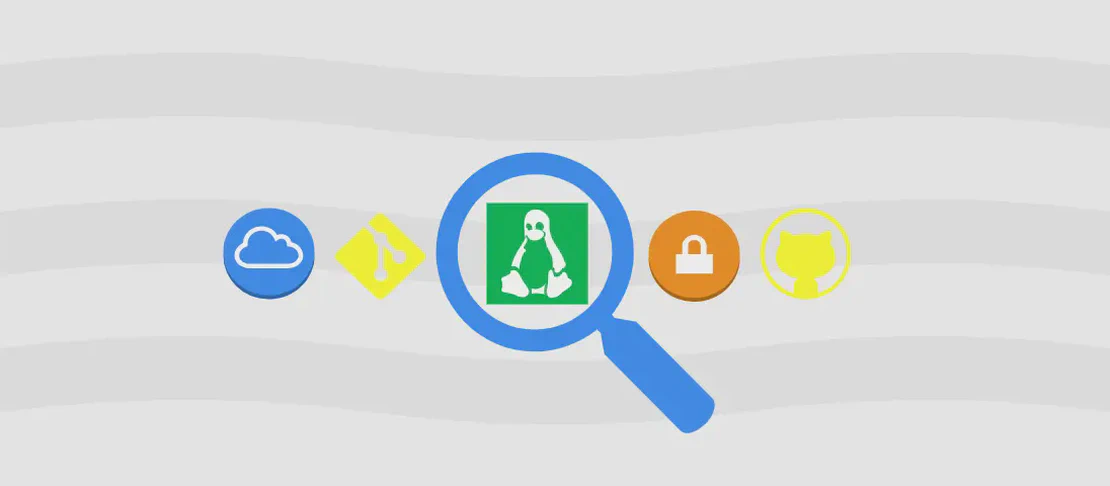
How to Use the Command 'jdeps' (with examples)
The jdeps
command is a powerful tool that comes bundled with the Java Development Kit (JDK). It is used to analyze the dependencies of Java class files and JAR files. By providing insights into which classes are used by your applications and which packages they depend on, jdeps
can be instrumental in understanding and managing your code’s architecture. It’s particularly useful for tasks such as troubleshooting classpath issues, assessing the modularity of your Java applications, and preparing for migration to newer Java versions.
Use case 1: Analyze the dependencies of a .jar
or .class
file
Code:
jdeps path/to/filename.class
Motivation:
When working with Java applications, it’s often critical to understand which other classes a particular class depends upon. This knowledge can help developers troubleshoot issues, optimize memory usage, or refactor code for modularization. Analyzing dependencies can also assist in identifying unnecessary imports, thus making the code cleaner and more efficient.
Explanation:
jdeps
: The command-line tool used for Java class dependency analysis.path/to/filename.class
: The path to the.class
file whose dependencies you wish to analyze. This could also be a.jar
file for analyzing dependencies at a broader level.
Example Output:
example.com/app/Main (Main.java)
-> java.base/java.io
-> java.base/java.util
-> java.sql/java.sql
The output indicates that the Main
class depends on classes from the java.io
, java.util
, and java.sql
packages.
Use case 2: Print a summary of all dependencies of a specific .jar
file
Code:
jdeps path/to/filename.jar -summary
Motivation:
A summary of dependencies is essential for a high-level overview of a JAR file’s external dependencies. This can be particularly valuable during the early phase of application migration to newer platform versions or modules, as it quickly highlights the namespaces and packages upon which your application relies.
Explanation:
jdeps
: The tool being used to analyze dependencies.path/to/filename.jar
: Path to the JAR file for which you want a summary of dependencies.-summary
: This flag instructsjdeps
to generate a summary rather than a detailed analysis, thus providing a compact view of dependencies.
Example Output:
JAR file: myapplication.jar
-> java.base
-> java.logging
Here, the summary shows that the JAR file myapplication.jar
depends primarily on the java.base
and java.logging
modules.
Use case 3: Print all class-level dependencies of a .jar
file
Code:
jdeps path/to/filename.jar -verbose
Motivation:
Understanding the specific class-level dependencies within a JAR file is crucial for deep-dive inspections, such as when investigating issues or optimizing the classpath. This detailed view can assist developers in debugging complex problems related to dependency conflicts and in ensuring that all necessary classes are included.
Explanation:
jdeps
: The command for analyzing dependencies.path/to/filename.jar
: Path to the JAR file containing classes whose dependencies need detailed inspection.-verbose
: This option tellsjdeps
to produce a detailed report, listing all the dependencies at the class level.
Example Output:
example.com/app/SomeClass
-> java.base/java.io.File
-> java.base/java.lang.String
-> java.base/java.util.List
-> java.logging/java.util.logging.Logger
The detailed output specifies the exact classes which SomeClass
depends on, allowing for thorough analysis and troubleshooting.
Use case 4: Output the results of the analysis in a DOT file into a specific directory
Code:
jdeps path/to/filename.jar -dotoutput path/to/directory
Motivation:
Exporting analysis results into a DOT file format is highly beneficial for visualization purposes. Developers can use various graphing tools to generate dependency graphs, providing intuitive and easy-to-understand visual representations of complex dependency structures. Such visual tools aid in comprehending and communicating the architecture and dependencies within the development team.
Explanation:
jdeps
: The dependency tool.path/to/filename.jar
: Specifies the JAR file for which dependencies should be analyzed.-dotoutput
: This flag designates that output should be formatted as a DOT file, a graph description language.path/to/directory
: The directory where the DOT file will be saved.
Example Output:
No direct console output; instead, a file is generated in the specified directory. The file might look like this in DOT syntax:
digraph G {
"example.com/app/Main" -> "java.base/java.lang.String"
"example.com/app/Main" -> "java.base/java.io.File"
}
Use case 5: Display help
Code:
jdeps --help
Motivation:
Any software tool requires a clear understanding of its options and commands. The --help
flag is the first step for new users to familiarize themselves with jdeps
functionalities or for seasoned users to refresh their memory regarding specific command options and flags.
Explanation:
jdeps
: The command for which help is being requested.--help
: This flag outputs a detailed description of all available command options directly to the console, helping users to better understand and utilizejdeps
.
Example Output:
Usage: jdeps <options> <jar | class file | directory>...
where options include:
--help Print this help message
-summary Print dependency summary only
-verbose Print all dependencies
-dotoutput <dir> Generate DOT file in specified directory
Conclusion:
The jdeps
command is a versatile tool that serves to analyze and understand Java dependencies effectively. Whether it’s for code optimization, troubleshooting, or migrating applications, jdeps
provides valuable insights through various levels of analysis. As illustrated through the examples above, developers can leverage jdeps
to address different needs ranging from summaries to detailed class-level reporting and even formatted exports for visualization. This makes jdeps
an essential command in the toolkit of any Java developer aiming to maintain cleaner, more manageable codebases.