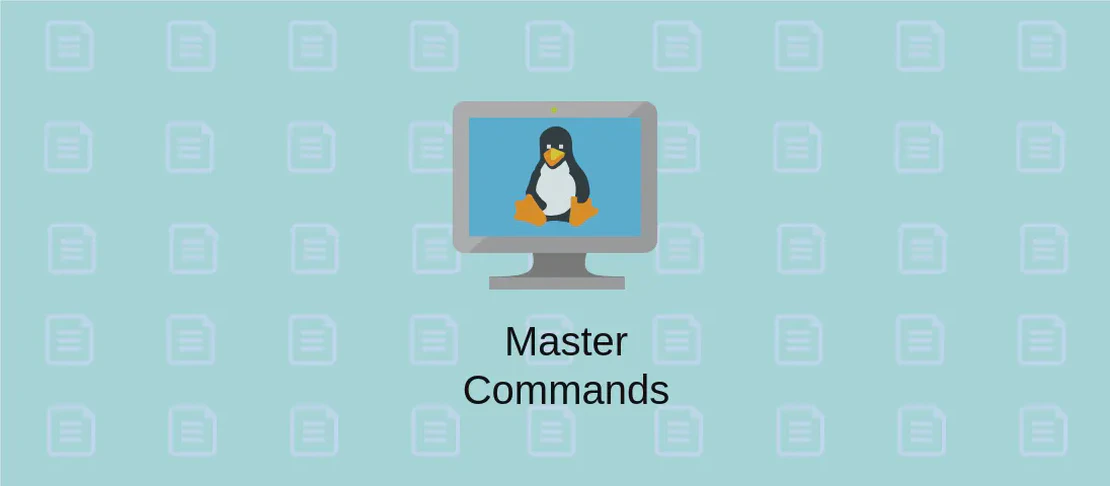
Utilizing 'kubectl describe' for Effective Kubernetes Management (with examples)
The kubectl describe
command is an essential tool within the Kubernetes ecosystem that provides detailed insights into Kubernetes objects and resources. Whether you’re managing a cluster or debugging issues, understanding the intricacies of your resources is crucial, and kubectl describe
is often the go-to command for retrieving comprehensive information. Its ability to dissect and present a wide array of details makes it invaluable for developers, system administrators, and DevOps engineers who need to manage Kubernetes clusters efficiently.
Use case 1: Show details of pods in a namespace
Code:
kubectl describe pods --namespace example-namespace
Motivation: In Kubernetes, pods represent a group of one or more containers. Understanding the status, events, and configuration of your pods is crucial to monitor their performance and health. Often, in a large cluster, resources are organized in different namespaces, and having the ability to view pod details specific to a namespace allows for targeted monitoring and debugging.
Explanation:
describe
: This command fetches detailed information about a resource.pods
: Specifies that the command should return details about pod entities.--namespace example-namespace
: Limits the scope of the operation to pods within the specified namespace, making it easier to focus on a specific subset of your cluster.
Example Output:
Name: my-pod
Namespace: example-namespace
Node: node-1/192.168.1.1
Start Time: Fri, 22 Oct 2023 14:30:00 +0000
Labels: app=my-app
Annotations: <none>
Status: Running
IP: 192.168.1.10
Containers:
my-container:
Container ID: containerd://123abc
Image: my-image:latest
Image ID: docker-pullable://my-image@sha256:abcdef123456
Port: 80/TCP
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal Scheduled 2m default-scheduler Successfully assigned my-pod to node-1
Normal Pulled 2m kubelet, node-1 Container image "my-image:latest" already present on machine
Normal Created 2m kubelet, node-1 Created container my-container
Use case 2: Show details of nodes in a namespace
Code:
kubectl describe nodes --namespace example-namespace
Motivation: Nodes are the virtual or physical machines in a Kubernetes cluster, and they host your pods. Analyzing node details such as resource availability, capacity, and usage can give you a better understanding of the cluster’s health and scalability. When troubleshooting issues like pod scheduling failures, reviewing node information within specific namespaces can provide insights into resource bottlenecks or configuration problems.
Explanation:
describe
: Requests detailed information on the resource.nodes
: Highlights that node-specific details will be presented.--namespace example-namespace
: Although nodes are cluster-wide resources, this flag would be used more hypothetically since nodes are not namespaced. However, it could help script comprehensive resource-checks where pods and node details are correlated.
