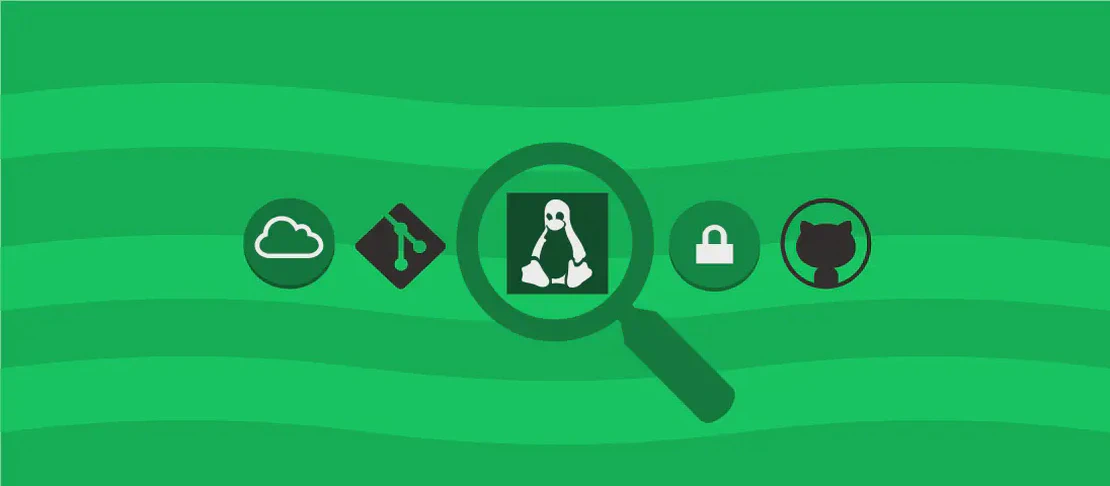
How to Use the Command 'kubectl run' (with Examples)
The kubectl run
command in Kubernetes provides a straightforward mechanism to launch pods, and it offers flexibility through its variety of options and parameters. Although newer Kubernetes versions have introduced different tools and methods, kubectl run
remains a valuable command due to its simplicity and ease of use for quickly creating containers when testing, developing, or deploying applications. Below are some common use cases, each illustrating different functionalities you can achieve with kubectl run
.
Use Case 1: Running an Nginx Pod and Exposing Port 80
Code:
kubectl run nginx-dev --image=nginx --port 80
Motivation:
This example demonstrates a quick way to launch an Nginx pod, establishing a default HTTP server setup. It’s particularly useful in development and testing environments where you need immediate access to a web server to test connectivity, configurations, or changes in a controlled Kubernetes environment.
Explanation:
nginx-dev
: Assigns a name to the pod for easy identification and management.--image=nginx
: Specifies the container image to use, in this case, the latest official Nginx image from the Docker Hub.--port 80
: Indicates that port 80 should be exposed, allowing network traffic to reach the Nginx web server.
Example Output:
pod/nginx-dev created
This output simply confirms the successful creation of an Nginx pod.
Use Case 2: Running an Nginx Pod with an Environment Variable
Code:
kubectl run nginx-dev --image=nginx --env="TEST_VAR=testing"
Motivation:
Setting environment variables at the time of pod creation can affect application behavior, parameterize configurations, or seamlessly integrate with external systems. This is especially useful to achieve reusability and scalability in configurations, adapting to different environments such as testing, staging, or production.
Explanation:
nginx-dev
: Uses a custom pod name for organization and management.--image=nginx
: Indicates the Nginx image from Docker Hub is the basis for the container.--env="TEST_VAR=testing"
: Sets an environment variable namedTEST_VAR
with the valuetesting
, which can be accessed by the application inside the container.
Example Output:
pod/nginx-dev created
The confirmation message that the pod has been successfully launched with the given environment variable.
Use Case 3: Displaying API Calls for Creating an Nginx Container
Code:
kubectl run nginx-dev --image=nginx --dry-run=none|server|client
Motivation:
Understanding the API interactions with Kubernetes is crucial for debugging and enhancing Kubernetes skills. The --dry-run
flag provides insight by simulating the creation of a pod, printing the equivalent API calls that would be made, which is invaluable when learning how Kubernetes constructs resources and interacts internally.
Explanation:
nginx-dev
: Assigns a name to the simulated pod.--image=nginx
: Designates the Nginx image for reference in the mock creation scenario.--dry-run=none|server|client
: Chooses between different dry run modes (none
does not simulate,server
performs a server-side check,client
is local) to show what interactions would occur at the server or client level.
Example Output:
pod "nginx-dev" created (dry run)
This message indicates a simulation of the pod creation, suggesting that the real execution would be successful.
Use Case 4: Running an Interactive Ubuntu Pod Without Restart and Auto-Removal
Code:
kubectl run temp-ubuntu --image=ubuntu:22.04 --restart=Never --rm -- /bin/bash
Motivation:
Running an interactive pod is beneficial for administrators and developers needing an immediate shell within the Kubernetes cluster for debugging or exploring system commands and configurations. The automatic removal ensures clutter is minimized when the task completes.
Explanation:
temp-ubuntu
: Names the pod for identification during its short lifetime.--image=ubuntu:22.04
: Specifies a particular Ubuntu image version to suit compatibility and use-case requirements.--restart=Never
: Instructs Kubernetes not to restart the pod, suitable for single-use and transient tasks.--rm
: Automatically deletes the pod after it exits, preventing namespace pollution and resource waste./bin/bash
: Launches a Bash shell interactively within the container.
Example Output:
If you don't see a command prompt, try pressing enter.
root@temp-ubuntu:/#
This interactive prompt allows users to interact with the Ubuntu environment directly.
Use Case 5: Running an Ubuntu Pod with Custom Command and Arguments
Code:
kubectl run temp-ubuntu --image=ubuntu:22.04 --command -- echo argument1 argument2 ...
Motivation:
There are scenarios where executing a specific command with custom arguments in a new pod proves beneficial, such as task automation, script execution, or utility operations within a cluster environment. It offers versatility in how tasks and applications interact with Kubernetes resources.
Explanation:
temp-ubuntu
: Provides an identifiable name for the pod.--image=ubuntu:22.04
: Uses an Ubuntu image, well-suited for general-purpose tasks.--command
: Directs Kubernetes to interpret the following input as the command to run, rather than the default entry point.echo argument1 argument2 ...
: Replaces the default container start-up command withecho
and its arguments, allowing dynamic output generation.
Example Output:
argument1 argument2 ...
The example output displays the given arguments, as the custom echo
command is executed in the container.
Conclusion:
The versatility of the kubectl run
command extends beyond mere pod creation, serving as a convenient tool for developers and administrators to quickly set up, test, and automate applications within Kubernetes. By combining different arguments and options, kubectl run
can address a variety of needs, from initial application deployments to intricate task execution, fostering a more efficient and manageable Kubernetes environment.