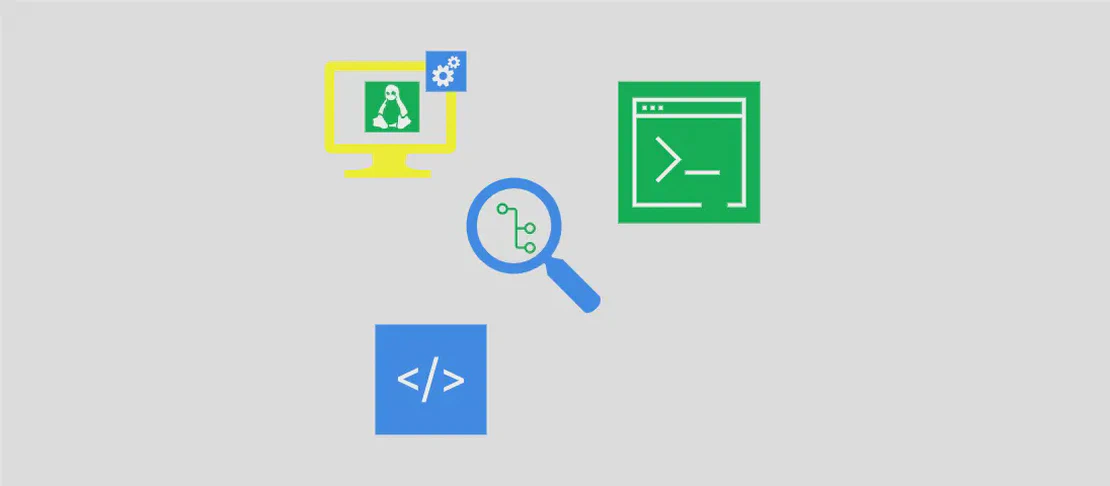
How to use the command 'ld' (with examples)
The ’ld’ command, commonly known as the linker, is a crucial part of the software build process. It takes one or more object files generated by a compiler, like gcc
, and combines them to create an executable program or library. This operation is an essential step in translating code from high-level programming languages into a format that can be understood and executed by a computer’s hardware. The ’ld’ command allows developers to link object files with necessary libraries and specify output executable file options.
Use case 1: Link a specific object file with no dependencies into an executable
Code:
ld path/to/file.o --output path/to/output_executable
Motivation: In software development, especially in the initial phases or when debugging small sections of code, a developer might want to link a simple, standalone object file into an executable. This small-scale linking can be helpful for testing purposes or when the code has no external dependencies. By transforming the object file directly into an executable, developers can quickly identify if a problem lies within the code or if it emerges due to interactions between multiple linked files.
Explanation:
path/to/file.o
: This is the object file that was previously compiled from source code. Object files represent an intermediate step in the build process and contain machine code that hasn’t yet been linked with libraries or other parts of a program.--output path/to/output_executable
: This flag specifies the name and location of the output executable file. The linker will take the object file, resolve any symbols or references within it (if necessary), and produce a final executable file.
Example Output: Executing the command successfully would result in an executable file created at the specified location. If the process fails, an error message will be provided, indicating issues such as missing symbols or incorrect file paths.
Use case 2: Link two object files together
Code:
ld path/to/file1.o path/to/file2.o --output path/to/output_executable
Motivation: As software becomes more complex, it often gets divided into multiple source files, each compiled into its respective object file. Linking two or more object files is crucial for creating cohesive applications where functionalities spread across different modules can interact seamlessly. This is often the case in larger projects where different features or components are developed separately and are combined at the linking stage to produce a full executable.
Explanation:
path/to/file1.o
andpath/to/file2.o
: These are the object files that need to be linked together. Each object file includes machine code representing part of the application, possibly with calls to functions that will be resolved in the other object file or in external libraries.--output path/to/output_executable
: The output option is once again used to specify the result of the linking process — an executable file that incorporates the functionality encoded in both object files.
Example Output: Upon running the command, if both object files are compatible and all dependencies can be resolved, the output will be a single executable file. Any errors during linking, such as unresolved references, will be displayed as error messages.
Use case 3: Dynamically link an x86_64 program to glibc
Code:
ld --output path/to/output_executable --dynamic-linker /lib/ld-linux-x86-64.so.2 /lib/crt1.o /lib/crti.o -lc path/to/file.o /lib/crtn.o
Motivation: Dynamic linking allows programs to use shared libraries at runtime rather than compiling them into the executable. This saves memory and storage space, facilitates easier updates, and can improve application performance. In this specific use case, we are linking an x86_64 architecture program to the GNU C Library (glibc), a standard library in many Unix-like operating systems. The process is crucial for applications that require standard library functionalities.
Explanation:
--output path/to/output_executable
: Specifies the resultant executable from the linking process.--dynamic-linker /lib/ld-linux-x86-64.so.2
: This specifies the dynamic linker to use. The dynamic linkage is crucial for connecting the executable with shared libraries at runtime./lib/crt1.o
,/lib/crti.o
, and/lib/crtn.o
: These files are used as startup routines and represent essential components in the C runtime initialization and termination process. They help set up the process environment and establish the parameters needed for the program to start execution from the main function.-lc
: This indicates that the linker should link against the standard C library,glibc
. This linkage allows the executable to use standard functions likeprintf
ormalloc
.path/to/file.o
: The object file that constitutes the core logic of the executable being built.
Example Output: Successfully executing this command would generate a dynamically linked executable that, when run, utilizes glibc for essential library functions. If path issues or incompatibility arise during the linking phase, the linker will output relevant error messages to help pinpoint the problem.
Conclusion
The ’ld’ command is a powerful tool in the software build process, allowing developers to link object files into cohesive executable applications. By understanding its various use cases and configurations, software engineers can effectively manage dependencies and streamline the development process, from simple single-file applications to complex, multi-file programs that require dynamic linking with essential libraries.