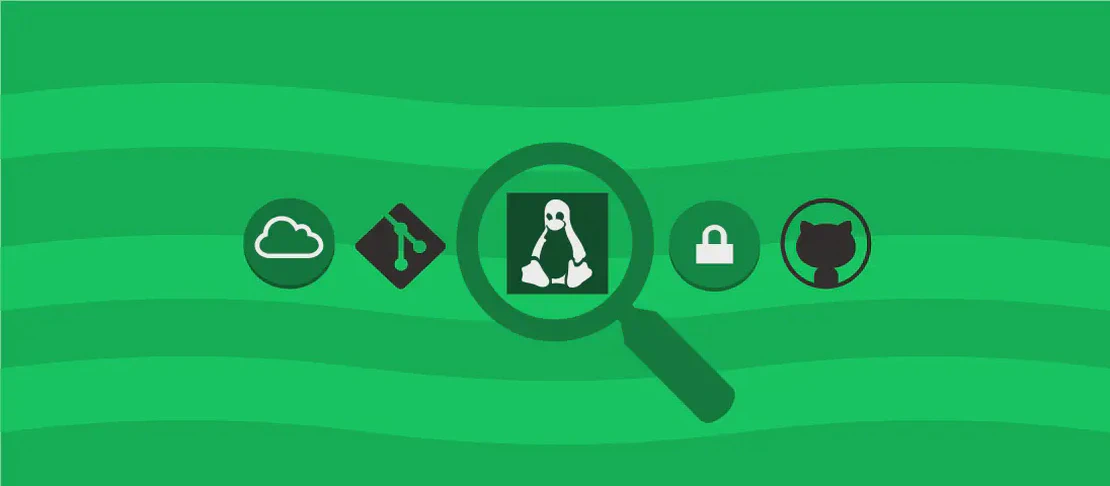
How to Use Input Redirection with the Less Than Symbol (with Examples)
In the world of command-line environments, input and output redirection plays a crucial role by allowing users to direct the flow of data into and out of commands. One fundamental concept in this area is using the less than (<
) symbol to redirect input into a command. This technique is particularly useful in situations where you need to provide data to a command from a source other than the command line itself. Here, we explore three specific use cases of using the less than symbol for input redirection, accompanied by detailed examples and explanations.
Use Case 1: Redirecting a File to stdin
Code:
command < path/to/file.txt
Motivation:
Redirecting a file directly into the standard input (stdin
) of a command is a highly efficient way to process the contents of that file. Suppose you have a program or utility that reads input data but doesn’t take a filename as an argument. Instead of using a pipeline with cat
, you can redirect a file directly. This approach can make scripts neater and potentially enhance performance by avoiding the use of cat
.
Explanation:
command
: This is the command or utility that you wish to execute, which will read data fromstdin
.<
: The less than symbol is used for input redirection. It directs the content of a file into the standard input of the command that precedes it.path/to/file.txt
: This represents the path to the file whose contents you want to provide as input to the command. The command reads the file line by line or process it according to its functionality.
Example Output:
Suppose we use wc
(word count) as the command with a file containing “Hello World”:
wc <path/to/hello.txt
Output:
1 2 11
This output indicates there’s one line, two words, and eleven characters in the file.
Use Case 2: Creating a Here Document and Passing It into stdin
Code:
command << EOF
multiline_data
EOF
Motivation:
A here document is an ideal solution in scenarios where you need to input multiple lines of text directly to a command as though it were being typed into a file. It provides a literal, multiline input source and is particularly effective during scripting, where programs need multiple input lines, such as configurations or command sequences.
Explanation:
command
: This is the command or utility to which you are passing the multiline data. It reads the input directly as if it were coming from a file.<<
: This symbol is used to initiate a here document. It signifies that what follows until the specified delimiter (EOF
in this case) is the input data.EOF
: This serves as both the starting and ending identifier of the here document. The data is read until this token is encountered.multiline_data
: These lines represent the actual data you wish to pass as input. The command processes it as it would a file’s content.
Example Output:
Consider using cat
to echo a simple multiline text:
cat << EOF
Multiline input example!
Another line of input.
EOF
Output:
Multiline input example!
Another line of input.
This demonstrates how the entire block of text is treated as input to the command.
Use Case 3: Creating a Here String and Passing It into stdin
Code:
command <<< string
Motivation:
Using a here string is a quick and efficient way to pass a single line of input directly into stdin
. This avoids the overhead of creating and managing a temporary file or a pipe for just one line of data. It’s particularly helpful when you need to test commands or provide simple inputs within scripts or one-liners.
Explanation:
command
: The command or program that will receive the input from the here string in place of its standard input.<<<
: This operator introduces a here string, which redirects a literal string into the command’sstdin
.string
: The string you wish to pass as input. It is processed immediately by the command.
Example Output:
Let’s pipe a single word “Hello” into the tr
command to convert it to uppercase:
tr 'a-z' 'A-Z' <<< "Hello"
Output:
HELLO
This output shows the transformation of the input string to uppercase, demonstrating the effectiveness of a here string for one-liners.
Conclusion:
Understanding and utilizing input redirection techniques using the less than symbol can greatly enhance your scripting and command-line operations. Whether you’re funneling file contents, multiline text blocs, or single-line inputs into commands, these methods streamline the flow of data and can lead to cleaner, more efficient scripts. These redirection capabilities allow for flexible data management, making command-line operations more dynamic and powerful.