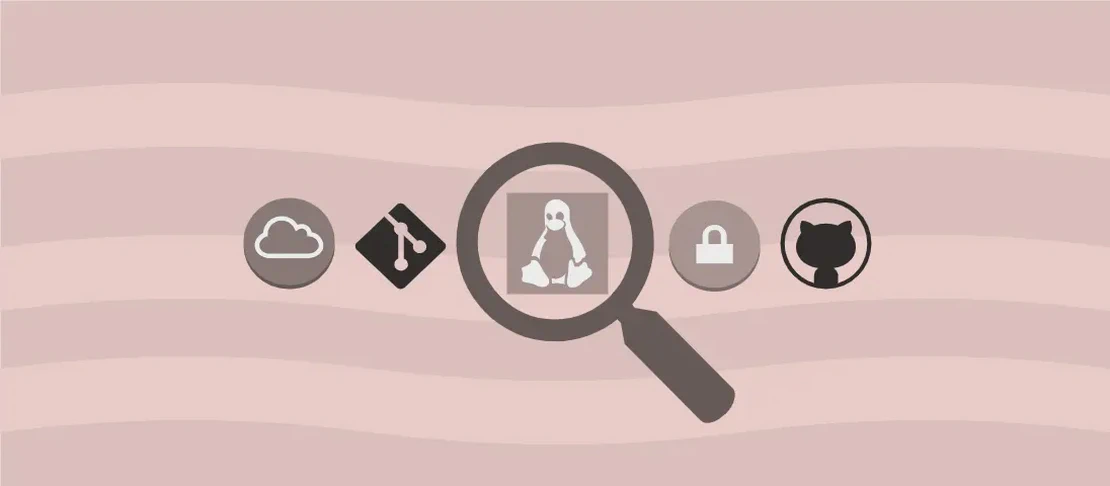
How to Use the Command 'kdialog' (with Examples)
- Linux
- December 17, 2024
The kdialog
command is a versatile tool used to display graphical dialog boxes within shell scripts, specifically designed for KDE desktop environments. It allows developers and system administrators to interact with users via dialogs such as message boxes, input prompts, file choosers, and more. This makes it an essential tool for creating interactive scripts that require user input or provide feedback based on user responses. Below, we’ll explore various use cases of this command to understand its practical applications and functionality.
Open a Dialog Box Displaying a Specific Message
Code:
kdialog --msgbox "Hello, World!" "This is a detailed message."
Motivation: Using a message box is a simple and effective way to communicate with users. Whether you need to inform users about the success of an operation or provide instructions, a message box ensures that important information is conveyed in a straightforward manner.
Explanation:
--msgbox
: This option tellskdialog
to open a message box."Hello, World!"
: This is the primary message displayed in the dialog."This is a detailed message."
: This optional second argument allows you to provide additional details or context related to the primary message.
Example Output: A dialog box opens with the main message “Hello, World!” and a detailed message underneath, stating “This is a detailed message.”
Open a Question Dialog with Yes and No Buttons
Code:
kdialog --yesno "Do you want to proceed?"
Motivation: Question dialogs are invaluable when you need users to confirm actions. By presenting users with a choice, you allow them to take control over their decisions, reducing the risk of accidental actions.
Explanation:
--yesno
: This sets up a dialog with “Yes” and “No” options."Do you want to proceed?"
: This message prompts the user to make a decision.
Example Output:
A dialog appears asking “Do you want to proceed?” with two buttons: “Yes” and “No.” The program returns 0
if “Yes” is selected or 1
if “No” is chosen.
Open a Warning Dialog with Yes, No, and Cancel Buttons
Code:
kdialog --warningyesnocancel "Unsaved changes detected. Continue without saving?"
Motivation: Warning dialogs are essential for informing users of potential consequences before proceeding with an action. They add another layer of security and assurance by providing options to abort, proceed, or reconsider.
Explanation:
--warningyesnocancel
: This option sets up a more detailed dialog with three options: Yes, No, and Cancel."Unsaved changes detected. Continue without saving?"
: The warning message indicating the potential risk of data loss.
Example Output:
A dialog box appears saying “Unsaved changes detected. Continue without saving?” with buttons for Yes, No, and Cancel. The program returns 0
, 1
, or 2
based on the user’s choice.
Open an Input Dialog Box and Print the Input to stdout
Code:
kdialog --inputbox "Enter your name:" "John Doe"
Motivation: Input dialogs are crucial when user-specific data is required during a script’s execution. They enable a personalized interaction by accepting user input and utilizing it further in the script.
Explanation:
--inputbox
: This creates an input dialog for text entry."Enter your name:"
: The prompt message displayed to the user."John Doe"
: An optional default text that appears in the input field, providing a hint or example.
Example Output:
An input box that says “Enter your name:” with “John Doe” in the text field. Upon pressing OK, the entered name is printed to stdout
.
Open a Dialog to Prompt for a Password
Code:
kdialog --password "Enter your password"
Motivation: Password dialogs are secure ways to capture sensitive user data without exposing it, crucial for login validations or secure access scenarios.
Explanation:
--password
: Generates a dialog for secure password entry, where inputted text is masked."Enter your password"
: The prompt message for entering a password.
Example Output:
A password dialog appears with the message “Enter your password,” and input looks like asterisks or bullet points. Upon submission, the password is output to stdout
.
Open a Dialog Containing a Dropdown Menu
Code:
kdialog --combobox "Select your favorite fruit:" "Apple" "Banana" "Cherry"
Motivation: Dropdown menus simplify decision-making by providing a list of predefined choices. They are perfect for selecting from a range of options with ease.
Explanation:
--combobox
: This creates a dropdown with several options."Select your favorite fruit:"
: The label guiding user selection."Apple" "Banana" "Cherry"
: The list of selectable items.
Example Output:
A dropdown menu dialog shows “Select your favorite fruit:” with options Apple, Banana, and Cherry. The selected fruit is printed to stdout
when chosen.
Open a File Chooser Dialog
Code:
kdialog --getopenfilename
Motivation: File chooser dialogs streamline the process of file selection within scripts, enabling users to pick files without manually entering paths, reducing error potential.
Explanation:
--getopenfilename
: Launches a file browser dialog for selecting a file to open.
Example Output:
A file browser window appears. Upon file selection, the full file path is output to stdout
.
Open a Progressbar Dialog and Print a D-Bus Reference
Code:
kdialog --progressbar "Loading..."
Motivation: Progress bars give users visual feedback on the status of ongoing processes, improving user experience by indicating that a task is in progress and offering insights into its completion timeframe.
Explanation:
--progressbar
: Generates a dialog displaying a progress bar."Loading..."
: Describes the process currently occurring.
Example Output:
A dialog with a progress bar under the message “Loading…” appears. A D-Bus reference is output to stdout
, allowing for real-time updates via inter-process communication.
Conclusion
The kdialog
command empowers scripts to engage with users through intuitive graphical interfaces. Each use case discussed highlights distinct functionalities, from simple message notifications to secure password inputs, making kdialog
a powerful addition to any shell scripting toolkit aimed at the KDE environment. By utilizing these dialog functions, developers can create more interactive and user-friendly scripts, enhancing overall user experience.