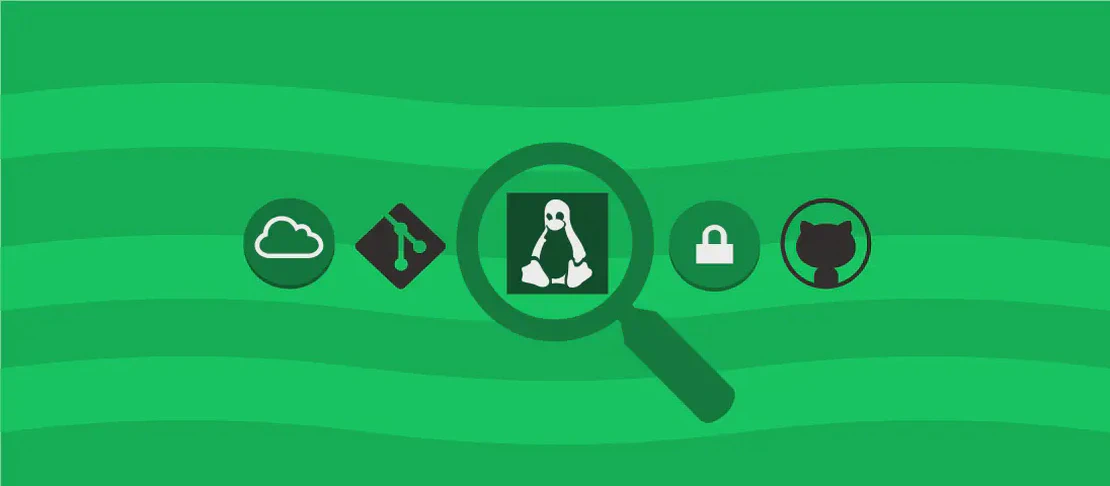
How to use the command 'kubectl create' (with examples)
The kubectl create
command is used in Kubernetes to create a new resource. It can create a resource from a file or from stdin
. This command is commonly used for creating deployments, services, namespaces, and other resources in a Kubernetes cluster.
Use case 1: Create a resource using the resource definition file
Code:
kubectl create -f path/to/file.yml
Motivation: Using a resource definition file allows you to declaratively define the desired state of your resource (such as a deployment or service) and use it to create the resource. This can be useful for maintaining a version-controlled configuration and automating resource creation.
Explanation:
kubectl create
: The base command for creating a resource.-f path/to/file.yml
: Specifies the file path to the resource definition file.
Example output:
deployment.apps/my-deployment created
Use case 2: Create a resource from stdin
Code:
kubectl create -f -
Motivation: This use case allows you to create a resource by providing its definition through stdin
. This can be useful when you want to dynamically generate the resource definition or when the resource definition is not stored in a file.
Explanation:
kubectl create
: The base command for creating a resource.-f -
: Specifies that the resource definition will be provided throughstdin
.
Example output:
deployment.apps/my-deployment created
Use case 3: Create a deployment
Code:
kubectl create deployment deployment_name --image=image
Motivation: Deployments are commonly used in Kubernetes for managing the rollout of containerized applications. This use case allows you to create a deployment with a specified name and container image.
Explanation:
kubectl create
: The base command for creating a resource.deployment deployment_name
: Specifies the resource type (deployment) and the name of the deployment.--image=image
: Specifies the container image to use for the deployment.
Example output:
deployment.apps/my-deployment created
Use case 4: Create a deployment with replicas
Code:
kubectl create deployment deployment_name --image=image --replicas=number_of_replicas
Motivation: Specifying the number of replicas allows you to control the scalability of your application. This use case allows you to create a deployment with a specified number of replicas.
Explanation:
kubectl create
: The base command for creating a resource.deployment deployment_name
: Specifies the resource type (deployment) and the name of the deployment.--image=image
: Specifies the container image to use for the deployment.--replicas=number_of_replicas
: Specifies the number of replicas to create for the deployment.
Example output:
deployment.apps/my-deployment created
Use case 5: Create a service
Code:
kubectl create service service_type service_name --tcp=port:target_port
Motivation: Services in Kubernetes are used to expose applications running in the cluster to other services within or outside the cluster. This use case allows you to create a service with a specified type, name, and port mapping.
Explanation:
kubectl create
: The base command for creating a resource.service service_type service_name
: Specifies the resource type (service), the type of the service (such as ClusterIP or NodePort), and the name of the service.--tcp=port:target_port
: Specifies the mapping between the port exposed by the service and the target port of the application.
Example output:
service/my-service created
Use case 6: Create a namespace
Code:
kubectl create namespace namespace_name
Motivation: Namespaces are used to logically divide a Kubernetes cluster into multiple virtual clusters. This use case allows you to create a namespace with a specified name.
Explanation:
kubectl create
: The base command for creating a resource.namespace namespace_name
: Specifies the resource type (namespace) and the name of the namespace.
Example output:
namespace/my-namespace created
Conclusion:
The kubectl create
command is a versatile command in Kubernetes that allows you to create various resources. Whether you want to create a deployment, service, namespace, or any other resource, this command provides the flexibility to define the desired state and create the resource in your cluster.