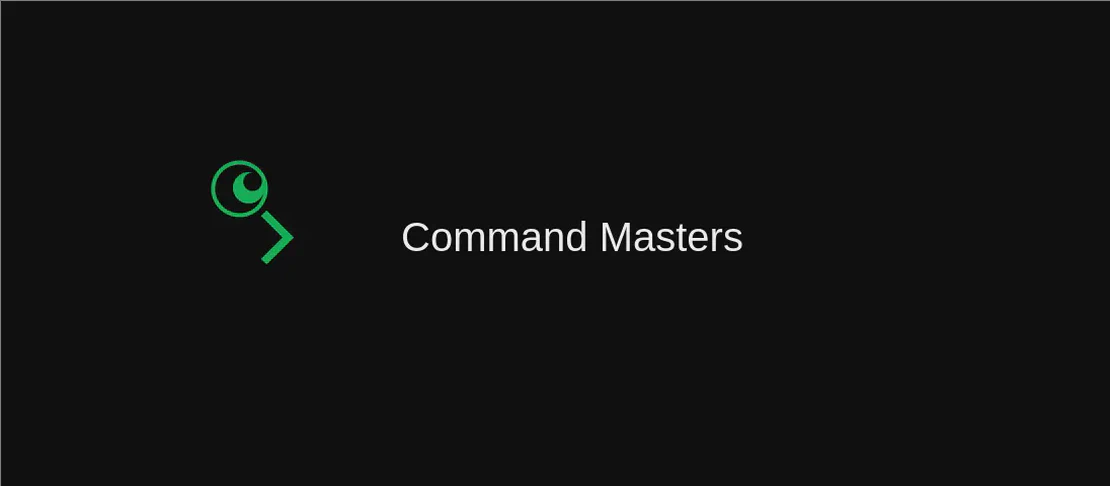
How to use the command ldc (with examples)
The ldc command is a D programming language compiler that uses LLVM as a backend. It allows developers to compile D source code files into executable binaries. This article will illustrate various use cases of the ldc command and provide code examples, motivation for using each example, an explanation of the command arguments, and example outputs.
Use case 1: Compile a source code file into an executable binary
Code:
ldc2 path/to/source.d -of=path/to/output_executable
Motivation: Compiling a source code file into an executable binary is a common task in software development. This use case allows developers to easily compile their D source code into an executable that can be run independently on their target platform.
Explanation:
ldc2
: The ldc2 command invokes the ldc compiler.path/to/source.d
: Specifies the path to the source code file that needs to be compiled.-of=path/to/output_executable
: Specifies the output file path and name for the generated executable.
Example output: If the compilation is successful, the command will generate an executable binary file at the specified output path.
Use case 2: Compile the source code file without linking
Code:
ldc2 -c path/to/source.d
Motivation: In some cases, it may be useful to compile the source code file without linking it to generate an object file. This allows developers to use the generated object file in subsequent compilation steps or link it with other object files later.
Explanation:
ldc2
: Same as in use case 1.-c
: This option tells ldc2 to compile the source code file without linking it.
Example output: If the compilation is successful, the command will generate an object file (.o file) at the same location as the source code file.
Use case 3: Select the target architecture and OS
Code:
ldc -mtriple=architecture_OS -c path/to/source.d
Motivation: Sometimes, it is necessary to compile the source code for a specific target architecture and operating system environment. This use case allows developers to specify the desired target architecture and OS, ensuring compatibility and optimized execution on the intended platform.
Explanation:
ldc
: Same as in use cases 1 and 2.-mtriple=architecture_OS
: Specifies the target architecture and OS using the LLVM triple format. For example, x86_64-pc-windows-msvc indicates a 64-bit Windows target.
Example output: If the compilation is successful, the command will generate an object file (.o file) at the same location as the source code file, specific to the selected target architecture and OS.
Use case 4: Display help
Code:
ldc2 -h
Motivation: Having quick access to the ldc command’s help documentation can be immensely useful, especially when developers need to refresh their memory about specific command-line options, flags, or usage instructions.
Explanation:
ldc2
: Same as in use cases 1 and 2.-h
: This flag displays a brief help message with the available command-line options.
Example output: Running the above command will output a brief help message, providing an overview of the available command-line options and their descriptions.
Use case 5: Display complete help
Code:
ldc2 -help-hidden
Motivation: While the previous use case provides a brief help message, this use case allows developers to access the complete and detailed help documentation, including all available options, flags, and their descriptions.
Explanation:
ldc2
: Same as in use cases 1, 2, and 4.-help-hidden
: This flag displays the complete help documentation, including all available command-line options, flags, and their descriptions.
Example output: Running the above command will output the complete help documentation for the ldc command, providing detailed information about all available command-line options, flags, and their descriptions.
Conclusion:
The ldc command is a powerful compiler for the D programming language, utilizing LLVM as a backend. It offers various options and flags to compile and optimize D source code files. By understanding these use cases and their examples, developers can efficiently utilize the ldc command to compile their D programs for specific architectures and operating systems, control the linking process, and access the help documentation when needed.