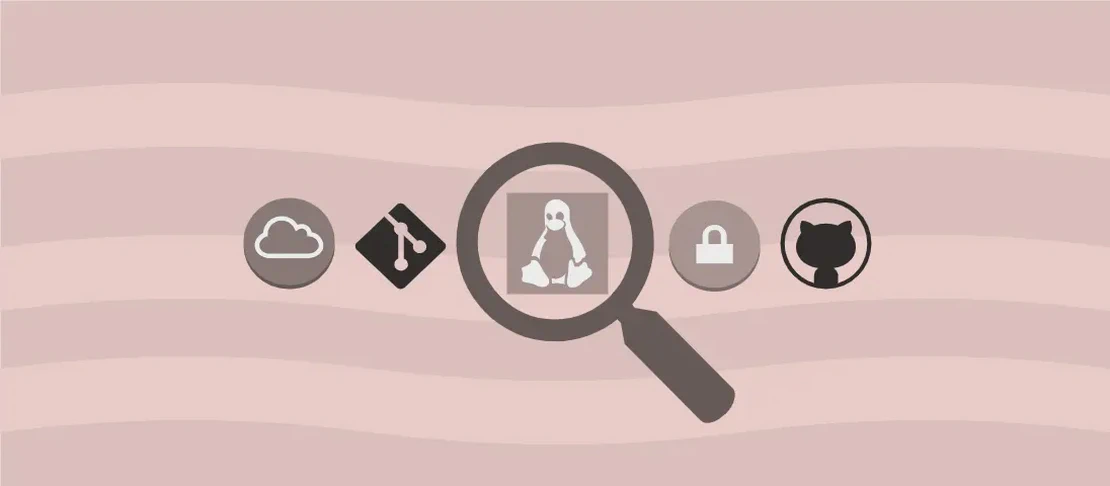
How to use the command 'julia' (with examples)
Julia is a high-level, high-performance dynamic programming language, particularly well-suited for numerical and technical computing tasks. Due to its wide array of features, Julia is popular in fields such as data science, machine learning, and scientific research. The command-line interface for Julia empowers users to interact with the language in various ways, from running individual scripts to executing code snippets and leveraging multi-threading for parallel computations.
Use case 1: Start a REPL (interactive shell)
Code:
julia
Motivation:
The Julia REPL (Read-Eval-Print Loop) provides an interactive shell where users can type and execute Julia commands on-the-fly. This functionality is particularly useful when testing small chunks of code, experimenting with different programming techniques, or learning the language. By offering real-time feedback and error messages, the REPL enhances the programming experience by allowing immediate test and iteration, fostering a dynamic workflow where you can develop and refine your code incrementally.
Explanation:
julia
: Launches the Julia interactive REPL. No additional arguments are required because this command is solely focused on starting the interactive environment for Julia programming.
Example output:
_
_ _ _(_)
(_) | (_) |
_ _ _| |_| |_
| | | | | | | _|
| | |_| | | | |
_/ |\__,_|_|_|\__|
|__/
You are greeted with the Julia prompt which signals that Julia is ready for input.
Use case 2: Execute a Julia program and exit
Code:
julia program.jl
Motivation:
Running a Julia script directly from the command line provides an efficient way to execute programs that have been pre-written and saved in a file. This use case is ideal for deploying applications or running computations that have been tested and finalized. Scripting allows for reproducibility and automation, making it well-suited for data processing pipelines, simulations, or repeated analyses.
Explanation:
julia
: Calls the Julia interpreter to execute a program.program.jl
: Denotes the file name of the Julia script to be executed. The.jl
extension is standard for Julia files, indicating a file that contains Julia code.
Example output:
Assuming program.jl
prints “Hello World!”:
Hello World!
Use case 3: Execute a Julia program that takes arguments
Code:
julia program.jl arguments
Motivation:
Passing arguments to a Julia script via the command line is extremely useful for creating flexible and dynamic programs. This functionality allows you to customize the behavior of a script without modifying its codebase, thereby supporting a wide array of input scenarios or configurations. This capability is especially beneficial for batch processing of datasets or running parameterized simulation models.
