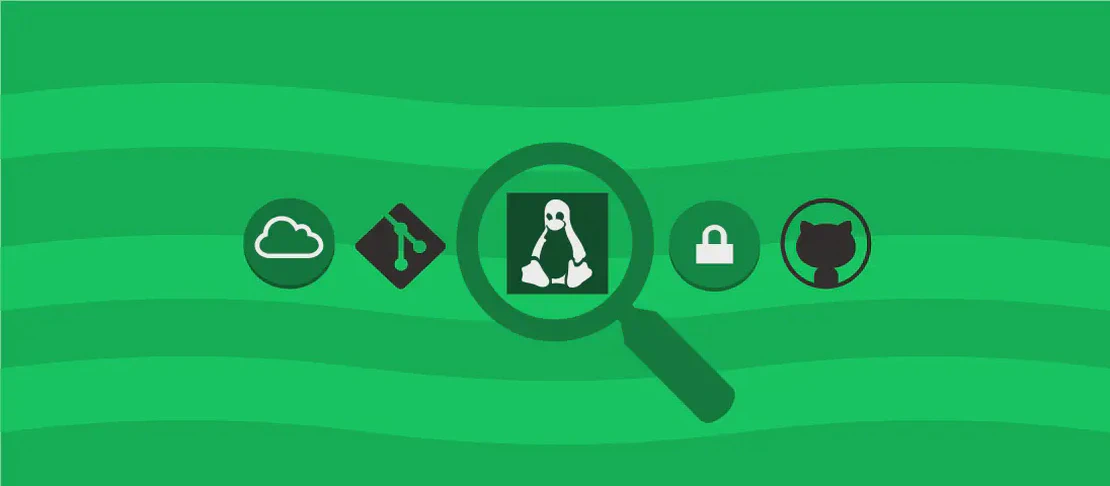
How to use the command 'javac' (with examples)
The javac
command is a pivotal tool in the Java development ecosystem, allowing developers to convert their human-readable .java
files into Java bytecode (contained within .class
files). Compiling code is an essential step in building Java applications, enabling execution within the Java Virtual Machine (JVM). This article outlines various javac
use cases, highlighting scenarios that optimize a developer’s workflow.
Use case 1: Compile a .java
file
Code:
javac path/to/file.java
Motivation:
Compiling individual .java
files is a common practice when developers work on specific parts of an application. For instance, when editing and testing a single Java class, recompiling just that file saves time, particularly in large projects where full recompilation could be resource-intensive.
Explanation:
javac
: This is the Java compiler command.path/to/file.java
: This argument specifies the path to the Java source file that you want to compile. By entering the correct path and filename,javac
locates and compiles the specified Java file.
Example Output:
Upon successful compilation, there may not be any console output, but a file.class
file containing the bytecode will be generated in the same directory as the source file. In case of compilation errors, such as syntax errors, detailed error messages will be displayed in the terminal.
Use case 2: Compile several .java
files
Code:
javac path/to/file1.java path/to/file2.java ...
Motivation:
Compiling multiple .java
files simultaneously is beneficial when working on interconnected classes or modules. This operation reduces the need to repeatedly invoke the javac
command for each file, streamlining the developer’s process by ensuring that related classes are compiled together, which can be advantageous during code integration and testing phases.
Explanation:
javac
: The Java compiler utility invoked to perform the compilation process.path/to/file1.java path/to/file2.java ...
: A list of source files to compile. Each path specifies the location of a Java file that needs to be compiled, allowing the developer to handle multiple files in a single run.
Example Output:
Successful execution results in the creation of a corresponding .class
file for each .java
file in the given paths. If errors occur in any file, the output will include detailed error messages for each problematic source file, facilitating quicker debugging.
Use case 3: Compile all .java
files in the current directory
Code:
javac *.java
Motivation:
Compiling all Java files in a directory is particularly useful during early development stages or when a project structure maintains all source files within a single folder. This command is a significant time-saver, ensuring that updates across various files are compiled together without manually specifying each file.
Explanation:
javac
: The main command for initiating the compilation process.*.java
: A wildcard pattern that matches all Java source files in the current directory. It simplifies batch compilation by automatically selecting every.java
file present.
Example Output:
Following execution, a series of .class
files, one for each .java
file, will be produced in the directory. Compilation errors in any of the files will generate error messages, pinpointing the exact file and line of the issue.
Use case 4: Compile a .java
file and place the resulting class file in a specific directory
Code:
javac -d path/to/directory path/to/file.java
Motivation:
Organizing compiled class files into specified directories aligns with best practices for maintaining a clean and scalable project structure. This is extremely useful in large projects involving multiple packages and modules. By directing output to a dedicated directory, developers can separate source files from compiled artifacts, aiding in easier project management and deployment.
Explanation:
javac
: Calls the Java compiler.-d path/to/directory
: The-d
flag specifies the destination directory for.class
files. Thepath/to/directory
indicates where the compiled bytecode should be stored, allowing the user to define a location outside of the source files themselves.path/to/file.java
: The source file path, indicating which file should be compiled.
Example Output:
As a result of this operation, a file.class
file will be generated within the specified directory. If the destination directory does not exist, javac
will create it. Any detected errors during compilation will be reported to the console.
Conclusion:
The javac
command provides a versatile range of options to cater to various compilation needs, whether handling individual files, multiple files, or integrating organization by directing output files to specific directories. Understanding and applying these use cases can significantly enhance productivity and code management in any Java development project.