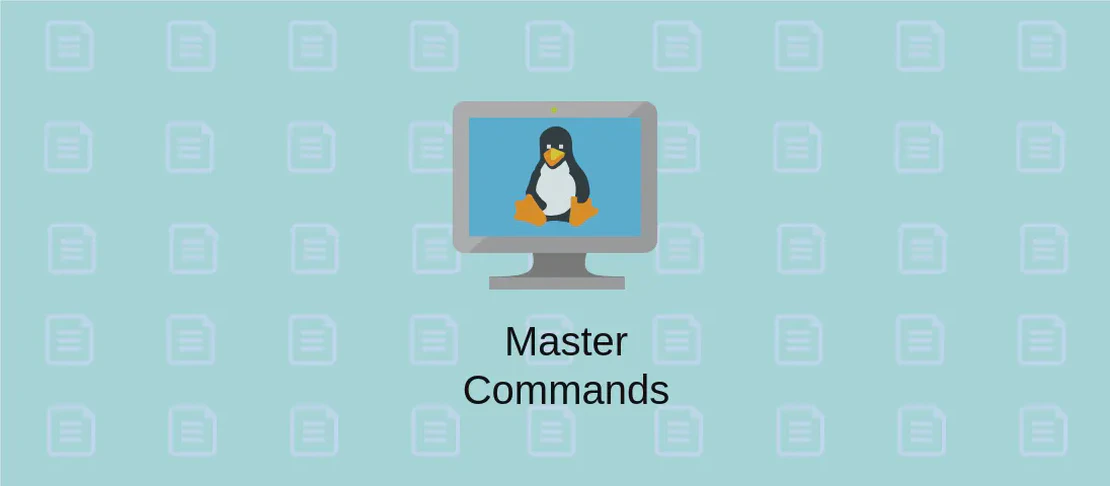
How to use the command 'kubectl delete' (with examples)
The kubectl delete
command is a powerful tool used in Kubernetes to manage and delete resources within a cluster. It allows administrators and developers to remove specific resources, entire collections of resources, or resources defined in configuration files. This command helps in maintaining and managing the cluster’s state by cleaning up resources that are no longer needed, freeing up resources, and ensuring that the cluster does not have extraneous services or pods running. The kubectl delete
command can target individual resources or groups, making it flexible for various operational needs.
Use case 1: Delete a specific pod
Code:
kubectl delete pod pod_name
Motivation:
Deleting individual pods is a common task when you need to remove faulty instances, test new configurations, or simply free up system resources. By removing a specific pod, you can troubleshoot issues related to that instance or prepare for deploying new versions of your applications.
Explanation:
kubectl
: The command-line tool used to interact with Kubernetes clusters.delete
: Indicates the action that needs to be performed, in this case, deletion.pod
: Specifies the type of resource you want to delete.pod_name
: The specific name of the pod you wish to delete.
Example output:
pod "pod_name" deleted
Use case 2: Delete a specific deployment
Code:
kubectl delete deployment deployment_name
Motivation:
Deployments are used to manage a set of identical pods. If you need to remove or update the deployment due to updates in application code or configurations, deleting it allows you to stop its existing instances effectively. This command is crucial when transitioning your application to newer versions or cleaning up outdated deployments.
Explanation:
kubectl
: The Kubernetes command-line tool.delete
: The operation to be executed.deployment
: Identifies the type of resource to be deleted.deployment_name
: The exact name of the deployment that is targeted for deletion.
Example output:
deployment.apps "deployment_name" deleted
Use case 3: Delete a specific node
Code:
kubectl delete node node_name
Motivation:
Removing nodes from a cluster can be necessary during scaling down of resources or when a node is malfunctioning. Deleting a node ensures that workloads are redistributed, preventing resource wastage on faulty or underutilized machines.
Explanation:
kubectl
: The tool to manage Kubernetes clusters.delete
: Specifies the action of removal.node
: Indicates that a node is the target for deletion.node_name
: The specific node you want to remove from the cluster.
Example output:
node "node_name" deleted
Use case 4: Delete all pods in a specified namespace
Code:
kubectl delete pods --all --namespace namespace
Motivation:
Clearing all pods in a namespace is useful when you want to reset the environment or clean up after tests. It helps in managing the lifecycle of pods and ensuring that a namespace is only running the necessary pods.
Explanation:
kubectl
: The Kubernetes command tool.delete
: Action to remove resources.pods
: Specifies that the resources being deleted are pods.--all
: A flag indicating that all pods should be targeted.--namespace
: Filters the command to only affect pods within the specified namespace.namespace
: The name of the namespace containing the pods you want to delete.
Example output:
pod "pod1" deleted
pod "pod2" deleted
...
Use case 5: Delete all deployments and services in a specified namespace
Code:
kubectl delete deployments,services --all --namespace namespace
Motivation:
Deleting all deployments and services in a namespace simplifies the cleanup process, which is especially useful during development or testing phases. It ensures all relevant resources are terminated without manually specifying each one, helping maintain an organized cluster state.
Explanation:
kubectl
: Refers to the command-line tool for Kubernetes.delete
: Denotes the operation for deletion.deployments,services
: Specifies the types of resources to be deleted, separated by a comma.--all
: Indicates that every resource in the specified types should be included.--namespace
: Limits the scope to a particular namespace.namespace
: The specific namespace from which you’re removing resources.
Example output:
deployment.apps "deployment1" deleted
deployment.apps "deployment2" deleted
service "service1" deleted
service "service2" deleted
Use case 6: Delete all nodes
Code:
kubectl delete nodes --all
Motivation:
In scenarios that require a complete teardown of a cluster or when starting fresh, deleting all nodes is a comprehensive way to ensure that no workloads are left running. It’s crucial when changing infrastructure or fully redeploying the cluster environment.
Explanation:
kubectl
: The utility for Kubernetes operations.delete
: The action to remove resources.nodes
: Specifies nodes as the target resource type.--all
: Directs the command to act on all nodes within the cluster.
Example output:
node "node1" deleted
node "node2" deleted
...
Use case 7: Delete resources defined in a YAML manifest
Code:
kubectl delete --filename path/to/manifest.yaml
Motivation:
This command is extremely useful for managing resources using configuration as code. When configurations need to be updated, deleted, or reapplied, using a YAML manifest allows for a reproducible and consistent approach to resource management.
Explanation:
kubectl
: The command-line interface for Kubernetes.delete
: Specifies the deletion operation.--filename
: Indicates that the resources to be deleted are specified in a file.path/to/manifest.yaml
: The path to the YAML manifest file that defines the resources for deletion.
Example output:
service "service-name" deleted
deployment.apps "deployment-name" deleted
Conclusion:
The kubectl delete
command provides a robust toolset for managing resources within a Kubernetes cluster. Its range of options allows for targeted actions on specific resources, as well as broader deletions across namespaces and types. By understanding and utilizing these commands effectively, administrators and developers can maintain an efficient and orderly cluster environment.