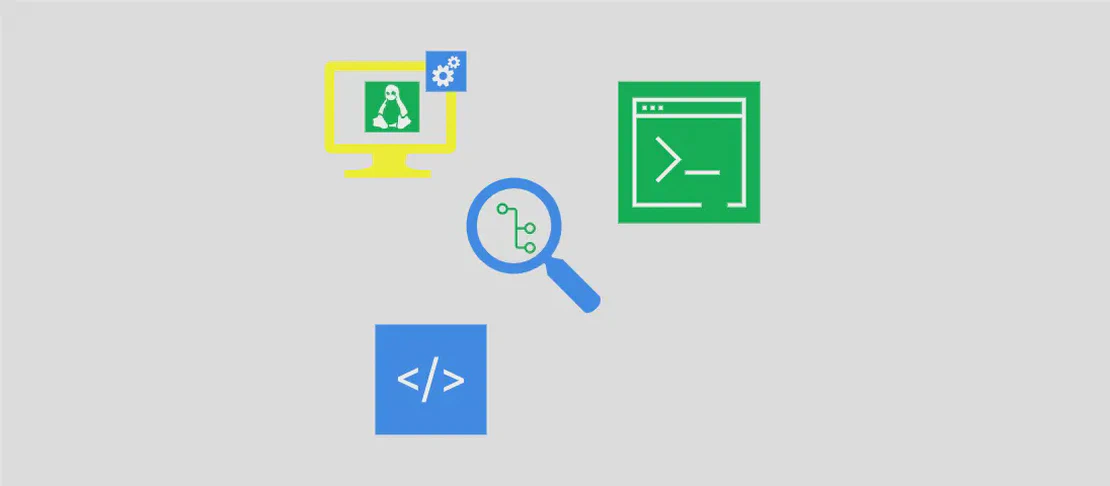
Mastering JBang: Effective Java Scripting (with examples)
JBang is a powerful tool that simplifies the creation and execution of Java programs directly from source files, much like scripting languages. This tool provides developers with an easy-to-use platform for developing self-contained Java applications without the need for a traditional project structure. JBang allows running Java snippets, managing dependencies, and even creating CLI applications effortlessly. Here, we illustrate various use cases of JBang, providing an in-depth look into its capabilities and practical examples to understand its applications better.
Use Case 1: Initialize a Simple Java Class
Code:
jbang init path/to/file.java
Motivation: Initializing a basic Java class is often the first step in starting a Java project. With JBang, you can quickly generate a skeleton Java file that saves time setting up a boilerplate structure. This is particularly useful for developers who want to dive straight into coding without manually setting up folders and files.
Explanation:
jbang
: The main command to invoke the JBang tool.init
: A sub-command that generates a new Java file with a template for a simple Java program.path/to/file.java
: The path where you want the new Java file to be created. Specify the filepath where the Java class should be initialized.
Example output: Running this command will create a bare-bones Java file at the specified location with a simple template for a Java class, including a main method.
Use Case 2: Initialize a Java Class for Scripting
Code:
jbang init --template=cli path/to/file.java
Motivation:
When writing command-line applications or scripts, you may need a Java class already tailored for scripting. JBang provides a cli
template that guides you in setting up a class specifically designed for CLI operations. This not only saves time but also gives you a structured starting point for your CLI application.
Explanation:
--template=cli
: Specifies that the generated template should be suitable for command-line interface (CLI) applications, providing additional infrastructure like handling command-line arguments.path/to/file.java
: The location and name of the Java file to be created.
Example output: This command will generate a Java file with pre-filled structures such as command-line argument parsing, ready to be expanded into a full-fledged CLI application.
Use Case 3: Explore Java and Dependencies in a REPL
Code:
jbang run --interactive
Motivation: Interactive programming environments like REPL (Read-Eval-Print Loop) are beneficial for exploring APIs, prototyping, and experimenting with Java code. It allows developers to test code snippets and dynamically load and unload dependencies. This can be a significant advantage in learning and debugging complex Java applications.
