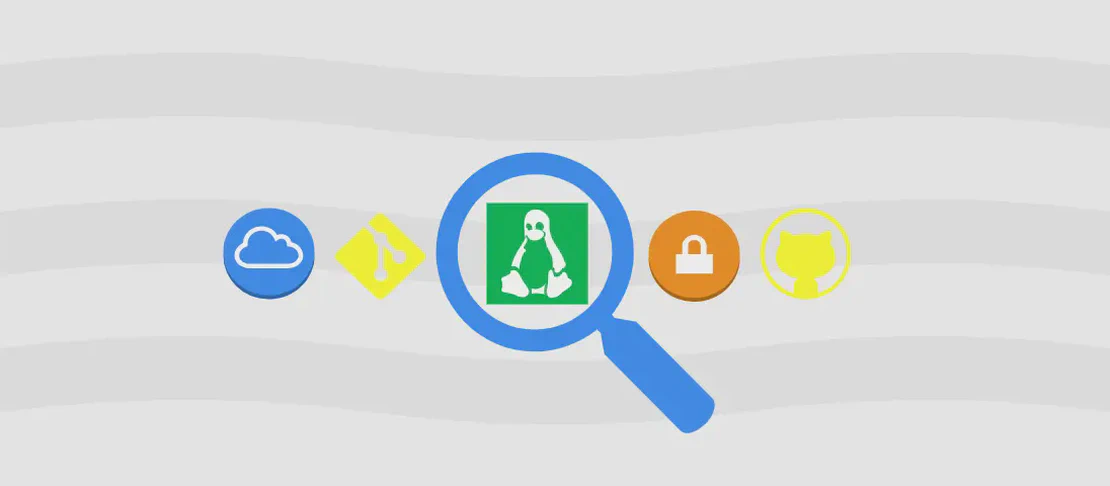
How to Sign and Verify JAR Files Using 'jarsigner' (with examples)
The jarsigner
tool is a command-line utility included in the Java Development Kit (JDK) that allows developers to digitally sign Java Archive (JAR) files. This utility ensures that the contents of the JAR file have not been tampered with and that they originate from a trusted source. By adding a signature to a JAR file, developers can assure users of the file’s authenticity and integrity. Additionally, jarsigner
can be used to verify the signatures of JAR files, assisting in confirming their reliability.
Use case 1: Sign a JAR file
Code:
jarsigner path/to/file.jar keystore_alias
Motivation:
Digitally signing a JAR file is a critical security practice for developers aiming to distribute Java applications. By signing the JAR file, developers provide users with an assurance that the code originates from a verified source and has not been altered since it was signed. This process helps establish trust between the developer and the end user, as users can verify the signature to confirm the file’s authenticity.
Explanation:
jarsigner
: This is the command used to invoke the JAR signing tool.path/to/file.jar
: This represents the path to the JAR file that needs to be signed. Before signing, ensure that you have the correct path to avoid any errors.keystore_alias
: The alias is a reference to a specific entry within a keystore. A keystore is a repository of security certificates, either certificates of Java applications or public keys to check security certificates. The alias is used to access a specific keypair/certificate for signing the JAR.
Example Output:
Upon running the command, if the signing operation is successful, the utility might not display specific output indicating completion, but you can expect the following type of message when specifying verbose mode:
jar signed.
Note: If you encounter “permission denied” or “keystore password” issues, ensure you have the necessary permissions and passwords.
Use case 2: Sign a JAR file with a specific algorithm
Code:
jarsigner -sigalg algorithm path/to/file.jar keystore_alias
Motivation:
In the ever-evolving landscape of cybersecurity, various algorithms offer different levels of security. A default signing algorithm might not always provide the desired level of security. By explicitly specifying an algorithm, developers can choose an appropriate cryptographic algorithm that matches their security needs or regulatory requirements, ensuring better protection and compliance.
Explanation:
-sigalg algorithm
: This option allows the user to specify a signing algorithm. By using-sigalg
, the developer can determine which cryptographic algorithm to use for the signing process. Common choices includeSHA256withRSA
,SHA1withDSA
, etc.path/to/file.jar
: Indicates the JAR file’s location that is being signed.keystore_alias
: As previously described, this refers to the specific signature used to sign the JAR within the keystore.
Example Output:
The output for signing with a specific algorithm will typically be similar to the general signing process, but you’ll be assured the selected algorithm was applied:
jar signed using algorithm: SHA256withRSA.
Use case 3: Verify the signature of a JAR file
Code:
jarsigner -verify path/to/file.jar
Motivation:
Verification of a JAR file signature is crucial for developers and users to ensure that the file hasn’t been tampered with and originates from a credible source. Verifying the signature allows one to confirm the JAR file’s integrity and authenticity. This process is typically done post-download to ensure that the file received matches the signed original version.
Explanation:
-verify
: This argument instructsjarsigner
to verify the JAR file’s signature rather than sign it.path/to/file.jar
: Specifies the JAR file whose signature needs to be verified, indicating its location on the filesystem.
Example Output:
When a JAR file’s signature is verified, the output indicates the results of the verification attempt:
jar verified.
If verification fails, the tool might output an error showing that the file’s signature doesn’t match, signaling a potential security concern.
Conclusion:
The jarsigner
command is a powerful and essential tool for Java developers, ensuring the authenticity and integrity of JAR files that are distributed to users. Signing with jarsigner
establishes trust, while verifying uses the tool to affirm received files are untampered. Developers should leverage the flexibility of this command, such as specifying signing algorithms, to meet varied security needs and regulatory requirements effectively.