Example Output:
Name: node-1
Roles: worker
Labels: beta.kubernetes.io/arch=amd64
beta.kubernetes.io/instance-type=m4.large
CPU Requests: 1 (2%)
Memory Requests: 1.1 Gi (5%)
PodCIDR: 192.168.1.0/24
PodCIDRs: 192.168.1.0/24
Allocatable:
cpu: 8
memory: 32Gi
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal NodeHasSufficientMemory 43m kubelet, node-1 Node node-1 status is now: NodeHasSufficientMemory
Normal NodeHasSufficientDisk 43m kubelet, node-1 Node node-1 status is now: NodeHasSufficientDisk
Use case 3: Show the details of a specific pod in a namespace
Code:
kubectl describe pods specific-pod --namespace example-namespace
Motivation: Knowing the exact details of a specific pod is critical when diagnosing issues or when you need to verify configurations after deployment. It helps in identifying pod-specific problems such as incorrect configurations, image pull errors, or resource limitations. This command focuses on retrieving detailed information for one particular pod within a defined namespace.
Explanation:
describe pods
: The command targets pods, requesting detailed information.specific-pod
: The name of the specific pod you wish to describe.--namespace example-namespace
: Ensures the command operates within the designated namespace, focusing solely on what you are interested in.
Example Output:
Name: specific-pod
Namespace: example-namespace
Priority: 0
Node: node-2/192.168.1.2
Containers:
my-app-container:
Container ID: docker://abcd1234
Image: my-application:v1.0
Image ID: docker-pullable://my-application@sha256:abcd1234
State: Running
Started: Sat, 23 Oct 2023 22:30:00 +0000
Ready: true
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal Pulled 10m kubelet, node-2 Successfully pulled image "my-application:v1.0"
Normal Created 10m kubelet, node-2 Created container my-app-container
Use case 4: Show the details of a specific node in a namespace
Code:
kubectl describe nodes specific-node --namespace example-namespace
Motivation: When managing Kubernetes, individual nodes might exhibit problems like high resource usage or node taints that could affect pod scheduling. This command is crucial for investigating node-specific configurations, status, and events that might help in troubleshooting node-related issues.
Explanation:
describe nodes
: Indicates that detailed information about the nodes is being requested.specific-node
: Identifies the individual node to describe.--namespace example-namespace
: Even though nodes are not constrained by namespaces, this parameter can maintain consistency within scripts or specifically formatted inputs where integration with namespaces occurs.
Example Output:
Name: specific-node
Roles: master
Labels: kubernetes.io/hostname: specific-node
node-role.kubernetes.io/master: ''
Allocatable:
cpu: 16
memory: 64Gi
System Info:
Kernel Version: 5.4.0-1046-aws
OS Image: Ubuntu 20.04.3 LTS
Operating System: linux
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal Starting 2d1h kubelet, specific-node Starting kubelet.
Normal Registered 2d1h kubelet, specific-node Node specific-node registered with master
Use case 5: Show details of Kubernetes objects defined in a YAML manifest file
Code:
kubectl describe --file path/to/manifest.yaml
Motivation: YAML manifests are often used to define the desired state of Kubernetes objects, including services, deployments, and pods. Before applying changes from a manifest, understanding the detailed configuration can prevent potential deployment issues. This command enables users to review the intricate details of the configurations specified in the manifest.
Explanation:
describe
: Fetches a detailed description of the resource configurations indicated within a provided manifest.--file path/to/manifest.yaml
: Specifies the path to the YAML file, which contains the definitions of Kubernetes objects you wish to describe.
Example Output:
Name: my-service
Type: ClusterIP
IP: 10.0.0.1
Port: http 80/TCP
Endpoints:
Events: <none>
---
Name: my-deployment
StrategyType: Recreate
Replicas: 1 desired | 1 updated | 1 total | 1 available | 0 unavailable
Containers:
my-container:
Image: nginx:1.18
Port: <none>
Environment: <none>
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal Scheduled 12m default-scheduler Successfully assigned my-deployment-5cb65d7556-lgh9m
Conclusion:
In conclusion, the kubectl describe
command is indispensable for obtaining a comprehensive understanding of the Kubernetes objects and resources. Through varying scopes of focus—from namespaces to individual nodes, pods, and YAML manifests—it provides essential insights that help in both routine management and troubleshooting specific issues. As clusters grow in complexity, the ability to quickly access detailed information using kubectl describe
becomes increasingly valuable for efficient operations and maintenance.