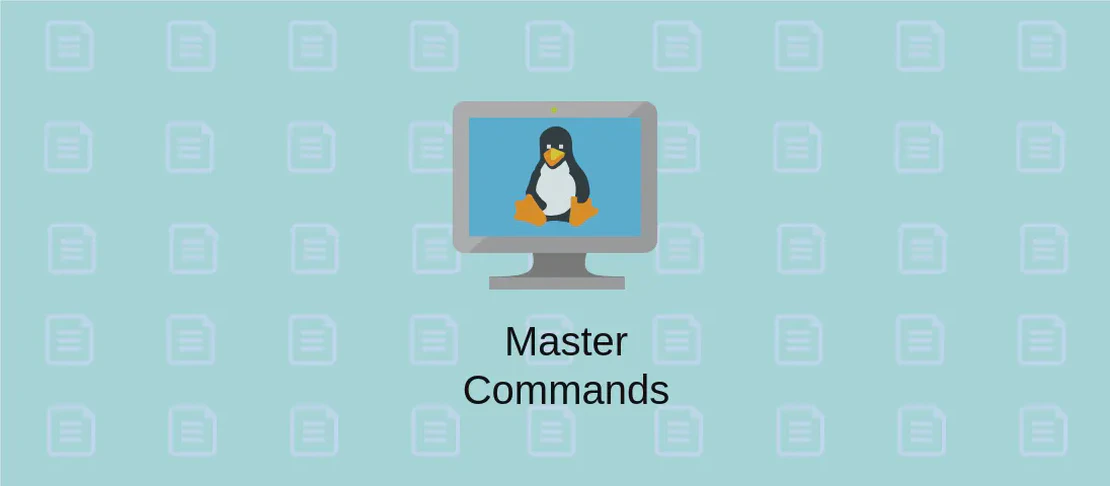
How to use the command 'lex' (with examples)
Lex is a powerful tool used in computer programming for generating lexical analyzers, also known as scanners. A lexical analyzer processes an input sequence of characters (often a source code file) and categorizes these characters into meaningful tokens, which are then used by a parser for syntax analysis. This command reads a specification file containing patterns and actions, typically written in a .l
file, and transforms them into C source code, thereby simplifying the task of developing lexical analyzers. On many operating systems, lex
is simply an alias for flex
, which is a more recent and more widely used implementation of lex.
Use case 1: Generate an analyzer from a Lex file, storing it to the file lex.yy.c
Code:
lex analyzer.l
Motivation for using the example:
The primary motivation for using lex
to generate a lexical analyzer is to streamline the process of tokenizing input data. Writing a lexical analyzer from scratch can be a complex and error-prone task, particularly as the syntax definitions grow more complex. By providing pattern and action definitions in a lex specification file (analyzer.l), developers can automatically generate a C source file that performs these tasks, saving both time and reducing the potential for errors.
Explanation for every argument given in the command:
lex
: Invokes the lexical analyzer generator. The base command necessary for initiating the code generation process.analyzer.l
: This is the input file containing the lex specifications. It defines the rules for tokenizing the input based on regular expressions, alongside actions to perform when these patterns are matched.
Example output:
When this command is executed, it generates a C source file named lex.yy.c
. This file contains the code for a lexical analyzer that can process the rules and patterns defined in analyzer.l
. The output file isn’t typically printed to the console, but checking the directory will reveal the newly created lex.yy.c
file.
Use case 2: Specify the output file
Code:
lex -t analyzer.l > analyzer.c
Motivation for using the example:
Specifying the output file name that lex
generates can be particularly useful in organized project setups and build systems where specific filenames are required. Rather than working with the default lex.yy.c
, renaming the output directly can help integrate the lexical analyzer into larger projects without additional post-processing or renaming steps.
Explanation for every argument given in the command:
lex
: This initiates the lexical analysis generation process.-t
: This option tellslex
to write the generated C code to standard output. Usually, this wouldn’t show up on the console sincelex
typically writes directly tolex.yy.c
.analyzer.l
: The lex specification file as in the first example, containing rules and action definitions.>
: This shell redirection operator captures standard output and writes it to a file instead.analyzer.c
: The specified output file where the generated C code will be written. This allows more control over file naming and management.
Example output:
No visible output is provided on the console due to the use of the >
redirection operator; instead, the generated C code is saved in analyzer.c
. Checking the project directory should reveal an analyzer.c
file, now containing the lex-generated code readable for compilation or further modifications.
Use case 3: Compile a C file generated by Lex
Code:
c99 path/to/lex.yy.c -o executable
Motivation for using the example:
Once the lexical analyzer is generated by lex
, it needs to be compiled and linked into an executable form that can be run independently. The C file output by lex
might include library functions and dependencies that need to be resolved through the compilation process. Utilizing a C compiler such as c99
ensures the code is transformed into an efficient, runnable program.
Explanation for every argument given in the command:
c99
: This command invokes the C99 standard compiler, which compiles C source files complying with the C99 standard.path/to/lex.yy.c
: This points to the C source file that was generated by the lex process. It must be specified with the correct path if not in the current directory.-o
: This option specifies the output format. It allows the compiled binary to be named according to the user’s preference.executable
: This is the chosen name for the resulting output file, the final compiled binary that users can run. Naming it allows clarity on what the compiled program is designed to do.
Example output:
Running this command does not produce a visible output on the terminal; however, an executable file named executable
is produced in the working directory. This file is the compiled and linked version of the C code, which can be executed to perform lexical analysis based on the rules defined in analyzer.l
.
Conclusion
Using lex
for generating and compiling lexical analyzers significantly simplifies the process of tokenization in language processing tasks. The command’s ability to translate high-level specifications into structured C code means that developers can focus more on defining intricate patterns and behaviors and can spend less time dealing with the complexities of C programming directly. The demonstrated use cases highlight how lex
becomes a valuable asset in managing, outputting, and compiling lexical analysis processes efficiently.