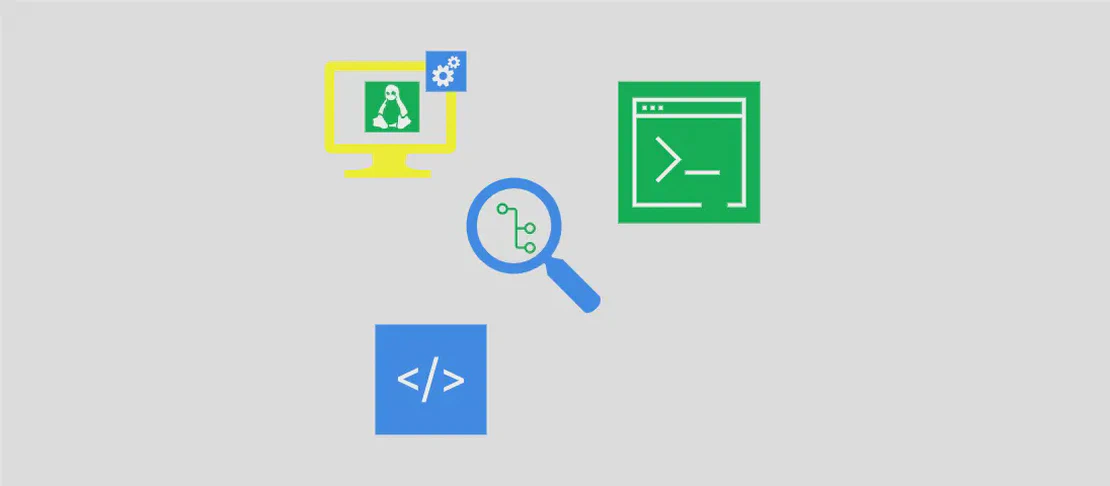
Disassembling Java Class Files with the 'javap' Command (with examples)
The javap
command, part of the Java Development Kit (JDK), is a useful tool for dissecting Java .class
files, which are compiled Java code. It allows developers to view the bytecode, the intermediate representation of Java code and inspect method signatures, fields, and other elements of the Java bytecode architecture. This can be particularly useful for learning and debugging Java programs, as well as understanding the structure of compiled classes.
Use case 1: Disassemble and List One or More .class
Files
Code:
javap path/to/file1.class path/to/file2.class
Motivation:
By disassembling .class
files, you gain insight into what the Java compiler is actually producing when it compiles your source code. This is particularly beneficial when you’re trying to understand optimization decisions, access levels, or just exploring the anatomy of a class, especially if source code is unavailable.
Explanation:
javap
: The command itself, invoking the program to disassemble the specified.class
files.path/to/file1.class path/to/file2.class
: These are the paths to the.class
files you want to examine. You can specify one or more files, separated by spaces.
Example Output:
Compiled from "Example1.java"
public class Example1 {
public Example1();
public void methodA();
public int methodB(int);
static {};
}
Compiled from "Example2.java"
public class Example2 {
public Example2();
public void displayInfo();
}
Use case 2: Disassemble and List a Built-In Class File
Code:
javap java.util.Date
Motivation:
Exploring built-in Java classes can be illuminating regarding how these classes are structured and can help in using them more effectively within your code. It can also be valuable for educational purposes, as it shows real-world examples of object-oriented principles applied by experienced developers.
Explanation:
javap
: Again, this is the command to disassemble Java files.java.util.Date
: This specifies the fully qualified name of the class you want to examine, which is a part of Java’s standard library.
Example Output:
Compiled from "Date.java"
public class java.util.Date implements java.io.Serializable, java.lang.Cloneable, java.lang.Comparable<java.util.Date> {
public java.util.Date();
public java.util.Date(long);
...
}
Use case 3: Display Help
Code:
javap -help
Motivation:
Accessing the help documentation from the command line is quick and efficient, particularly when you’re already working within terminal environments. Understanding the various options and flags available with javap
can enhance how you utilize its capabilities.
Explanation:
javap
: This is the base command being used.-help
: This option prints out the help information, listing all available options and their descriptions, providing guidance on how to usejavap
.
Example Output:
Usage: javap <options> <classes>
where options include:
-help --output information about options and exit
-version --print version information and exit
...
Use case 4: Display Version
Code:
javap -version
Motivation:
Knowing the version of javap
is crucial, especially when dealing with potential compatibility issues or when different features are available in different versions of the Java Development Kit (JDK). It also helps when reporting issues or seeking support.
Explanation:
javap
: This points to the tool being utilized.-version
: This flag outputs the version of thejavap
tool you have installed, which is tied to your JDK version.
Example Output:
javap 20.0.1
Conclusion:
In summary, the javap
command is a highly versatile tool for developers. It demystifies compiled Java files, allowing for a deeper understanding of Java bytecode, method declarations, and class structure, whether exploring custom classes, built-in library classes, or needing guidance through comprehensive help options. With the ability to check versions, it remains relevant for developers navigating various JDK versions. Whether it is for education, debugging, or sheer curiosity, javap
adds a layer of visibility into the workings of Java applications.