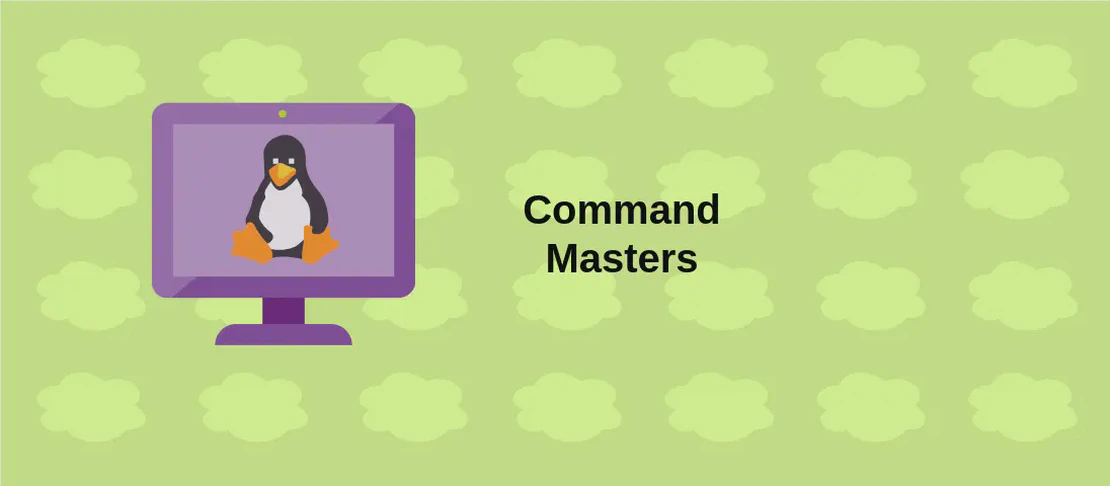
Exploring the Command 'kubectl' (with examples)
kubectl
is a command-line interface tool designed for interacting with Kubernetes clusters. It allows users to perform various operations by communicating with the Kubernetes API server and manage applications, deploy resources, and view cluster data with ease. kubectl
plays a crucial role in the management and orchestration of containerized applications and is an essential tool for developers, operators, and admins working with Kubernetes.
Use Case 1: Listing Information About a Resource with More Details
Code:
kubectl get pod|service|deployment|ingress|... -o wide
Motivation:
Understanding the intricacies of your resources within a Kubernetes cluster is essential for management and troubleshooting. By using the -o wide
option, you can obtain detailed information that goes beyond the default output. This typically includes node hostnames and other useful information that aids in pinpointing resource distribution and status across nodes.
Explanation:
kubectl get
: Fetches and displays information about the specified resource type.pod|service|deployment|ingress|...
: Specifies the type of resource to query. This can be any resource kind managed by Kubernetes.-o wide
: Requests more comprehensive details, usually including additional columns related to IP addresses, node names, and more.
Example Output:
NAME READY STATUS RESTARTS AGE IP NODE
my-pod 1/1 Running 0 3d 10.1.0.15 node1
my-service 10.1.0.1 <none> 443:31519/TCP 3d
Use Case 2: Updating a Pod’s Label
Code:
kubectl label pods name unhealthy=true
Motivation:
Labeling resources in Kubernetes is vital for organizing, selecting, and monitoring purposes. By altering or adding a label to a pod, you can efficiently categorize pods for better management and automating tasks. This is particularly useful for flagging pods that need attention or for creating sets of resources with common traits.
Explanation:
kubectl label
: Modifies or adds labels to existing resources.pods
: Specifies the resource type ‘pods’.name
: The specific pod to be targeted by the label command.unhealthy=true
: Adds or updates the label on the specified pod to indicate that it is unhealthy.
