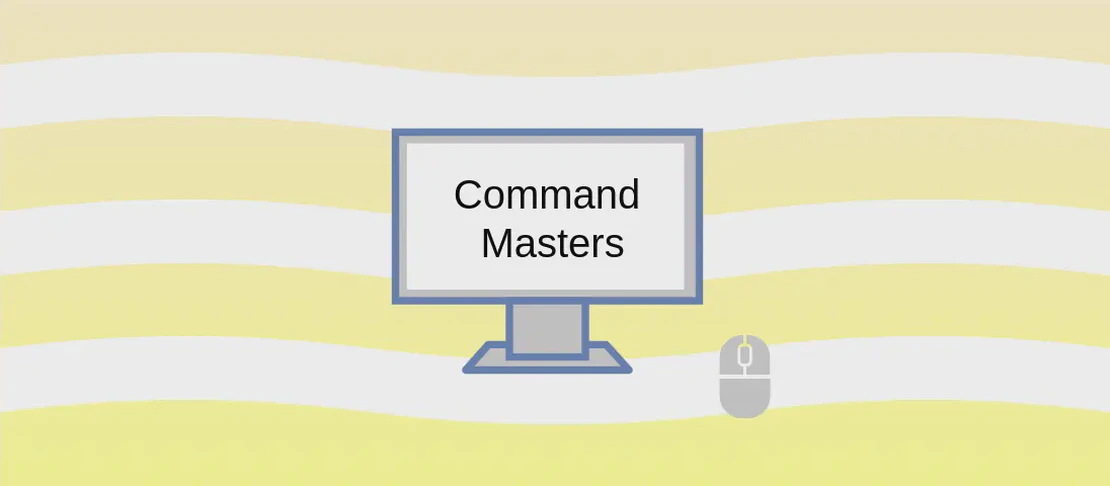
How to Use the Command 'jc' to Convert Command Output to JSON (with Examples)
The jc
command is a powerful tool that allows you to convert the output of traditional command-line utilities into JSON format. This enables better parsing and integration with modern programming environments that support JSON. By transforming command output into a structured format, jc
simplifies data manipulation and usage in scripts or applications where JSON is a preferred or required format.
Use case 1: Convert Command Output to JSON via Pipe
Code:
ifconfig | jc --ifconfig
Motivation:
Using command output directly as a JSON object streamlines the process of parsing and analyzing data from command-line utilities. For example, network administrators might use jc
with ifconfig
to capture and analyze detailed network interface data in JSON format, which simplifies integrating this information into dashboards or logs for automated monitoring and alerting systems.
Explanation:
ifconfig
: This command is used to configure network interfaces in Unix and Unix-like operating systems. It provides detailed information about each network interface.|
: The pipe operator sends the output of one command as input to another, allowing commands to be chained together.jc --ifconfig
: Thejc
command with the--ifconfig
argument tellsjc
to specifically interpret the output of theifconfig
command and convert it into JSON format.
Example Output:
[
{
"interface": "lo",
"flags": ["UP", "LOOPBACK", "RUNNING"],
"mtu": 65536,
"inet": "127.0.0.1",
"netmask": "255.0.0.0",
"prefixlen": 8,
"inet6": "::1",
"group": ["local"],
"txqueuelen": 1000
},
{
"interface": "eth0",
"flags": ["UP", "BROADCAST", "MULTICAST", "RUNNING"],
"mtu": 1500,
"inet": "192.168.1.5",
"netmask": "255.255.255.0",
"broadcast": "192.168.1.255",
"prefixlen": 24,
"inet6": "fe80::a00:27ff:fe4e:66a1",
"group": ["global"],
"txqueuelen": 1000
}
]
Use case 2: Convert Command Output to JSON via Magic Syntax
Code:
jc ifconfig
Motivation:
The magic syntax makes it even simpler to use jc
by allowing you to run and convert the command output to JSON in a single step without worrying about the intricate details of command piping. This use case is particularly useful in scripts where clarity and brevity are highly valued because it reduces the number of pipe operators necessary, contributing to a more readable script.
