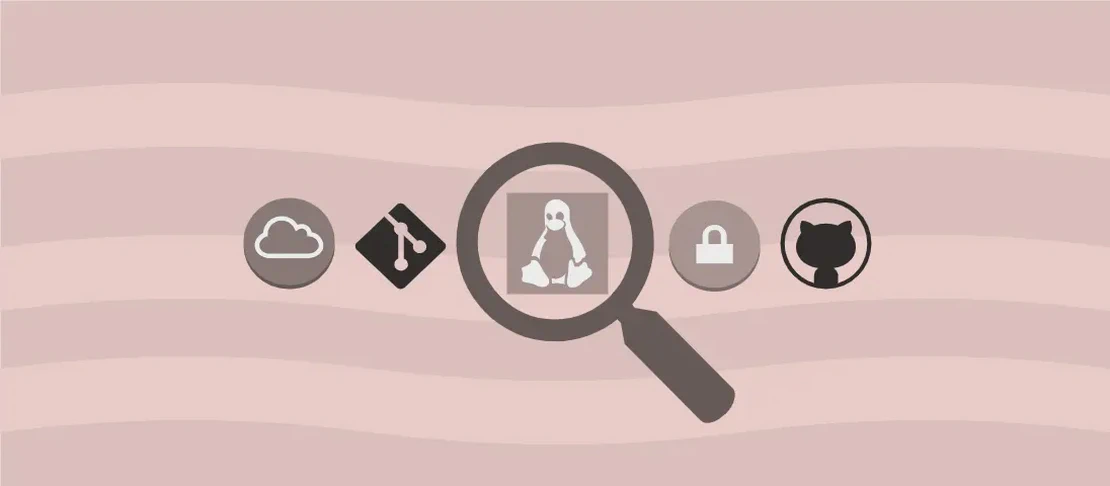
How to Use the Command 'irb' (with examples)
The irb
command launches an Interactive Ruby Shell, which is an environment where users can write and evaluate Ruby code in real-time. This tool is incredibly valuable for both beginners learning Ruby and experienced developers testing snippets of code on the fly. It reads Ruby code from stdin
and provides immediate feedback by evaluating the code and displaying results or errors. This immediacy makes irb
an excellent tool for experimentation and debugging.
Use case 1: Start the Interactive Shell
Code:
irb
Motivation:
Starting the Interactive Ruby Shell using the irb
command is a fundamental use case for anyone interested in running Ruby code interactively. This allows developers to input code line-by-line and execute it on the spot, getting immediate results. It’s particularly useful for those who are new to Ruby, as it provides a sandboxed environment to explore the language’s syntax and features without the need to set up a full development environment or write complete Ruby scripts.
Explanation:
irb
: The sole argument here stands for Interactive Ruby Shell. When you enter this command, it initializes an interactive session where you can type in your Ruby code. Since no additional arguments are provided, it starts with default settings, which means it loads with the current Ruby interpreter available on your system.
Example Output:
irb(main):001:0>
This output indicates that you are now in the main session of the IRB. The prompt irb(main):001:0>
shows the session and line number, helping you keep track of your commands and their sequence.
Conclusion:
The irb
command is a powerful tool in the Ruby programming arsenal, offering a responsive and interactive way to explore and manipulate Ruby code. The simplicity of starting it through a single command (as shown in our example) underscores its accessibility, ensuring that even novice programmers can quickly immerse themselves in learning Ruby. This tool’s flexibility and immediacy make it appealing for experimenting with new code, troubleshooting, or just playing around with Ruby syntax and functionality. Whether for education or professional development, irb
is an essential component of a Ruby programmer’s toolkit.