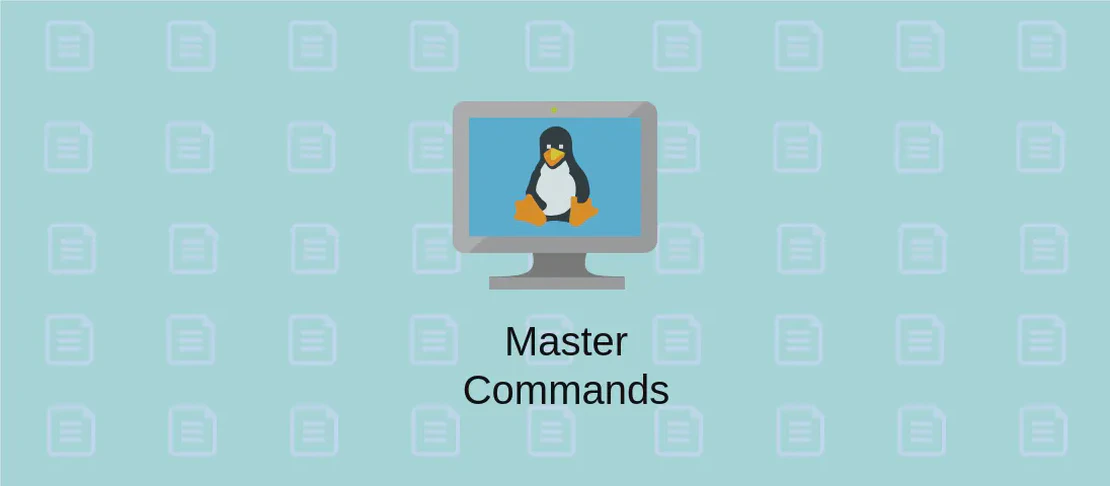
How to use the command 'kotlinc' (with examples)
Kotlin is a statically typed programming language that runs on the Java Virtual Machine (JVM) and can be used to develop various types of applications. The ‘kotlinc’ command is the Kotlin compiler that is used to compile Kotlin source code into JVM bytecode or execute Kotlin scripts. It provides various use cases for compiling Kotlin files, executing scripts, and starting an interactive shell.
Use case 1: Start a REPL (interactive shell)
Code:
kotlinc
Motivation: Starting a REPL allows you to experiment and interactively write Kotlin code without the need for a full compilation process. It can be helpful for quickly testing small snippets or experimenting with language features.
Explanation: When you run the ‘kotlinc’ command without providing any arguments, it launches the Kotlin REPL (Read-Evaluate-Print Loop) or interactive shell. This allows you to directly enter Kotlin code and see the output interactively. You can enter multiple statements, run loops, define functions, and evaluate expressions in this shell.
Example output:
Welcome to Kotlin version 1.6.10 (JRE 1.8.0_312-b07)
Type :help for help, :quit for quit
>> val name = "John"
>> println("Hello, $name!")
Hello, John!
Use case 2: Compile a Kotlin file
Code:
kotlinc path/to/file.kt
Motivation: Compiling Kotlin files is necessary when you want to convert Kotlin source code into JVM bytecode that can be executed directly by the JVM. This use case is commonly used when developing larger applications or libraries.
Explanation: In this use case, you need to provide the path to the Kotlin file that you want to compile as an argument to the ‘kotlinc’ command. The command takes the file path and compiles the Kotlin code into bytecode, generating a corresponding ‘.class’ file.
Example output:
No output is displayed if the compilation is successful. However, a '.class' file will be generated in the same directory as the source file.
Use case 3: Compile several Kotlin files
Code:
kotlinc path/to/file1.kt path/to/file2.kt ...
Motivation: When developing larger applications or projects, it is common to have multiple Kotlin files that depend on each other. In such cases, it is necessary to compile all the Kotlin files together to ensure that all dependencies are resolved correctly during the compilation process.
Explanation: This use case is similar to the previous one, but instead of providing the path to a single Kotlin file, you provide the paths to multiple Kotlin files as arguments to the ‘kotlinc’ command. The command then compiles all the files together, resolving dependencies and generating the corresponding ‘.class’ files.
Example output:
No output is displayed if the compilation is successful. However, the corresponding '.class' files will be generated for each of the input Kotlin files.
Use case 4: Execute a specific Kotlin Script file
Code:
kotlinc -script path/to/file.kts
Motivation: Kotlin allows you to write scripts that can be directly executed. These scripts can be used for automation, quick one-off tasks, or small utilities. This use case is helpful when you want to run a specific Kotlin script file.
Explanation: To execute a Kotlin script file, you need to provide the ‘-script’ flag followed by the path to the Kotlin script file as an argument to the ‘kotlinc’ command. The command executes the script file, running the Kotlin code and producing the output, if any.
Example output:
Output produced by the executed Kotlin script will be displayed in the console.
Use case 5: Compile a Kotlin file into a self-contained jar file with the Kotlin runtime library included
Code:
kotlinc path/to/file.kt -include-runtime -d path/to/file.jar
Motivation: In some scenarios, you might want to distribute your Kotlin code as a standalone jar file that includes the Kotlin runtime library. This allows the jar file to be executed independently, without the need for separately providing the Kotlin runtime library.
Explanation: To compile a Kotlin file into a self-contained jar file, you need to provide the ‘-include-runtime’ flag followed by the path to the Kotlin source file and the ‘-d’ flag followed by the path where you want to save the jar file. The ‘kotlinc’ command then compiles the Kotlin code, includes the Kotlin runtime library, and produces a jar file with the specified name and location.
Example output:
No output is displayed if the compilation is successful. However, a self-contained jar file will be generated at the specified location, which can be executed independently.
Conclusion:
The ‘kotlinc’ command is a versatile tool that provides various use cases for working with Kotlin source code. Whether you need to start an interactive shell, compile individual files or multiple files together, execute Kotlin scripts, or create self-contained jar files, the ‘kotlinc’ command has you covered. These examples demonstrate the flexibility of the command and its ability to handle different scenarios when working with Kotlin. So go ahead, start exploring Kotlin and make the most of the ‘kotlinc’ command!