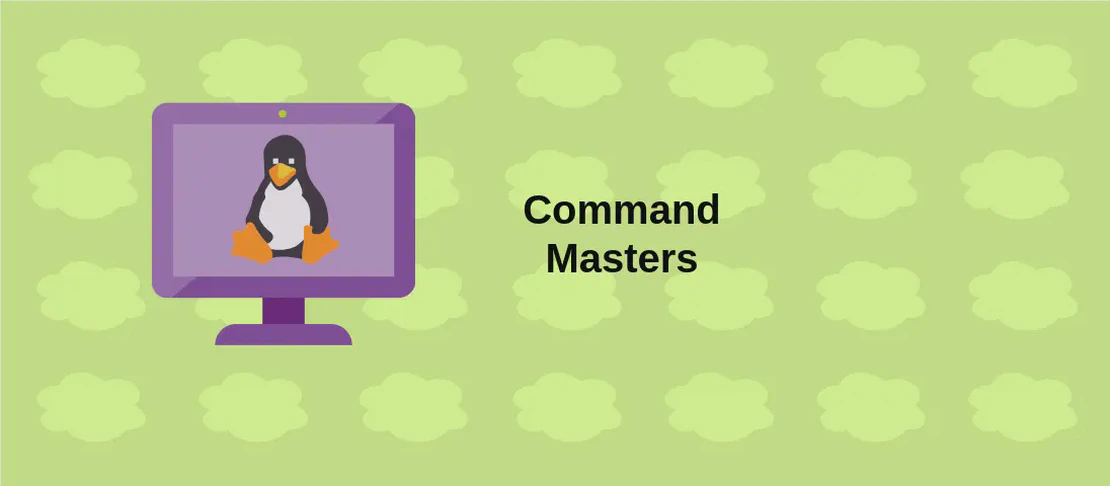
How to Use the Command "java" (with examples)
Java is a versatile and widely-used programming language that comes with a powerful application launcher command, java
. This command is responsible for initiating the Java Runtime Environment (JRE), which executes the bytecode produced by Java compilers. Whether you are running simple command-line applications or complex GUI-based applications, the java
command is indispensable. It allows developers to execute compiled Java classes, manage libraries, run pre-packaged JAR files, as well as provide debugging capabilities. Below, we explore several key use cases of the java
command.
Execute a Java .class
File with Main Method
Code:
java classname
Motivation:
This simple command is used to execute Java applications directly from a compiled class file. When you develop Java programs, they are initially organized into class files that contain bytecode, which can only be interpreted by the Java Virtual Machine (JVM). Running a .class
file using this command ensures that the application is executed in a platform-independent manner, making Java a highly portable programming language.
Explanation:
java
: Java application launcher.classname
: The name of the class containing themain
method that you want to execute. Themain
method serves as the entry point of the Java application.
Example output:
If “HelloWorld” is your class name and it includes a main
method that prints “Hello, World!”, the output of this command will be:
Hello, World!
Execute a Java Program With Additional Classpath
Code:
java -classpath path/to/classes1:path/to/classes2:. classname
Motivation:
Applications often depend on external classes and libraries that are not part of the core project. The -classpath
option is used to specify one or more locations (directories or JAR files) where the JVM can search for user-defined or third-party classes. This is particularly useful for projects that segregate classes into organized structures or depend on external libraries to function properly.
Explanation:
java
: Java application launcher.-classpath
: Specifies the classpath, which is a list of locations that the JVM searches for classes and other resources.path/to/classes1:path/to/classes2:.
: A colon-separated list of directories and JAR files. The dot (.
) denotes the current directory.classname
: The main class that you want to run, located within the provided classpath.
Example output:
You might run an enterprise application that relies on external libraries for database connectivity. The output varies based on what the main method in classname
executes.
Execute a .jar
Program
Code:
java -jar filename.jar
Motivation:
Java applications are often distributed as JAR files, which are Java ARchives, packaging multiple class files, resources, and metadata. Using the -jar
option enables you to execute the JAR file directly, making it convenient for deploying applications across different environments without requiring additional class management.
Explanation:
java
: Java application launcher.-jar
: Indicates that the JVM should execute the specified JAR archive.filename.jar
: The name of the JAR file you intend to execute.
Example output:
If filename.jar
is a packaged application that plays a sound file, running this command could immediately begin audio playback with no textual output.
Execute a .jar
Program with Debug on Port 5005
Code:
java -agentlib:jdwp=transport=dt_socket,server=y,suspend=y,address=*:5005 -jar filename.jar
Motivation:
Debugging complex applications is a common requirement during development. This command sets up a remote debugging environment by configuring Java Debug Wire Protocol (JDWP), making it possible to track down intricate issues using external tools like IntelliJ IDEA or Eclipse. Developers can set breakpoints, evaluate expressions, and interact with the application in a way normal execution does not allow.
Explanation:
java
: Java application launcher.-agentlib:jdwp=transport=dt_socket,server=y,suspend=y,address=*:5005
: Options that enable JDWP for debugging.transport=dt_socket
: Defines the transport mechanism as sockets.server=y
: Starts the JVM in server mode, waiting for a debugger to connect.suspend=y
: Suspends the execution of the application until the debugger is connected.address=*:5005
: Specifies the network address and port (5005) where the JVM listens for debugger connections.
-jar filename.jar
: Execute the specified JAR file.
Example output:
Once executed, the command line will indicate that the application is “Listening for transport dt_socket at address: 5005”. The program execution will suspend, awaiting a debugger connection.
Display JDK, JRE, and HotSpot Versions
Code:
java -version
Motivation:
Version information is essential for developers and system administrators who ensure compatibility across various environments, especially when different versions provide distinct features and bug fixes. This command offers clarity on the installed Java Development Kit (JDK), Java Runtime Environment (JRE), and the underlying HotSpot JVM.
Explanation:
java
: Java application launcher.-version
: Requests the currently installed version details of the Java platform components.
Example output:
Executing this command will provide output similar to:
java version "20.0.1" 2023-04-18
Java(TM) SE Runtime Environment (build 20.0.1+10)
Java HotSpot(TM) 64-Bit Server VM (build 20.0.1+10, mixed mode)
Display Help
Code:
java -help
Motivation:
The java
command has myriad options and parameters, each serving specific functions. Displaying the help information is essential for new developers and even experienced ones who need a brief rundown of available options. This command provides a comprehensive list of command-line options for the java
launcher.
Explanation:
java
: Java application launcher.-help
: Displays a help message that lists all available options for the command.
Example output:
The output consists of a detailed list of options, similar to:
Usage: java [options] <mainclass> [args...]
(To execute a class)
or java [options] -jar <jarfile> [args...]
(To execute a jar file)
...
Conclusion:
The java
command is the backbone of Java application execution, offering various functionalities to support routine development and deployment processes. By understanding its numerous options and applications, developers and administrators can optimize the execution, debugging, and management of Java programs effectively.