Explanation:
julia
: Initiates the Julia interpreter.program.jl
: Represents the Julia script to be executed.arguments
: Placeholder for one or more arguments that the executed program will process. These could be anything from file names to configuration settings, depending on how the program is designed.
Example output:
If program.jl
contains code that prints each argument, running julia program.jl arg1 arg2
could output:
arg1
arg2
Use case 4: Evaluate a string containing Julia code
Code:
julia -e 'julia_code'
Motivation:
This command’s functionality captures the need for quick, inline evaluation of Julia code directly from the command line. It is especially helpful for executing short, one-off tasks or integrating within shell scripts for computational operations. This allows users to leverage Julia’s powerful numerical capabilities for ad-hoc tasks without needing to create and save a full script file.
Explanation:
julia
: Calls the Julia interpreter.-e
: A switch indicating that what follows is a code snippet to be executed rather than a file name.'julia_code'
: The snippet of Julia code to be executed, encapsulated in single quotes.
Example output:
Executing julia -e 'println("Quick calculation: ", 3 + 4)'
outputs:
Quick calculation: 7
Use case 5: Evaluate a string of Julia code, passing arguments to it
Code:
julia -e 'for x in ARGS; println(x); end' arguments
Motivation:
This use case extends the inline execution capabilities by incorporating command-line arguments into single lines of Julia code. It can be beneficial for scenarios where input data dynamically influences computations, such as filtering lists, doing mathematical transformations, or modifying text strings. It allows scripting of more responsive and adaptable executions directly from the terminal.
Explanation:
julia
: Initializes the interpreter.-e
: Signifies that the following text is a snippet of Julia code.'for x in ARGS; println(x); end'
: A loop in Julia that iterates over each argument passed, printing each one.arguments
: Denotes one or more parameters provided after the code snippet, which are accessible within the Julia string asARGS
.
Example output:
Running julia -e 'for x in ARGS; println(x); end' argA argB
results in:
argA
argB
Use case 6: Evaluate an expression and print the result
Code:
julia -E '(1 - cos(pi/4))/2'
Motivation:
The use of the -E
option allows for the evaluation of mathematical expressions with the result printed directly. This feature is particularly handy for quick and straightforward calculations involving complex numbers, linear algebra, or advanced calculus, serving as an efficient replacement for a calculator with the added power of Julia’s scientific computing capabilities.
Explanation:
julia
: Calls the Julia interpreter.-E
: Designates the following argument as an expression to evaluate.'(1 - cos(pi/4))/2'
: Represents the mathematical expression to be evaluated. The result of this computation will be printed out.
Example output:
For the given expression:
0.07612046748871326
Use case 7: Start Julia in multithreaded mode, using N threads
Code:
julia -t N
Motivation:
Julia excels in performance by supporting parallel computations. By leveraging multi-threaded computing, this use case allows programs to run tasks concurrently, significantly boosting performance, especially on multi-core processors. This is highly relevant for high-performance computing tasks, simulations, data science workloads, and more, where time-to-completion and efficiency are crucial factors.
Explanation:
julia
: Launches the interpreter.-t N
: Option that specifies launching the Julia environment with N threads, where N stands for the number of threads you wish to utilize. Increasing thread count can lead to performance improvements in computations designed to take advantage of parallelism.
Example output:
If you execute julia -t 4
, the Julia session starts with four threads available for your program’s parallel computations. While no direct output signifies starting this mode, you can validate multithreading availability within Julia code through the constant Threads.nthreads()
, which will yield:
4
Conclusion:
Julia provides a multitude of versatile command-line options tailored for various needs, from interactive sessions to inline code execution, parallel computing, and running entire programs with dynamic inputs. Understanding and using these functionalities effectively unleashes the full potential of Julia’s computing power, making it a robust choice for scientific computing and data-intensive applications. Through this guide, readers should feel better equipped to harness these command capabilities in their programming endeavors.