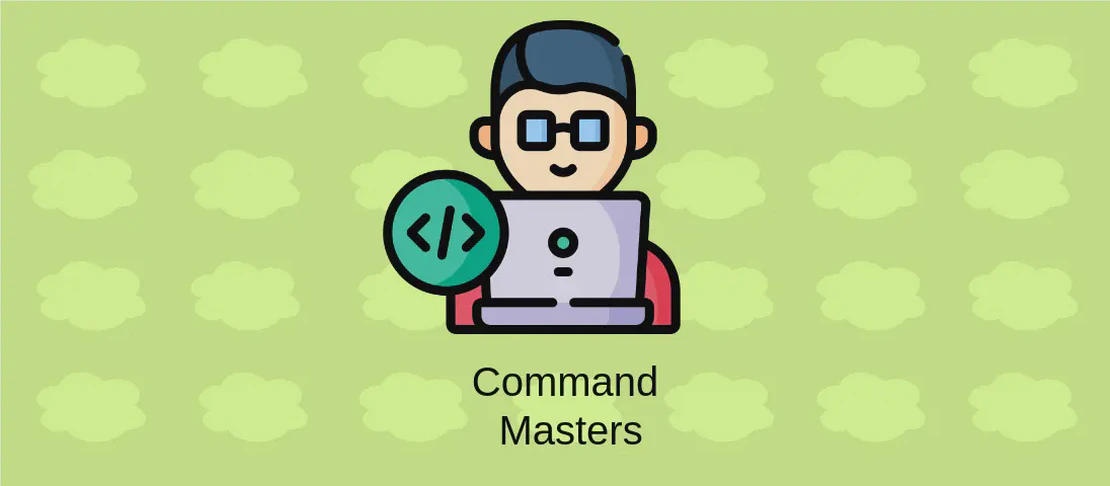
How to use the command 'Invoke-WebRequest' (with examples)
- Windows
- December 17, 2024
Invoke-WebRequest is a powerful command in PowerShell designed to perform HTTP or HTTPS requests to web resources. This utility is particularly useful for system administrators and developers who need to interact with web services, download data, or automate web-based tasks directly from the command line interface. By leveraging this command, users can send GET and POST requests, manage authentication, and handle advanced HTTP functionalities like custom headers and content types.
Use case 1: Download the contents of a URL to a file
Code:
Invoke-WebRequest http://example.com -OutFile path\to\file
Motivation:
Downloading the contents of a URL directly to a file is a common requirement in automating tasks such as updating software, backing up data, or retrieving web pages for further processing. This command simplifies the methodology by providing a straightforward way to save online content locally without needing a browser or a dedicated download tool.
Explanation:
Invoke-WebRequest
: This is the command used to perform the web request.http://example.com
: This is the URL from which the content is to be downloaded. It could be any web resource accessible over the internet.-OutFile path\to\file
: This specifies the local path where the downloaded content should be saved. Replacepath\to\file
with the actual destination path and filename.
Example output:
Upon successful execution, the content from http://example.com
will be saved to the specified file path, with no visible output in the console unless an error occurs.
Use case 2: Send form-encoded data (POST request of type application/x-www-form-urlencoded
)
Code:
Invoke-WebRequest -Method Post -Body @{ name='bob' } http://example.com/form
Motivation:
Sending data through a POST request is a common operation when interacting with web forms or APIs that require data submission. This use case is particularly useful for automating tasks where information needs to be sent to a server, such as filling out web forms automatically or interacting with older APIs that don’t use JSON.
Explanation:
Invoke-WebRequest
: The command to perform the HTTP request.-Method Post
: This option specifies that the request should be a POST, typically used to send data to a web server.-Body @{ name='bob' }
: This argument defines the data sent in the request body. In this case, we are sending a parametername
with the valuebob
, formatted as form-encoded data.http://example.com/form
: The URL to which the POST request is sent, typically the action URL of a web form.
Example output:
When executed, this command will submit the form data to http://example.com/form
. The output will not display in the console by default, but you can inspect the response to verify successful submission or errors.
Use case 3: Send a request with an extra header, using a custom HTTP method
Code:
Invoke-WebRequest -Headers @{ X-My-Header = '123' } -Method PUT http://example.com
Motivation:
Including custom headers in a web request is critical when interacting with APIs that require authentication tokens or delivering metadata. Employing different HTTP methods like PUT or DELETE is necessary for operations involving data updates or deletion on a server.
Explanation:
Invoke-WebRequest
: The command initiating the web request.-Headers @{ X-My-Header = '123' }
: This parameter allows the inclusion of custom headers, providing additional context or instructions to the web server.-Method PUT
: Specifies the HTTP method to be used. Here, PUT is typically used for updating resources on a server.http://example.com
: The resource URL where the request is directed.
Example output:
After execution, the server should process the request with the provided header and method. There will be minimal console output unless an error occurs, but the server’s response can be examined for confirmation or further information.
Use case 4: Send data in JSON format, specifying the appropriate content-type header
Code:
Invoke-WebRequest -Body '{"name":"bob"}' -ContentType 'application/json' http://example.com/users/1234
Motivation:
Sending JSON data in HTTP requests is standard in modern web development, especially for API communications. JSON is a lightweight data-interchange format that is easy to parse and generate, making it ideal for client-server communications.
Explanation:
Invoke-WebRequest
: This command performs the web request.-Body '{"name":"bob"}'
: Specifies the data payload in JSON format, withname
as the key andbob
as its value.-ContentType 'application/json'
: Ensures the server knows to interpret the request body as JSON.http://example.com/users/1234
: The target URL, which frequently involves an API endpoint that operates on user data.
Example output:
On successful execution, a JSON-formatted request body will be sent to http://example.com/users/1234
, with results visible in the server’s response output or console feedback upon interception of errors.
Use case 5: Pass a username and password for server authentication
Code:
Invoke-WebRequest -Headers @{ Authorization = "Basic "+ [System.Convert]::ToBase64String([System.Text.Encoding]::ASCII.GetBytes("myusername:mypassword")) } http://example.com
Motivation:
Many web services, especially RESTful APIs, enforce security through basic HTTP authentication, requiring valid credentials. This use case demonstrates how to supply such credentials automatically without manual input during the request process.
Explanation:
Invoke-WebRequest
: Initiates the web request.-Headers @{ Authorization = "Basic "...}
: Provides the basic authentication header, necessary for access to secured resources.[System.Convert]::ToBase64String(...)
: Converts the username and password into a Base64 string, as HTTP Basic Authentication requires encoding the credentials in this manner.http://example.com
: The URL requiring authentication for access.
Example output:
Execution sends a request with encoded credentials to the specified URL. Successful authentication will result in a response appropriate to the resource accessed, while failure may return an “Unauthorized” error.
Conclusion:
Invoke-WebRequest supports a multitude of web interaction capabilities, making it indispensable for administrators and developers working in PowerShell. Each use case displays different functionalities such as downloading content, submitting form data, handling JSON payloads, customizing headers, and managing authentication, enabling users to perform complex web operations directly via command line.