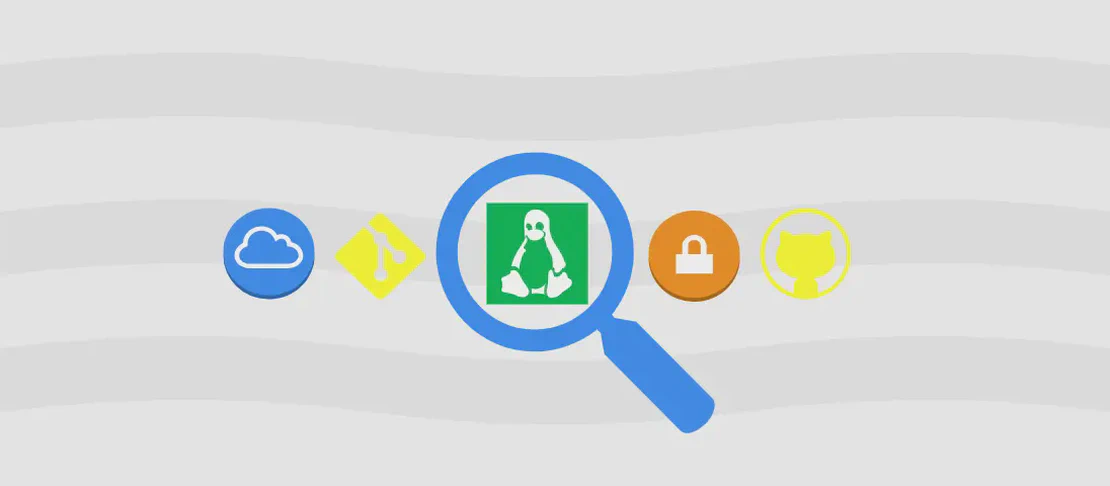
How to use the command 'kubectl logs' (with examples)
In Kubernetes, pods are the smallest deployable units that can be created and managed. Each pod consists of one or more containers running in your cluster. The command kubectl logs
is a powerful tool that allows you to retrieve and stream the logs of these containers, which is essential for debugging and monitoring applications. By accessing the logs, developers and operators can gain insights into the performance and behavior of their applications. The following examples illustrate various use cases of the kubectl logs
command.
Use case 1: Show logs for a single-container pod
Code:
kubectl logs pod_name
Motivation: Fetching logs from a single-container pod is perhaps the most straightforward use case. This is particularly useful for situations where you need to quickly check the output of an application, investigate an issue, or monitor interaction logs without the complexity introduced by multiple containers within a pod.
Explanation:
kubectl logs
: This command is invoking the Kubernetes command-line tool to access logs.pod_name
: Replace this placeholder with the actual name of the pod whose logs you want to view. In a single-container pod, specifying the pod name is sufficient to fetch the logs of the container it encapsulates.
Example output:
2023-10-01T12:00:00.000Z This is a log entry from the application within the pod.
2023-10-01T12:01:00.000Z Another relevant log entry...
Use case 2: Show logs for a specified container in a pod
Code:
kubectl logs --container container_name pod_name
Motivation:
When working with multi-container pods, it becomes crucial to isolate the log output of individual containers for effective debugging and monitoring. Specifying the container in the kubectl logs
command helps in focusing only on the logs of interest, such as logs pertaining to a specific microservice within a pod.
Explanation:
--container container_name
: This flag specifies the exact container you want to retrieve logs from within a multi-container pod. Replacecontainer_name
with the desired container’s name.pod_name
: The name of the pod that houses the specified container.
Example output:
2023-10-01T12:05:00.000Z Log entry from the specified container.
2023-10-01T12:06:00.000Z Another log entry specific to the container...
Use case 3: Show logs for all containers in a pod
Code:
kubectl logs --all-containers=true pod_name
Motivation: In scenarios where you need to get a holistic view of everything happening inside a pod, it is beneficial to capture logs from all containers within that pod. This can provide a more complete picture, especially if you suspect that issues might arise from the interaction between multiple containers.
Explanation:
--all-containers=true
: By setting this flag to true, the command fetches logs from every container in the specified pod.pod_name
: The specific pod from which you wish to retrieve all container logs.
Example output:
[container1] 2023-10-01T12:10:00.000Z Log entry from container 1.
[container2] 2023-10-01T12:10:01.000Z Log entry from container 2.
Use case 4: Stream pod logs
Code:
kubectl logs --follow pod_name
Motivation: When actively debugging or monitoring a pod, it is advantageous to stream logs in real time. This continuous feed enables developers to see logs as they are generated, facilitating immediate insight into the application’s runtime behavior and allowing quick responses to issues.
Explanation:
--follow
: This flag streams the logs in real-time so that new log entries are printed to the console as they are made.pod_name
: The name of the pod whose logs you wish to stream live.
Example output:
2023-10-01T12:15:00.000Z Live log entry 1 from the running application.
2023-10-01T12:15:01.000Z Another live log entry appearing in real-time...
Use case 5: Show pod logs newer than a relative time
Code:
kubectl logs --since=5m pod_name
Motivation:
Sometimes, the logs of interest are only those that have been generated recently, for instance, after a recent deployment or a new issue arising. By using the --since
flag, you can filter logs to only those that are newer than a specified relative time period.
Explanation:
--since=5m
: This argument tellskubectl logs
to only return logs that are newer than the specified relative time, in this case, 5 minutes.pod_name
: The name of the pod from which newer logs are needed.
Example output:
2023-10-01T12:20:00.000Z Recent log entry 1.
2023-10-01T12:21:00.000Z Another recent log entry within the last 5 minutes...
Use case 6: Show the 10 most recent logs in a pod
Code:
kubectl logs --tail=10 pod_name
Motivation: In the interest of brevity and relevance, sometimes it’s sufficient to view just the last few log entries. This is particularly the case when checking for errors that are causing a pod to crash, or when you wish to see the most recent transactions processed by the application.
Explanation:
--tail=10
: This flag restricts the output to only the last 10 log entries.pod_name
: The targeted pod from which you want to view recent logs.
Example output:
2023-10-01T12:25:00.000Z Log entry -10 from the tail end.
...
2023-10-01T12:26:00.000Z Most recent log entry.
Use case 7: Show all pod logs for a given deployment
Code:
kubectl logs deployment/deployment_name
Motivation: Viewing logs at the deployment level is beneficial when you have multiple replicas of a pod and need to aggregate their logs. This is helpful when diagnosing issues affecting an entire application or service, rather than individual instances.
Explanation:
deployment/deployment_name
: Here,kubectl logs
is used with the path to target all pods within a deployment. Replacedeployment_name
with the specific deployment identifier.- The command will aggregate logs across all replicas associated with the deployment.
Example output:
[pod1] 2023-10-01T12:30:00.000Z Log entry from pod 1, replica A.
[pod2] 2023-10-01T12:30:01.000Z Log entry from pod 2, replica B.
Conclusion:
The kubectl logs
command is an essential tool for Kubernetes administrators and developers, providing a flexible way to access application logs for debugging, monitoring, and auditing purposes. Whether you’re working with single or multi-container pods, need real-time updates, or want to focus on particular time-bound log entries, kubectl logs
offers multiple options to cater to various use cases.