Example Output:
pod/name labeled
Use Case 3: Listing All Resources with Different Types
Code:
kubectl get all
Motivation:
Obtaining a broad overview of your cluster’s resources is critical for assessments and audits. The kubectl get all
command consolidates the list of various resource types, such as pods, services, deployments, etc., allowing you to understand the overall state and operational load on your cluster quickly.
Explanation:
kubectl get all
: This command requests a list of all kinds of resource types currently deployed within the namespace.
Example Output:
NAME READY STATUS RESTARTS AGE
pod/my-app-12345 1/1 Running 0 4d
service/my-service ClusterIP 10.1.95.1 <none> 8080/TCP 4d
Use Case 4: Displaying Resource Usage
Code:
kubectl top pod|node
Motivation:
Monitoring the resource usage of your cluster’s nodes and pods is essential for performance tuning and capacity planning. By using the kubectl top
command, users can obtain real-time data on CPU and memory consumption, helping identify bottlenecks and optimize resources efficiently.
Explanation:
kubectl top
: Fetches resource metrics.pod|node
: Indicates whether to show resource usage for nodes or pods.
Example Output:
NAME CPU(cores) MEMORY(bytes)
my-node 130m 450Mi
Use Case 5: Printing the Cluster Info
Code:
kubectl cluster-info
Motivation:
Access to vital cluster-level information, such as addresses of master and services is crucial for configuring network access, setting up monitoring tools, and connecting APIs. This command quickly surfaces that information, allowing administrators to orchestrate and troubleshoot clusters effectively.
Explanation:
kubectl cluster-info
: A command that retrieves basic cluster details including service endpoints like Kubernetes control plane, DNS, and other service-specific URLs.
Example Output:
Kubernetes control plane is running at https://192.168.99.100:8443
KubeDNS is running at https://192.168.99.100:8443/api/v1/namespaces/kube-system/services/kube-dns:dns/proxy
Use Case 6: Explaining a Specific Field
Code:
kubectl explain pods.spec.containers
Motivation:
Understanding the definitions and schema of specific fields in Kubernetes resources is fundamental for proper configuration and usage. Using kubectl explain
, developers and administrators gain insights into what fields represent and are expected without needing to navigate extensive documentation.
Explanation:
kubectl explain
: Describes resource types and fields for insightful data.pods.spec.containers
: Indicates the specific field within a pod spec to be explained.
Example Output:
KIND: Pod
VERSION: v1
FIELD: containers
DESCRIPTION:
List of containers belonging to the pod.
Use Case 7: Printing Logs for a Pod’s Container
Code:
kubectl logs pod_name
Motivation:
Logs are critical for debugging, monitoring, and auditing system activities within Kubernetes clusters. The kubectl logs
command easily provides access to the logs for containers within pods, aiding users in troubleshooting issues by examining historical data output by applications and processes running within those containers.
Explanation:
kubectl logs
: Retrieves logs from containers.pod_name
: Specifies the pod whose container log is to be extracted.
Example Output:
2023-06-01T10:21:32Z Service started
2023-06-01T10:22:45Z Received incoming request
Use Case 8: Running a Command in an Existing Pod
Code:
kubectl exec pod_name -- ls /
Motivation:
Being able to run a command directly within a pod is useful for debugging, exploration, and direct management. It allows you to inspect container filesystems, execute scripts, or interact with processes directly from the command line.
Explanation:
kubectl exec
: Runs a command within a container.pod_name
: The specific pod in which to execute the command.--
: Separateskubectl
options and pod name from arguments that the user wants to pass to the command.ls /
: The command to list directory contents of the root directory within the pod.
Example Output:
bin
boot
dev
etc
Conclusion:
kubectl
provides an extensive and powerful toolkit for managing Kubernetes clusters. The use cases outlined illustrate a range of functionalities from basic resource listing to intricate resource management and debugging. As users grow more acquainted with each command’s syntax and options, it becomes increasingly apparent that kubectl
is indispensable for efficient Kubernetes operations. Its flexibility and depth empower users to maintain, customize, and optimize the deployment and operation of their clusters.