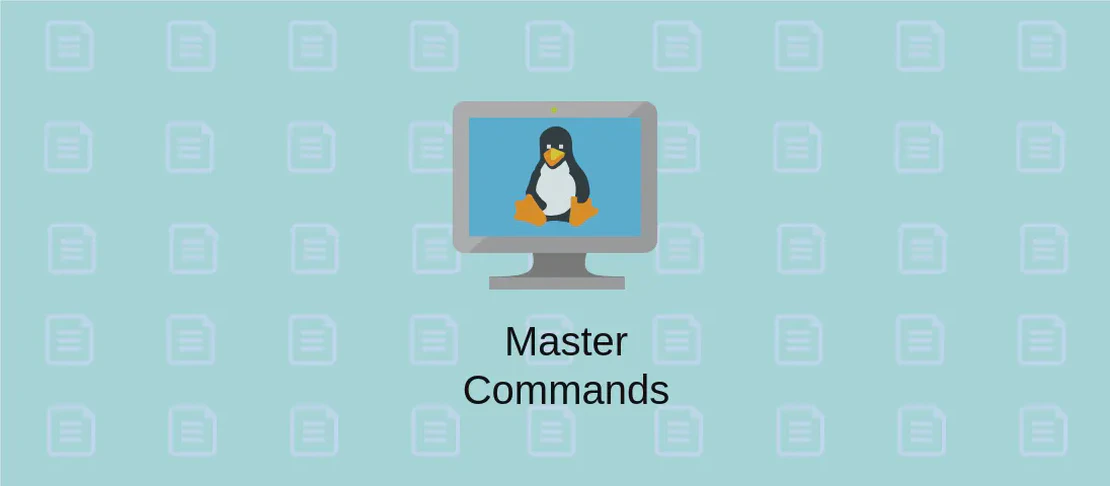
How to use the command jwt (with examples)
JSON Web Tokens (JWTs) are a compact and self-contained way to securely transmit information between parties as a JSON object. The jwt
command-line tool allows users to work with JWTs by providing various functionalities such as decoding, encoding, and using different encryption algorithms. In this article, we will explore the different use cases of the jwt
command.
Use case 1: Decode a JWT
Code:
jwt decode jwt_string
Motivation: Decoding a JWT allows users to extract the information encoded within the token and inspect its content. This can be useful for debugging or understanding the token’s payload.
Explanation:
jwt decode
: This command instructs thejwt
tool to decode a JWT.jwt_string
: The JWT string that needs to be decoded.
Example Output:
{
"alg": "HS256",
"typ": "JWT"
}
Use case 2: Decode a JWT as a JSON string
Code:
jwt decode -j jwt_string
Motivation: Decoding a JWT as a JSON string provides a more readable output by displaying the token’s properties and values in a well-structured format.
Explanation:
jwt decode
: This command instructs thejwt
tool to decode a JWT.-j
: This flag specifies that the output should be in JSON format.jwt_string
: The JWT string that needs to be decoded.
Example Output:
{
"alg": "HS256",
"typ": "JWT"
}
Use case 3: Encode a JSON string to a JWT
Code:
jwt encode --alg HS256 --secret 1234567890 'json_string'
Motivation: Encoding a JSON string to a JWT enables the creation of a new JWT with a specific algorithm and secret key. This is useful when generating a token for authentication or authorization purposes.
Explanation:
jwt encode
: This command instructs thejwt
tool to encode a JSON string to a JWT.--alg HS256
: Specifies the encryption algorithm to be used. In this example,HS256
is used.--secret 1234567890
: Specifies the secret key to be used for encryption.'json_string'
: The JSON string that needs to be encoded into a JWT.
Example Output:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.lBpFTJpgMFzToXy4upi-cwEwHNC1jDcTee4iLKKYO7I
Use case 4: Encode key pair payload to JWT
Code:
jwt encode --alg HS256 --secret 1234567890 -P key=value
Motivation: Encoding a key-value pair payload to a JWT allows users to include custom data as part of the token. This can be useful when passing additional information as claims in the token.
Explanation:
jwt encode
: This command instructs thejwt
tool to encode a key pair payload to a JWT.--alg HS256
: Specifies the encryption algorithm to be used. In this example,HS256
is used.--secret 1234567890
: Specifies the secret key to be used for encryption.-P key=value
: Specifies the key-value pair to be included in the token payload. Here,key
is the name of the key, andvalue
is the corresponding value.
Example Output:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJrZXkiOiJ2YWx1ZSJ9.M4SzCJRbAjJAq1OIAlqPP0HnOeNRvGMy9sfZJK4o11k
Conclusion:
The jwt
command-line tool provides a convenient way to work with JSON Web Tokens. By understanding the different use cases of the jwt
command, users can easily decode, encode, and manipulate JWTs according to their specific requirements. Whether verifying the integrity of a received token, generating a new one for authentication, or adding customized claims, the jwt
command offers a versatile set of functionalities to work with JWTs in a secure manner.