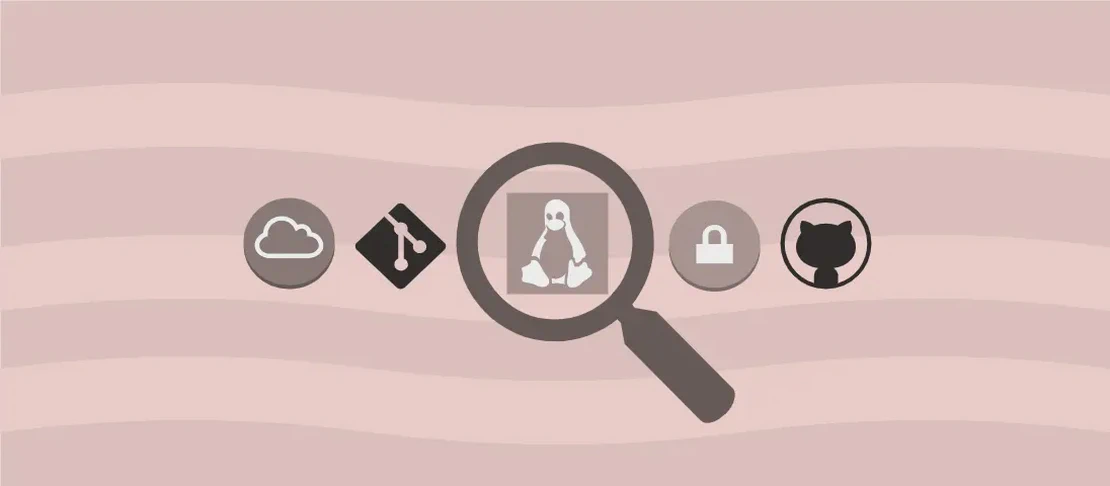
How to Utilize the 'lebab' Command (with examples)
Lebab is a JavaScript tool that transforms older ECMAScript (ES) codebases into more modern ES6/ES7 syntax. This tool is vital for developers striving to update legacy JavaScript code, enhancing readability, performance, and maintainability. By using different transformations offered by Lebab, developers can seamlessly convert functions, variables, and other JavaScript components to utilize the latest JavaScript standards.
Use case 1: Transpile using one or more comma-separated transformations
Code:
lebab --transform arrow,let
Motivation:
The motivation for this use case is to update legacy JavaScript code that uses traditional function expressions and variable declarations with var
. By transforming these to arrow functions and let
declarations, the code becomes more concise and behaves more predictably due to better variable scoping.
Explanation:
--transform
: This flag tells Lebab which transformations to apply.arrow,let
: These are specific transformation options.arrow
converts traditional anonymous functions to arrow functions, andlet
changes variable declarations fromvar
to block-scopedlet
.
Example Output: Given legacy code:
var x = function(a, b) { return a + b; };
After transformation, the code becomes:
let x = (a, b) => a + b;
Use case 2: Transpile a file to stdout
Code:
lebab path/to/input_file
Motivation:
Transpiling to stdout
is useful for quickly examining the output of transformations without altering any files. This aids developers in verifying the transformations and understanding how the code would change before committing to modifications.
Explanation:
path/to/input_file
: This represents the file path to the JavaScript file you want to transpile. The output is displayed in the terminal, hence ‘stdout’.
Example Output: Your terminal window might display the transformed JavaScript code directly, showing the changes without saving them to a particular file.
Use case 3: Transpile a file to the specified output file
Code:
lebab path/to/input_file --out-file path/to/output_file
Motivation: This use case is important when you want to preserve the original file and save the transformed JavaScript code in a different file. This action allows developers to experiment with or review transformed code separately before integrating it within a codebase.
Explanation:
path/to/input_file
: The file path pointing to the source JavaScript file.--out-file
: This flag signals Lebab to save the output to a specific file.path/to/output_file
: The file path where the transformed code should be written.
Example Output:
The contents of path/to/input_file
are transformed and saved to path/to/output_file
. The original file remains unchanged.
Use case 4: Replace all .js
files in-place in the specified directory, glob, or file
Code:
lebab --replace src/**/*.js
Motivation: By transforming files in-place, developers can update an entire codebase quickly and effectively. This is exceptionally useful for projects with numerous JavaScript files that need upgrading to use modern ES6+ features.
Explanation:
--replace
: This flag directs Lebab to overwrite existing JavaScript files with their transformed versions.src/**/*.js
: This is a glob pattern used to specify all JavaScript files under thesrc
directory and its subdirectories.
Example Output: The JavaScript files within the specified directory or file path are transformed according to the chosen settings and saved back to their original locations, replacing the old code.
Use case 5: Display help
Code:
lebab --help
Motivation: Using the help command is valuable for both beginners and experienced users of Lebab who need a quick reference of available commands and options. It provides a list of possible transformations and usage guide, which can serve as a handy resource during development.
Explanation:
--help
: This argument prompts Lebab to display a comprehensive guide of available transformations and options.
Example Output: A list of commands and descriptions, similar to a manual, is displayed in the terminal window, outlining all the options you can use with Lebab, including transformation types and usage formats.
Conclusion:
Lebab is a robust tool for modernizing JavaScript codebases with ease. Whether you need to perform a quick transformation on a single file or undertake a large-scale update across an entire project, understanding how to use Lebab efficiently can greatly aid in advancing legacy code to meet modern standards. By following the examples provided, you can leverage the capabilities of Lebab to enhance your JavaScript projects effectively.