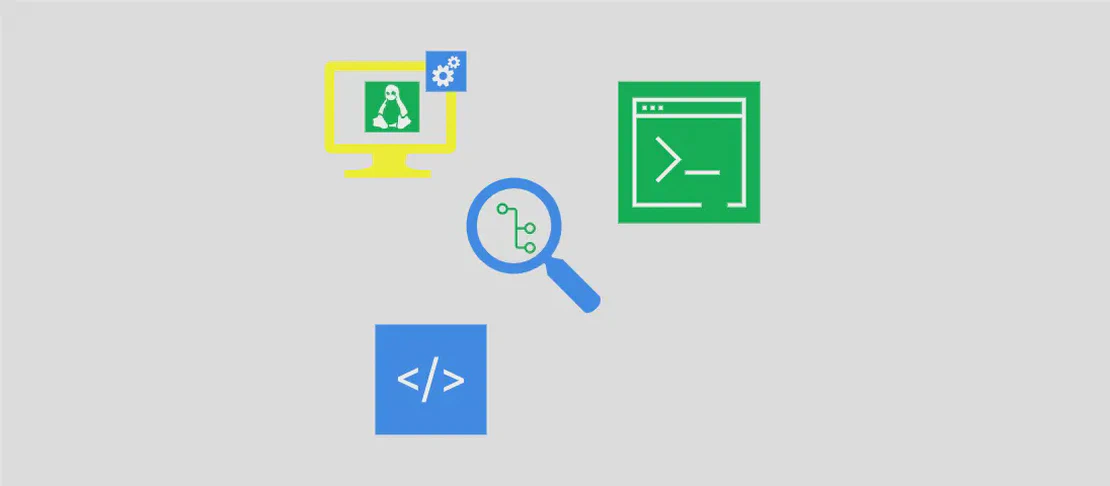
How to use the command 'ksh' (with examples)
Korn Shell (ksh) is a powerful command-line interpreter, compatible with Bash, that provides users with interactive command execution capabilities and script execution proficiency. It is designed for both interactive use and scripting, offering enhanced features and higher performance for everyday shell tasks. This article will explore several practical use cases for exploiting the capabilities of ksh, each paired with practical examples and a step-by-step breakdown.
Use case 1: Start an interactive shell session
Code:
ksh
Motivation:
Starting an interactive shell session using ksh is essential for users who want to engage directly with the shell environment. This provides the flexibility to execute commands one at a time and view their outputs immediately, making it ideal for learning, troubleshooting, and everyday tasks. It’s especially beneficial for testing environmental configurations or running commands that interact with files and processes.
Explanation:
When you enter ksh
in the terminal, it invokes the Korn Shell in interactive mode. This means you are entering a new environment where you can run various shell commands interactively. No additional arguments are needed to start this session.
Example output:
Upon execution, there might not be any visible output immediately. The user will simply find themselves in a new shell prompt (commonly denoted by $
or another character), ready to accept input.
$
Use case 2: Execute specific commands
Code:
ksh -c "echo 'ksh is executed'"
Motivation:
Executing specific commands directly from the command line using ksh can be beneficial for scripts or automation tasks where one-time command execution is required. This option is useful when you want to test a command inline or execute critical operations without launching a full interactive shell session.
Explanation:
-c
: The-c
flag tells ksh to take the next argument as a command string, which should be executed in the shell."echo 'ksh is executed'"
: This is the command you wish to run.echo
is a simple command used to print a string of text to the console or standard output.
Example output:
ksh is executed
Use case 3: Execute a specific script
Code:
ksh path/to/script.ksh
Motivation:
Running scripts through ksh is a common practice for automating tasks and managing system operations through batch processing. This use case allows for executing pre-written scripts, making it easy to perform complex series of operations repeatedly without manual intervention.
Explanation:
path/to/script.ksh
: This part of the command specifies the path to your script file. It becomes executed in the Korn Shell environment when entered. Make sure the script has executable permissions before running it.
Example output:
While the output depends entirely on what operations the script performs, you may expect console messages, computed values, or system changes per the script’s logic.
Script execution results...
Use case 4: Check a specific script for syntax errors without executing it
Code:
ksh -n path/to/script.ksh
Motivation:
This use case is particularly useful for developers and system administrators who prefer to catch syntax errors in a script before running it. Syntax verification helps in ensuring the code is error-free and adheres to structural rules, which is critical to avoiding runtime errors or unexpected results.
Explanation:
-n
: The-n
option tells ksh to read the commands in a script but not execute them. This way, it checks for syntax errors without implementing the functional logic of the script.path/to/script.ksh
: Refers to the script file path you want to check for syntax issues.
Example output:
If there are no syntax errors, there will be no output, indicating the script passed the check. If there are syntax errors, ksh will display error messages detailing the issues found.
(no output indicates the script is syntax error-free)
Use case 5: Execute a specific script, printing each command in the script before executing it
Code:
ksh -x path/to/script.ksh
Motivation:
This debugging technique helps users understand the execution flow of a script by displaying each command as it gets executed. It’s extremely valuable when trying to diagnose issues within scripts or verify command execution order and dependencies.
Explanation:
-x
: The-x
flag enables debugging mode, which prints each command to standard output as it is executed. This visualization aids in better understanding script behavior and pinpointing errors or inefficiencies.path/to/script.ksh
: This specifies the path to the script you want to debug by observing its command execution.
Example output:
The execution output will display each command in the script line-by-line, followed by its result.
+ echo 'Executing the first command'
Executing the first command
+ var=42
+ echo 'The variable value is' 42
The variable value is 42
...
Conclusion:
Each use case highlights a unique aspect of Korn Shell’s rich functionality in handling command execution and script management. Whether starting an interactive session or running scripts with increased visibility through debugging modes, ksh provides tools and features essential for effective system and application management. These capabilities make ksh a valuable resource for both beginner and advanced users in scripting and shell interactions.