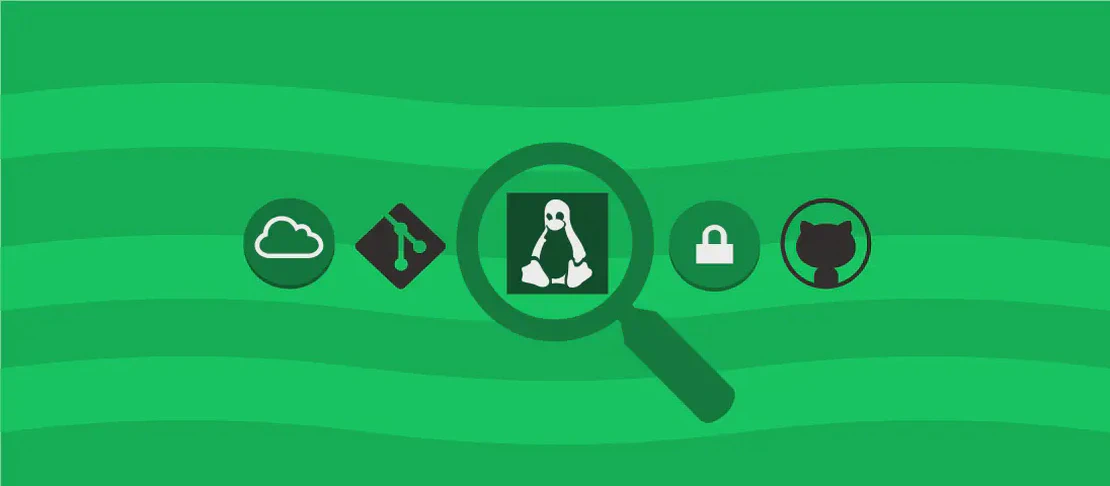
Understanding the 'kill' Command (with Examples)
The ‘kill’ command is a powerful utility in Unix-like operating systems used to send signals to processes. Despite its name, ‘kill’ is not solely about terminating processes; it can also be used to control and communicate with running processes through various signals. While many signals can be intercepted by the target process to perform a clean exit, some, like SIGKILL, are immediate and forceful. This command is essential for system administrators and users who need to manage process behavior on their systems.
Terminate a Program Using the Default SIGTERM (Terminate) Signal
Code:
kill process_id
Motivation:
The default action of the ‘kill’ command is to send the SIGTERM signal, which is a polite request to a process, asking it to terminate itself. This is useful when you want to stop a process that is no longer needed. Unlike SIGKILL, SIGTERM allows the process to perform cleanup operations like closing files and deallocating resources before exiting.
Explanation:
kill
: The command to send a signal.process_id
: The identifier of the process you wish to terminate.
Example Output:
The process may print any cleanup messages as it terminates, or nothing may be visible if it handles termination quietly. The terminal will simply return to a prompt, and the process will cease to run.
List Available Signal Names
Code:
kill -l
Motivation:
Listing all available signals is particularly handy for users who need to understand the different signals they can send to processes. This includes both standard and custom signals. Knowing the range of signals allows for better management of process control.
Explanation:
kill
: The command to send signals.-l
: This option lists all signal names available on the system.
Example Output:
1) SIGHUP 2) SIGINT 3) SIGQUIT 4) SIGILL 5) SIGTRAP 6) SIGABRT
...
This output provides a list of signal names and their corresponding numbers.
Terminate a Program Using the SIGHUP (Hang Up) Signal
Code:
kill -1 process_id
Motivation:
The SIGHUP signal, often used for reloading configuration files in daemons, can be a useful tool for restarting services without completely stopping and starting them. This is ideal for applying configuration changes on the fly.
Explanation:
kill
: The command to send a signal.-1
: The numeric representation of the SIGHUP signal.process_id
: The identifier of the process to receive the signal.
Example Output:
Service daemons typically reload settings or restart connections and may log this action or display messages indicating a reload.
Terminate a Program Using the SIGINT (Interrupt) Signal
Code:
kill -2 process_id
Motivation:
Sending a SIGINT is similar to pressing Ctrl + C in a terminal, which interrupts a running program. This is useful for stopping a process that needs to cleanly release resources and potentially save work before terminating.
Explanation:
kill
: The command to send a signal.-2
: The numeric representation of the SIGINT signal.process_id
: The identifier of the process to interrupt.
Example Output:
The process might display an interruption or cleanup message before exiting.
Signal the Operating System to Immediately Terminate a Program
Code:
kill -9 process_id
Motivation:
The SIGKILL signal is used when a program does not respond to SIGTERM. This immediate termination action is necessary when a process is unresponsive, misbehaving, or consuming excessive resources and needs to be forcefully stopped.
Explanation:
kill
: The command to send a signal.-9
: The numeric representation of the SIGKILL signal.process_id
: The identifier of the process to terminate immediately.
Example Output:
The process is killed without any chance of cleanup, and no output is generated; the process simply disappears.
Signal the Operating System to Pause a Program Until a SIGCONT Signal is Received
Code:
kill -17 process_id
Motivation:
Pausing a process can be beneficial for troubleshooting, managing system resources, or temporarily disabling non-critical processes to focus CPU resources elsewhere. The process remains in memory and can be resumed later.
Explanation:
kill
: The command to send a signal.-17
: The numeric representation of the SIGSTOP signal.process_id
: The identifier of the process to pause.
Example Output:
The process becomes inactive and its status changes to stopped. It will not continue until a SIGCONT signal is sent.
Send a SIGUSR1 Signal to All Processes with the Given GID
Code:
kill -SIGUSR1 -group_id
Motivation:
The SIGUSR1 signal allows users and administrators to send custom notifications or commands to a group of processes based on their group ID (GID). This can be used for communication or triggering specific actions defined within applications.
Explanation:
kill
: The command to send a signal.-SIGUSR1
: Represents a user-defined signal, typically used to trigger custom actions in programs.-group_id
: The group ID of the processes to receive the signal.
Example Output:
Processes may respond to this signal by executing predefined actions or logging messages indicating the signal has been received.
Conclusion:
The ‘kill’ command is an essential tool for managing processes within Unix-like operating systems. Its versatility in sending various signals offers users a broad range of options to control, communicate with, and terminate processes. By understanding each use case and the signals involved, users can leverage ‘kill’ to maintain system stability, manage resources effectively, and ensure smooth operation of their processes.