Explanation:
jc
: The base command that triggers the use of the JSON conversion tool.ifconfig
: Specifyingifconfig
directly afterjc
leverages the magic syntax, which indicates thatjc
should handle runningifconfig
and converting its output in one go.
Example Output:
[
{
"interface": "lo",
"flags": ["UP", "LOOPBACK", "RUNNING"],
"mtu": 65536,
"inet": "127.0.0.1",
"netmask": "255.0.0.0",
"prefixlen": 8,
"inet6": "::1",
"group": ["local"],
"txqueuelen": 1000
},
{
"interface": "eth0",
"flags": ["UP", "BROADCAST", "MULTICAST", "RUNNING"],
"mtu": 1500,
"inet": "192.168.1.5",
"netmask": "255.255.255.0",
"broadcast": "192.168.1.255",
"prefixlen": 24,
"inet6": "fe80::a00:27ff:fe4e:66a1",
"group": ["global"],
"txqueuelen": 1000
}
]
Use case 3: Output Pretty JSON via Pipe
Code:
ifconfig | jc --ifconfig -p
Motivation:
Pretty JSON formatting enhances data readability, particularly for debugging and manual analysis by developers and system administrators who need to review the structure and values of JSON data visually. By converting command outputs into well-indented, human-friendly JSON, you can make the data more accessible for further troubleshooting steps or validation purposes.
Explanation:
ifconfig
: Outputs the configuration and details of network interfaces.|
: Pipes theifconfig
output to the next command.jc --ifconfig
: Interprets and converts theifconfig
output to JSON.-p
: Stands for “pretty-print,” which makes the JSON output more human-readable by adding indentation and line breaks.
Example Output:
[
{
"interface": "lo",
"flags": [
"UP",
"LOOPBACK",
"RUNNING"
],
"mtu": 65536,
"inet": "127.0.0.1",
"netmask": "255.0.0.0",
"prefixlen": 8,
"inet6": "::1",
"group": [
"local"
],
"txqueuelen": 1000
},
{
"interface": "eth0",
"flags": [
"UP",
"BROADCAST",
"MULTICAST",
"RUNNING"
],
"mtu": 1500,
"inet": "192.168.1.5",
"netmask": "255.255.255.0",
"broadcast": "192.168.1.255",
"prefixlen": 24,
"inet6": "fe80::a00:27ff:fe4e:66a1",
"group": [
"global"
],
"txqueuelen": 1000
}
]
Use case 4: Output Pretty JSON via Magic Syntax
Code:
jc -p ifconfig
Motivation:
This use case combines the simplicity of magic syntax with the readability of pretty-printed JSON. It helps maintain a clean command line or script by reducing verbosity while ensuring output is easy to comprehend. It is particularly advantageous when you need to quickly review command output without writing the result to a file or using additional formatting tools.
Explanation:
jc
: Executes the JSON conversion process.-p
: Enabling pretty-printing for improved readability.ifconfig
: Specifies the command to run and convert, usingjc
to process the output directly.
Example Output:
[
{
"interface": "lo",
"flags": [
"UP",
"LOOPBACK",
"RUNNING"
],
"mtu": 65536,
"inet": "127.0.0.1",
"netmask": "255.0.0.0",
"prefixlen": 8,
"inet6": "::1",
"group": [
"local"
],
"txqueuelen": 1000
},
{
"interface": "eth0",
"flags": [
"UP",
"BROADCAST",
"MULTICAST",
"RUNNING"
],
"mtu": 1500,
"inet": "192.168.1.5",
"netmask": "255.255.255.0",
"broadcast": "192.168.1.255",
"prefixlen": 24,
"inet6": "fe80::a00:27ff:fe4e:66a1",
"group": [
"global"
],
"txqueuelen": 1000
}
]
Conclusion
The jc
command is an efficient utility that bridges the traditional command-line interface with modern applications by converting command output into the popular JSON format. The various use cases discussed demonstrate the flexibility and readability jc
introduces to your workflow, making data easier to parse and use in different contexts. Whether you’re simplifying script output, improving monitoring systems, or simply making data easier to work with, jc
offers a straightforward solution to output conversion challenges.