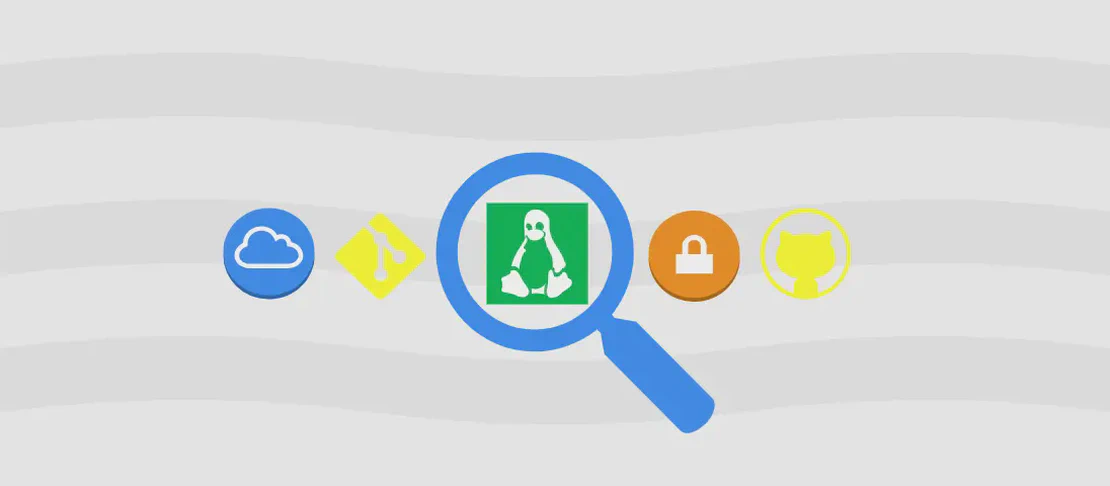
IPython (with examples)
IPython is an upgraded version of the standard Python interactive shell. It provides additional features such as automatic history, dynamic object introspection, easier configuration, command completion, access to the system shell, and more. In this article, we will explore various use cases of the ipython
command.
1: Start a REPL (interactive shell)
The ipython
command starts an interactive IPython session, also known as Read-Eval-Print Loop (REPL). This allows you to execute Python code interactively and see the results immediately.
$ ipython
Motivation: Use the IPython shell to experiment with code snippets, test small pieces of code, or explore the behavior of Python statements and expressions.
Example output:
Python 3.9.5 (default, May 4 2021, 03:36:27)
Type 'copyright', 'credits' or 'license' for more information
IPython 7.24.1 -- An enhanced Interactive Python. Type '?' for help.
In [1]: 2 + 2
Out[1]: 4
In [2]: print("Hello, World!")
Hello, World!
2: Enter an interactive IPython session after running a Python script
Sometimes, you may want to run a Python script and then enter an IPython session with the current execution context. The -i
option allows you to achieve this.
$ ipython -i script.py
Motivation: This is useful when you want to inspect the state of variables or interactively explore the execution environment after the script has completed.
Example output:
Hello from script.py!
>>> name
'John Doe'
>>> age
30
3: Create default IPython profile
IPython allows you to create profiles that define various configuration options. The ipython profile create
command creates a default profile with default configuration files.
$ ipython profile create
Motivation: Defining custom profiles can help you tailor the IPython environment to your needs, such as setting up startup scripts, configuring extensions, or changing default behaviors.
Example output:
[ProfileCreate] Generating default config file: '/home/user/.ipython/profile_default/ipython_config.py'
[ProfileCreate] Generating default config file: '/home/user/.ipython/profile_default/ipython_kernel_config.py'
4: Print the path to the directory for the default IPython profile
To locate the directory where the IPython profile is stored, you can use the ipython locate profile
command.
$ ipython locate profile
Motivation: This command allows you to quickly find the path to the default profile directory, which can be helpful when you want to modify the configuration files or access other profile-specific resources.
Example output:
/home/user/.ipython/profile_default
5: Clear the IPython history database, deleting all entries
IPython keeps track of the command history, which can be useful for reviewing and reusing previous commands. However, there might be situations where you want to clear the history. The ipython history clear
command clears the history database, deleting all entries.
$ ipython history clear
Motivation: Clearing the command history can be useful when you want to remove sensitive information from the command history or start fresh without any previous history.
Example output:
[HistoryClear] Deleting all entries from history database ... done
By exploring these different use cases of the ipython
command, you can leverage the features and functionalities provided by IPython to enhance your Python interactive shell experience.