Explanation:
run
: Triggers the execution of a Java program.--interactive
: Opens an interactive Java shell session usingjshell
, allowing you to run Java code snippets and dynamically manage dependencies.
Example output:
The command opens jshell
in your terminal, welcoming you into an interactive session where you can immediately start typing and executing Java code snippets.
Use Case 4: Setup a Temporary Project for IDE Editing
Code:
jbang edit --open=codium|code|eclipse|idea|netbeans|gitpod path/to/script.java
Motivation: Sometimes quick edits to a script are necessary, and having the ability to open your Java script in an IDE can enhance productivity by providing features like syntax highlighting, autocompletion, and debugging. JBang simplifies setting up a temporary project in various IDEs for you.
Explanation:
edit
: Initiates the creation of a temporary project setup.--open=codium|code|eclipse|idea|netbeans|gitpod
: Opens the script in the specified IDE. You can specify your preferred IDE from the list.path/to/script.java
: The script you want to edit.
Example output: Once executed, this command opens your Java script in the selected IDE, allowing immediate access to all the powerful tools and features available within the development environment.
Use Case 5: Run a Java Code Snippet
Code:
echo 'Files.list(Paths.get("/etc")).forEach(System.out::println);' | jbang -
Motivation: At times, you might need to execute a quick Java operation without creating an entire Java file. This is where running Java code snippets directly from the command line can be advantageous. It’s an efficient way to execute small tasks or test Java functionalities on-the-fly.
Explanation:
echo
: A shell command that outputs the specified string.'Files.list(Paths.get("/etc")).forEach(System.out::println);'
: A Java snippet that lists and prints all files in the/etc
directory.| jbang -
: Pipes the output ofecho
into JBang, where-
specifies that the source is coming from standard input rather than a file.
Example output:
The command lists all the files in the /etc
directory directly on the terminal console.
Use Case 6: Run a Command-Line Application
Code:
jbang path/to/file.java command arg1 arg2 ...
Motivation: Once you have developed a Java application or script, running it with command-line arguments mimics real-world usage. This use case demonstrates how JBang facilitates the execution of Java programs as command-line applications, passing arguments dynamically.
Explanation:
path/to/file.java
: Specifies the Java file to be executed.command arg1 arg2 ...
: Command-line arguments that you pass to the Java program upon execution.
Example output: This command executes the Java program located at the specified path, processes the provided command-line arguments, and outputs the result accordingly.
Use Case 7: Install a Script on the User’s $PATH
Code:
jbang app install --name command_name path/to/script.java
Motivation: Installing scripts on the system PATH makes them accessible from anywhere in the terminal. This is particularly useful for frequently used scripts or utilities that you want to be available globally without specifying the full path every time.
Explanation:
app install
: A sub-command that manages script installation.--name command_name
: Specifies the name of the command by which the script will be accessible.path/to/script.java
: The path to the Java script you want to install.
Example output: After execution, the script becomes accessible globally under the specified command name. You can invoke it from any directory without referencing its full path.
Use Case 8: Install a Specific Version of JDK
Code:
jbang jdk install version
Motivation: In projects with specific Java version dependencies, having the ability to install and manage particular JDK versions ensures compatibility and adherence to requirements. JBang offers a straightforward way to install different JDK versions to be used in JBang-based developments.
Explanation:
jdk install
: Command for managing JDK installations.version
: The specific Java version you want to install (e.g., 11, 14, 17).
Example output: Executing this command installs the desired JDK version, which will thereafter be available for any JBang task or script that specifies it.
Conclusion:
JBang provides a versatile command-line toolset that simplifies common Java development tasks, making Java more accessible for scripting, rapid prototyping, and command-line application development. By illustrating various use cases, this guide demonstrates how JBang can be an indispensable tool for Java developers, streamlining the process of creating, running, and managing Java programs efficiently.