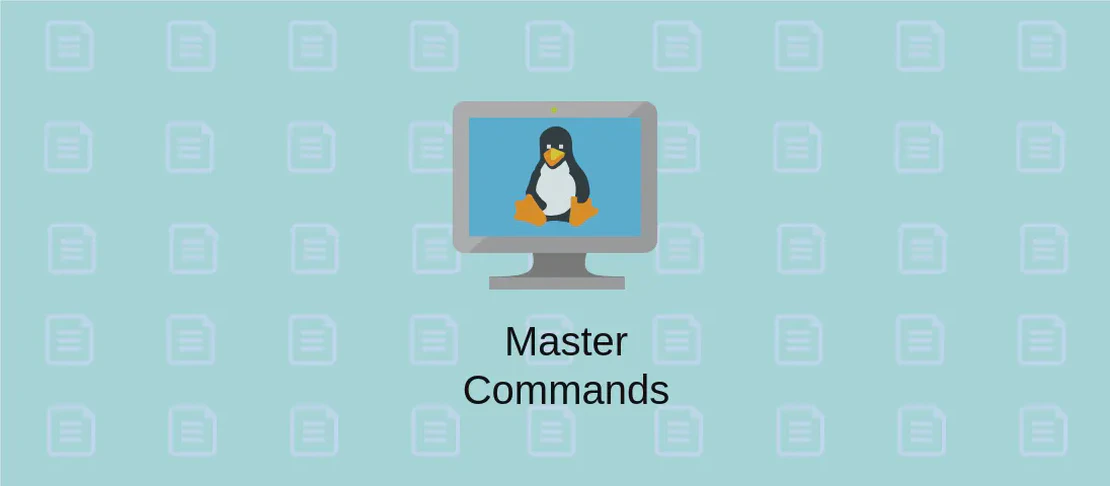
How to Use the Command 'let' in Shell (with Examples)
- Linux
- December 17, 2024
The let
command in shell scripting is a powerful tool used to evaluate arithmetic expressions. It supports various arithmetic operations, including addition, subtraction, multiplication, and division, as well as conditional expressions. By enabling arithmetic operations directly within shell scripts, the let
command provides a straightforward and effective method for performing calculations and logical evaluations within a script. Users can use variables, increment operators, and conditional expressions seamlessly with let
, making it an indispensable tool for bash scripting.
Use case 1: Evaluate a simple arithmetic expression
Code:
let "result = a + b"
Motivation:
In shell scripting, performing arithmetic operations is a common task, whether you’re calculating totals, differences, or averages. By using let
to evaluate simple arithmetic expressions, you can easily manipulate numerical data within your scripts. This particular use case demonstrates how to calculate the sum of two variables. Whether you’re working on budget calculations, aggregating data entries, or even summing configurations, knowing how to perform such basic arithmetic operations is essential.
Explanation:
let
: This invokes the command to evaluate arithmetic expressions."result = a + b"
: Inside the quotation marks, the arithmetic expression is specified. It’s a simple expression that assigns the sum of the variablesa
andb
to the variableresult
. The quotation marks are used to enclose the entire expression to avoid any misinterpretation by the shell.
Example output:
Suppose a=5
and b=10
. The output of the let
command will be:
echo $result # Outputs: 15
Use case 2: Use post-increment and assignment in an expression
Code:
let "x++"
Motivation:
Incrementing a variable is a fundamental operation often employed in loops, counters, or any scenario where variable incrementation is needed. Understanding how to easily and efficiently increment variables is crucial in many programming contexts. This use case focuses on demonstrating how to use the post-increment operator to increase the value of a variable x
by 1.
Explanation:
let
: This is the command used to perform arithmetic operations."x++"
: The post-increment operator++
is used here, which increases the variablex
by 1 after the expression is evaluated. It’s a concise way to update the loop counters or iterators without using more complex syntax.
Example output:
If x=4
initially, after executing the let
command, x
will be:
echo $x # Outputs: 5
Use case 3: Use conditional operator in an expression
Code:
let "result = (x > 10) ? x : 0"
Motivation:
Conditional operations are crucial in programming when decisions need to be made based on the values of variables or expressions. The ternary conditional operator is particularly useful for inline conditional evaluations, where you want to assign a value based on whether a condition holds true or false. This use case illustrates how to use the conditional operator to assign a value to result
depending on whether x
is greater than 10.
Explanation:
let
: Initiates the arithmetic evaluation."result = (x > 10) ? x : 0"
: This expression utilizes the ternary conditional operator.(x > 10)
is the condition being evaluated. If true,result
is assigned the value ofx
; otherwise, it is assigned0
. It’s an efficient one-liner for conditions that otherwise require a more verboseif-else
statement.
Example output:
Assume x=12
. After evaluating the let
command, the value of result
will be:
echo $result # Outputs: 12
If x=7
, then:
echo $result # Outputs: 0
Use case 4: Display help
Code:
let --help
Motivation:
When using any command-line tool, understanding the available options and syntax is critical—especially when starting out or needing a refresher on a less commonly used feature. Displaying help information is often the first step to mastering a command because it provides immediate insights into its usage, arguments, and extended functionalities.
Explanation:
let
: Thelet
command is invoked.--help
: This option prompts the command to display help information. While not all shell commands support--help
, using it with commands that do can provide quick access to reference material and detailed information about command syntax and use-case scenarios.
Example output:
The output will typically display usage information and descriptions of the command’s options and capabilities. However, note that let
might not produce an output with the --help
option in all shell environments, as it is more of a built-in shell command.
Conclusion:
By leveraging the let
command, shell scriptwriters can effectively perform arithmetic operations and conditional evaluations within their scripts. Whether performing simple arithmetic, incrementing values, or using conditional logic, let
simplifies script writing and enhances script performance. Additionally, understanding how to access help can empower users to explore and utilize let
more thoroughly.