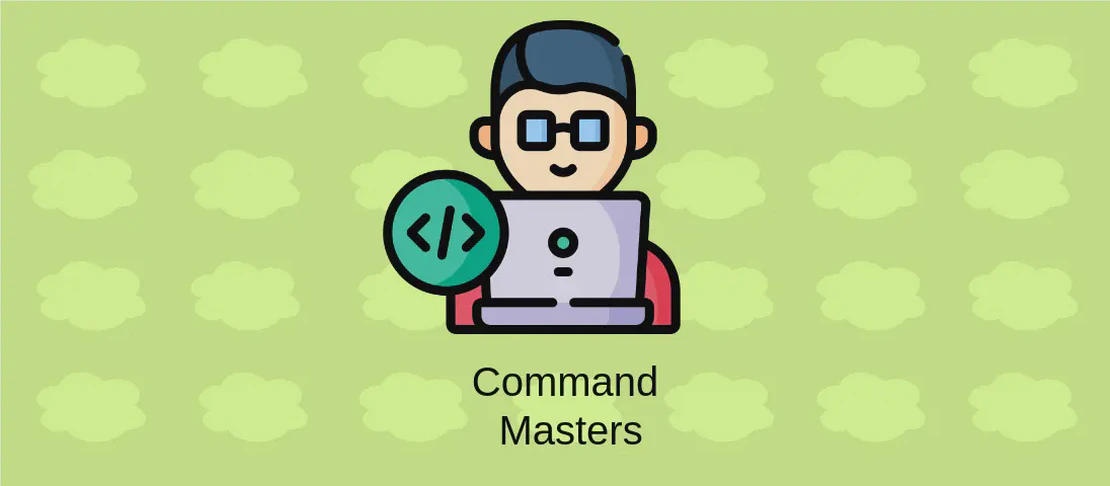
jello (with examples)
Pretty-print JSON or JSON-Lines data
Code:
cat file.json | jello
Motivation:
When dealing with large amounts of JSON or JSON-Lines data, it can be challenging to read and understand the structure. Pretty-printing the data makes it more readable and easier to navigate.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor.- No additional arguments are provided, so jello will pretty-print the JSON data from stdin to stdout.
Example Output:
{
"key1": "value1",
"key2": "value2"
}
{
"key3": "value3",
"key4": "value4"
}
Output a schema of JSON or JSON-Lines data
Code:
cat file.json | jello -s
Motivation:
Determining the structure of JSON or JSON-Lines data is valuable when working with large datasets or debugging issues. Outputting the schema helps identify the available keys and their types.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor.-s
is the argument to instruct jello to output the schema of the JSON data.
Example Output:
{
"key1": "string",
"key2": "number"
}
Output all elements from arrays or all values from objects
Code:
cat file.json | jello -l
Motivation:
In some cases, it’s necessary to extract all the elements from arrays or all the values from objects in JSON or JSON-Lines data. This can be useful for further processing or analysis.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor.-l
is the argument to instruct jello to output all elements from arrays or all values from objects.
Example Output:
"value1"
"value2"
"value3"
"value4"
Output the first element
Code:
cat file.json | jello _[0]
Motivation:
In scenarios where only the first element of JSON or JSON-Lines data is needed, extracting it can help reduce unnecessary processing or focus on essential information.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor._[0]
is an expression that extracts the first element (index 0) from the input data.
Example Output:
{
"key1": "value1",
"key2": "value2"
}
Output the value of a given key for each element
Code:
cat file.json | jello '[i.key_name for i in _]'
Motivation:
When specific information needs to be extracted from each element in a JSON array or JSON-Lines data, it can be useful to output the values of a particular key. This facilitates processing or analysis based on that key’s value.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor.[i.key_name for i in _]
is a list comprehension that extracts the value of the keykey_name
for each element in the JSON data.
Example Output:
"value1"
"value2"
Output the value of multiple keys as a new JSON object
Code:
cat file.json | jello '{"my_new_key": _.key_name, "my_other_key": _.other_key_name}'
Motivation:
In scenarios where a new JSON object needs to be created from existing keys, combining and renaming them, this command can be useful. It allows for generating a JSON object with specific key-value pairs based on the existing data.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor.{"my_new_key": _.key_name, "my_other_key": _.other_key_name}
is a JSON object that creates new keysmy_new_key
andmy_other_key
with corresponding values extracted from the original JSON data.
Example Output:
{
"my_new_key": "value1",
"my_other_key": "value2"
}
Output the value of a given key to a string (and disable JSON output)
Code:
cat file.json | jello -r '"some text: " + _.key_name'
Motivation:
In certain cases, it may be necessary to concatenate the value of a given key with a string or perform a custom operation. This command allows for more flexibility in manipulating the data and generating customized output.
Explanation:
cat file.json
reads the contents of the file.json file.|
is the pipe operator, which takes the output from the previous command and passes it as input to the next command.jello
is the command-line JSON processor.-r
is the argument to instruct jello to disable the default JSON output and instead print a string.'"some text: " + _.key_name'
is a string concatenation expression that combines the value of the keykey_name
with the text “some text”.
Example Output:
"some text: value1"
By understanding and utilizing the various functionalities of the jello command-line JSON processor, you can effectively manipulate, extract, and transform JSON or JSON-Lines data to meet your specific needs. Whether it’s for readability, analysis, or data transformation, jello provides a flexible and powerful tool for working with JSON data.