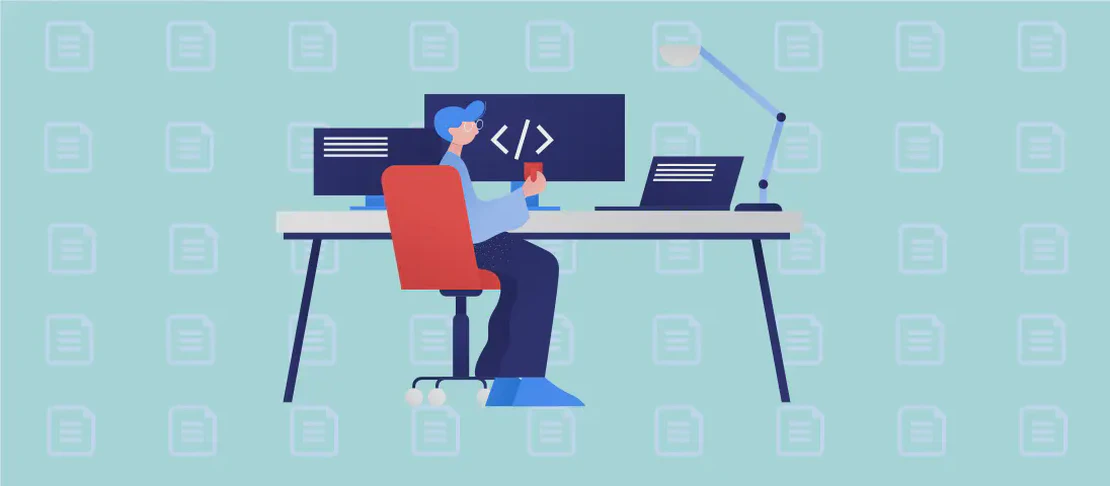
How to Use the Command 'jmap' (with Examples)
The jmap
tool is an integral part of Java’s suite of diagnostic tools, used to analyze and monitor various aspects of memory in Java applications. Specifically, it provides insights into memory footprint, heap usage, shared object mappings, and more. These capabilities are crucial for developers and system administrators aiming to optimize Java applications, troubleshoot memory leaks, and ensure overall application performance. The key advantage of jmap
is its ability to offer detailed memory-related statistics, which are instrumental in sustained application maintenance and swift resolution of memory issues.
Use Case 1: Print Shared Object Mappings for a Java Process
Code:
jmap java_pid
Motivation: By listing shared object mappings, developers and system administrators can gain visibility into the shared libraries loaded into a Java process. This information can be beneficial for debugging, as it helps identify the memory sections and related dependencies at play within a Java application. Understanding shared object mappings is essential when analyzing complex systems that depend on numerous external libraries or modules.
Explanation:
In this command, jmap
is followed by a single argument, java_pid
, which stands for the Java Process ID. This ID specifies the active process whose memory map you wish to investigate. The output resembles that produced by the pmap
command in Linux, detailing the memory arrangements of the process.
Example Output:
47870: /usr/bin/java
00400000 700K r-x-- /usr/bin/java
006B0000 10K rw--- /usr/bin/java
006B2000 4K rw--- /usr/bin/java
...
Use Case 2: Print Heap Summary Information
Code:
jmap -heap filename.jar java_pid
Motivation: Heap summary information is vital for understanding how your Java application uses memory. This includes the distribution and usage of various memory spaces, such as Eden, Survivor, and Old Generation spaces. Monitoring heap usage assists in tuning garbage collection processes and identifying potential memory bottlenecks that could affect application performance.
Explanation:
The command jmap -heap
comprises several elements:
-heap
: This option triggersjmap
to produce the summary of heap usage.filename.jar
: This denotes the JAR file name running as part of the Java process. While not necessary for the command to function, it provides context to the user-generated output.java_pid
: The Java Process ID for which the heap summary needs to be generated. It specifies the exact instance to analyze, particularly useful when multiple instances are running.
Example Output:
using thread-local object allocation.
Garbage-First (G1) GC with 16 thread(s)
Heap Configuration:
MinHeapFreeRatio = 40
MaxHeapFreeRatio = 70
MaxHeapSize = 1073741824 (1024.0MB)
NewSize = 1363144 (1.3MB)
...
Use Case 3: Print Histogram of Heap Usage by Type
Code:
jmap -histo java_pid
Motivation: The histogram of heap usage is a diagnostic tool that helps you discern what types of objects occupy the most memory in a Java application. This insight is critical for developers working to optimize object usage and minimize memory waste.
Explanation:
In this command, jmap -histo
provides:
-histo
: This flag commandsjmap
to generate a histogram display of heap usage by the type of objects. This option is crucial for detailed memory profiling.java_pid
: Represents the Process ID of the Java application being evaluated, ensuring the generated histogram corresponds to the correct running process.
Example Output:
num #instances #bytes class name
----------------------------------------------
1: 101 2300208 [C
2: 30072 1375616 [B
3: 28860 923456 java.lang.String
...
Use Case 4: Dump Contents of the Heap into a Binary File for Analysis with jhat
Code:
jmap -dump:format=b,file=path/to/file java_pid
Motivation:
Dumping the contents of the heap to a file allows for offline analysis with tools like jhat
or other heap analyzers. This capability is particularly important for post-mortem analysis of application crashes or for capturing a specific state of the application for performance analysis.
Explanation:
The command jmap -dump:format=b,file=path/to/file java_pid
breaks down as:
-dump:format=b
: This specifier indicates a heap dump should be created in binary format, suitable for analysis by utilities likejhat
.file=path/to/file
: The path indicates where the heap dump file will be stored. It’s advisable to choose a location with adequate space.java_pid
: The PID of the Java application whose heap content is to be exported, ensuring the dump reflects the correct process.
Example Output: (No console output; a binary dump file is created at the specified location)
Use Case 5: Dump Live Objects of the Heap into a Binary File for Analysis with jhat
Code:
jmap -dump:live,format=b,file=path/to/file java_pid
Motivation: Focusing the heap dump on live objects only provides a more efficient and focused dataset for analysis, cutting unnecessary clutter of garbage-collected objects. This is especially useful in applications with high object turnover and when memory analysis needs to spotlight what’s active at snapshot time.
Explanation:
The command jmap -dump:live,format=b,file=path/to/file java_pid
contains:
-dump:live
: This directive specifies the inclusion of only objects that are currently live, meaning those that are reachable and not subject to garbage collection.format=b
: As with previous examples, this denotes the creation of a binary format dump file.file=path/to/file
: The file path suggests where the resulting file will be stored.java_pid
: Stipulates which running Java process to target for the heap dump.
Example Output: (No console output; a binary dump file with live objects is saved to the specified location)
Conclusion:
The jmap
command is an indispensable tool for Java developers and systems administrators working to optimize and troubleshoot Java applications. Its capabilities extend from providing detailed memory mappings to dumping comprehensive heap analyses. With these examples, one can delve deeper into the intricacies of Java memory management, enabling enhanced application performance and stability. Understanding these use cases equips users with the knowledge necessary to tackle a variety of memory-related challenges in Java environments.