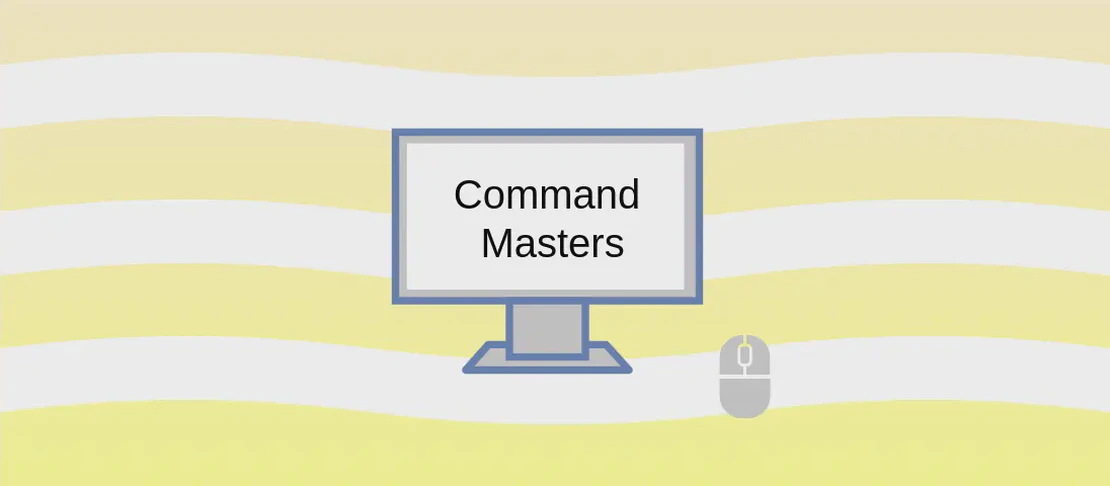
How to use the command 'jest' (with examples)
Jest is a zero-configuration JavaScript testing platform that allows developers to write effective tests for their applications without having to spend time on complex setups. It streamlines the process of running and managing tests through its simple command interface, providing tools for mocking, assertion, and more.
Use case 1: Run all available tests
Code:
jest
Motivation:
Running all available tests is a fundamental action for developers who need to ensure that the entire codebase is functioning as expected. This is typically done before pushing changes to a shared repository, to verify that no unforeseen bugs have been introduced.
Explanation:
Without any arguments, running jest
will execute all test files it discovers in the project. Jest intelligently identifies these files by their naming conventions, such as files ending in .test.js
or .spec.js
, as well as those residing in a __tests__
directory.
Example Output:
PASS ./sum.test.js
PASS ./subtract.test.js
Test Suites: 2 passed, 2 total
Tests: 4 passed, 4 total
Snapshots: 0 total
Time: 3.412s
Use case 2: Run the test suites from the given files
Code:
jest path/to/file1 path/to/file2
Motivation:
At times, developers may only need to test specific parts of a codebase, especially if they are making changes to a particular module. Opting to run tests from specific files provides a faster feedback loop compared to running the entire test suite.
Explanation:
The command jest path/to/file1 path/to/file2
specifies exact file paths which contain the test suites to be executed. This command overrides the default behavior of running all tests and focuses solely on the specified files.
Example Output:
PASS path/to/file1.js
PASS path/to/file2.js
Test Suites: 2 passed, 2 total
Tests: 5 passed, 5 total
Snapshots: 0 total
Time: 1.345s
Use case 3: Run the test suites from files within the current and subdirectories, whose paths match the given regular expression
Code:
jest regular_expression1 regular_expression2
Motivation:
When your project grows larger, you might need to selectively run tests from areas of interest identified by a pattern in their file paths. This can be especially useful during integration testing or when refactoring large systems by breaking tests down into more manageable subsets.
Explanation:
Using a regular expression via jest
, the command matches and runs tests from files whose paths fit the pattern specified. This enables complex filtering based on file structure or naming conventions, allowing greater flexibility in test execution strategies.
