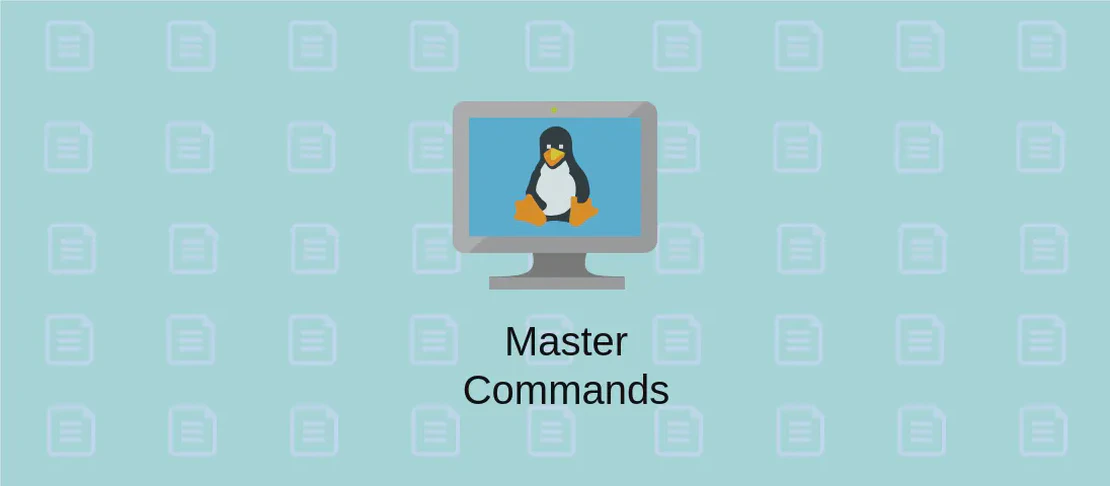
How to use the command 'jar' (with examples)
The jar
command in Java is a versatile tool used for packaging compiled Java classes and resources into a single archive, typically with a .jar (Java ARchive) extension. This format simplifies the distribution and deployment of Java applications and libraries. With jar
, you can compress, view, and manage these archive files efficiently. Below are some practical use cases illustrating how to utilize the jar
command effectively with examples.
Use case 1: Recursively archive all files in the current directory into a .jar file
Code:
jar cf file.jar *
Motivation:
This command is particularly useful when you want to bundle all the compiled classes and resources in a project for distribution or deployment. Packaging everything into a JAR file allows you to easily share and deploy your application without worrying about managing multiple files. It is essential when preparing your project for release or when various parts of the application need to be executed together.
Explanation:
c
: This stands for “create” and indicates that you want to create a new archive.f
: This signifies that the output should be written to a file, which in this case isfile.jar
.file.jar
: The name of the JAR file that will be created, which will contain all files from the directory.*
: A wildcard character used to specify all files in the current directory, ensuring that every file is recursively added to the JAR.
Example Output:
Executing this command will create a file.jar
in the current directory, which will contain all the files and subdirectories that were present in that directory. It doesn’t produce any terminal output, but you will find the file.jar
in your working directory which you can further inspect or use for deployment.
Use case 2: Unzip .jar/.war file to the current directory
Code:
jar -xvf file.jar
Motivation:
There are instances when you need to inspect the contents of a JAR or WAR file. Perhaps you need to check the files for debugging purposes or alter some configuration files. The jar
command allows you to extract these contents into your current directory, making it easy to view and edit the contained files.
Explanation:
x
: This stands for “extract,” meaning you wish to unzip or unpack the JAR file.v
: This represents “verbose,” indicating that the command should print out each file as it is being extracted. This is particularly useful for understanding the contents as they are decompressed.f
: Again, this indicates that the operation is to be conducted on a file,file.jar
in this context.file.jar
: The name of the JAR file you are extracting.
Example Output:
Running this command will output the names of the files as they are extracted into the current directory. You will see a list of files echoing in your terminal like META-INF/MANIFEST.MF
, com/example/App.class
, etc., depending on the contents of file.jar
.
Use case 3: List a .jar/.war file content
Code:
jar tf path/to/file.jar
Motivation:
Listing the contents of a JAR or WAR file without extracting them is often necessary when you want to quickly assess what is inside. It serves as a useful tool for verifying that certain classes or resources are present without the need to unpack the archive, thus saving time and avoiding file clutter.
Explanation:
t
: This stands for “table of contents,” meaning you want to list the files in the archive.f
: As before, this points out the operation to be performed on a file, in this case,file.jar
.path/to/file.jar
: The path leads to the JAR file whose contents you want to list.
Example Output:
Executing this command will result in a list of file names that are stored inside file.jar
. For instance, META-INF/MANIFEST.MF
, com/example/App.class
, and more depending on how the JAR was structured. This overview helps developers confirm the interior structure and file presence.
Use case 4: List a .jar/.war file content with verbose output
Code:
jar tvf path/to/file.jar
Motivation:
Sometimes it’s beneficial to get detailed information about each file in a JAR, such as its size and modification date. The verbose option allows you to see more detailed metadata for each of the files contained within the archive, which is useful for debugging or verifying the archive contents in detail.
Explanation:
t
: Requests a table of contents list for the archive.v
: Indicates a verbose output, meaning it will provide more comprehensive information (e.g., file size, last modification time) beyond just the file names.f
: Once again specifies that the command is acting on a file.path/to/file.jar
: The path to the JAR file in question.
Example Output:
This command outputs detailed information about each item in the JAR, such as:
927 Fri Oct 01 11:53:00 PDT 2023 META-INF/MANIFEST.MF
2255 Fri Oct 01 11:53:00 PDT 2023 com/example/App.class
Each line includes the size, date, and time of last modification, followed by the file name, providing thorough insight into the JAR’s contents.
Conclusion:
The jar
command is essential for managing Java archives, offering powerful options for creating, extracting, and inspecting JAR and WAR files efficiently. Whether you are bundling your Java application for deployment or inspecting a library’s contents, the examples above provide a solid foundation for leveraging this versatile tool in your workflow.