Example Output:
PASS src/components/__tests__/component.test.js
PASS src/pages/__tests__/page.test.js
Test Suites: 2 passed, 2 total
Tests: 6 passed, 6 total
Snapshots: 0 total
Time: 2.789s
Use case 4: Run the tests whose names match the given regular expression
Code:
jest --testNamePattern regular_expression
Motivation:
Sometimes, developers need to run specific tests based on the functionality they are working on, especially in a test suite that covers a wide array of functionalities. By targeting tests with names that match certain patterns, developers can quickly ascertain if a specific feature is functioning correctly.
Explanation:
By including the --testNamePattern
argument followed by a regular expression, Jest focuses on executing only those tests whose names conform to the specified pattern. This is highly useful for isolating specific functionality within larger test suits.
Example Output:
PASS ./filter_tests/desiredFunctionality.test.js
Test Suites: 1 passed, 1 total
Tests: 3 passed, 3 total
Snapshots: 0 total
Time: 1.567s
Use case 5: Run test suites related to a given source file
Code:
jest --findRelatedTests path/to/source_file.js
Motivation:
When modifications are made to a specific source file, it’s prudent to confirm that all related test suites still perform correctly. This command ensures any direct or indirect dependencies to that source file are verified through testing.
Explanation:
The --findRelatedTests
option allows jest to identify and run test suites that are related to the specified source file. This feature is useful for ensuring that changes to core functionality do not adversely affect dependent code.
Example Output:
PASS ./tests/relatedTestSuite1.test.js
PASS ./tests/relatedTestSuite2.test.js
Test Suites: 2 passed, 2 total
Tests: 8 passed, 8 total
Snapshots: 0 total
Time: 2.456s
Use case 6: Run test suites related to all uncommitted files
Code:
jest --onlyChanged
Motivation:
During the development phase, it is often desirable to run test suites related to files that have been changed but not yet committed. This ensures that any local amendments do not break any functionality before they are stored in version control.
Explanation:
The --onlyChanged
flag tells Jest to detect uncommitted changes and execute test suites related to those files. This is particularly useful in continuous integration pipelines where test coverage of recent changes is a key concern.
Example Output:
PASS ./tests/changedFileTestSuite.test.js
Test Suites: 1 passed, 1 total
Tests: 5 passed, 5 total
Snapshots: 0 total
Time: 1.201s
Use case 7: Watch files for changes and automatically re-run related tests
Code:
jest --watch
Motivation:
For an interactive real-time feedback loop while coding, running Jest in watch mode is invaluable. This allows developers to continue coding while tests automatically execute and report on functionality affected by their changes.
Explanation:
The --watch
command puts Jest into listening mode, where it watches files for changes and automatically runs tests relevant to those changes. This seamless integration into the development process boosts productivity by providing immediate confirmation of functionality.
Example Output:
PASS ./liveTestSuite.test.js
Test Suites: 1 passed, 1 total
Tests: 7 passed, 7 total
Snapshots: 0 total
Time: 0.923s
Watch Usage: Press 'a' to run all tests, 'q' to quit watch mode
Use case 8: Display help
Code:
jest --help
Motivation:
Jest is packed with numerous options and configurations, which can be daunting for new users or those looking to explore its full potential. Displaying the help documentation is an excellent way to view all available commands and options.
Explanation:
The --help
command provides an extensive list of all Jest options and features, along with brief descriptions. By using the help command, users can understand how to customize and utilize Jest effectively in their development workflows.
Example Output:
Usage: jest [--config=<pathToConfigFile>] [<regexForTestFiles>]
Options:
--help Show help
--version Print version
...
Conclusion:
Jest serves as a powerful tool in a developer’s toolbox, ensuring code quality and functionality through structured and flexible testing processes. Each command and parameter offers tailored capabilities to suit specific needs during the development cycle, enhancing reliability and efficiency in software testing.