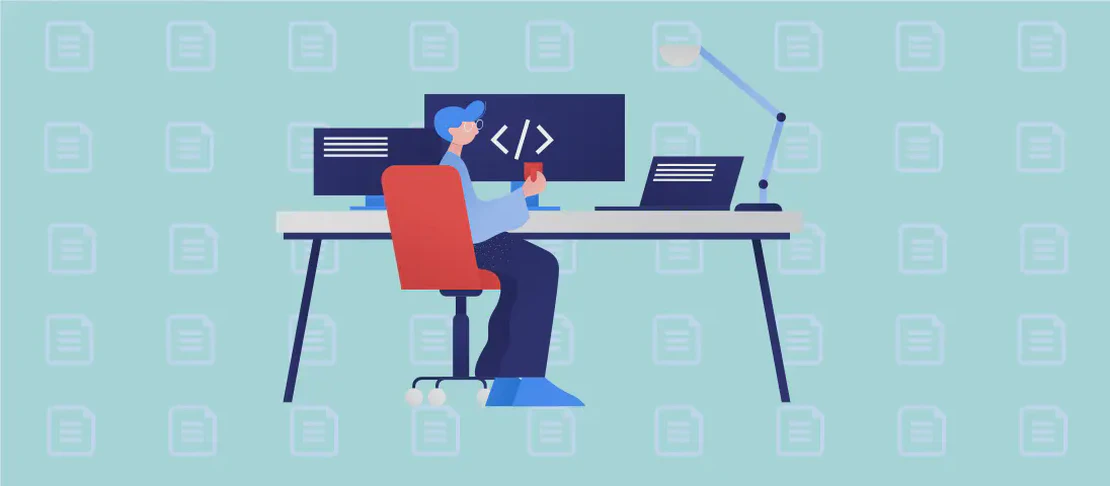
How to Use the Command 'llc' (with Examples)
The llc
command is a powerful utility used in the LLVM (Low Level Virtual Machine) compiler infrastructure. It is primarily used for compiling LLVM Intermediate Representation (IR) or bitcode into target-specific assembly language. This process is essential in various stages of software development where performance optimization and the ability to run on multiple platforms are of paramount importance. The llc
command supports a wide array of options allowing developers to fine-tune the way assembly is generated, tailored to their specific needs.
Use case 1: Compile a bitcode or IR file to an assembly file with the same base name
Code:
llc path/to/file.ll
Motivation:
This use case demonstrates a fundamental scenario wherein a developer wants to translate their LLVM IR file into an assembly language file. This is typically one of the first steps in the compilation pipeline, bridging the gap between human-readable high-level constructs and machine-readable binaries. It becomes particularly pertinent when developers wish to inspect or modify machine-level code manually, ensuring that the expected translation from IR to assembly occurs seamlessly.
Explanation:
llc
: Invokes the LLVM static compiler, which is responsible for converting LLVM IR to assembly language.path/to/file.ll
: Specifies the path to the input file, which in this scenario is an LLVM IR file. The.ll
extension indicates that the file contains LLVM IR code.
Example Output:
Upon execution, an assembly file is generated, possessing the same base name as the input file. If the input file was example.ll
, the output assembly file would be example.s
.
Use case 2: Enable all optimizations
Code:
llc -O3 path/to/input.ll
Motivation:
Optimization levels in compilers allow developers to generate more efficient code, potentially improving runtime performance and reducing resource usage. Using -O3
enables all available optimizations, which is especially vital for performance-critical applications, guaranteeing the best possible assembly output that the compiler can produce.
Explanation:
-O3
: This flag activates the highest level of optimization available inllc
. It combines several transformation and analysis passes to maximize performance, taking into account both compile time and execution efficiency.path/to/input.ll
: Indicates the input LLVM IR file to be compiled.
Example Output:
The result is an assembly file derived from the optimized intermediate representation where computational efficiency improvements can be observed in the code behavior.
Use case 3: Output assembly to a specific file
Code:
llc --output path/to/output.s path/to/input.ll
Motivation:
In scenarios requiring specific naming conventions or directory structures, directing the assembly output to a designated file ensures compliance with project guidelines. This ability also clarifies the build process, as the output file is explicitly named, reducing confusion and aligning with automation scripts.
Explanation:
--output path/to/output.s
: Directsllc
to store the assembly result in the specified file. This flexibility allows for better organization and management of the compiled results.path/to/input.ll
: Represents the input LLVM IR file which is used as the source for generating the assembly code.
Example Output:
The specified output file, say output.s
, will contain the translated assembly code, neatly placed at the defined location within the file system.
Use case 4: Emit fully relocatable, position independent code
Code:
llc -relocation-model=pic path/to/input.ll
Motivation:
Position-independent code (PIC) is crucial for shared libraries and dynamic loading scenarios. It allows code to execute regardless of its absolute address in memory, making it highly adaptable and secure. This is important for applications requiring extensive memory management and security, as it reduces the likelihood of code exploitation through predictable memory addresses.
Explanation:
-relocation-model=pic
: Instructsllc
to generate code that is position-independent. This is particularly important for ensuring the portability and safety of the code when loaded in different memory spaces.path/to/input.ll
: The source LLVM IR file from which PIC assembly is generated.
Example Output:
The resulting assembly file will contain directives optimized for relocatability, suitable for dynamic linkage in shared libraries or other environments necessitating flexible code placement.
Conclusion:
The llc
command, part of the LLVM compiler suite, provides a robust means of translating LLVM IR into assembly language, accompanied by a wealth of options for optimization and output control. Whether optimizing code, directing output, or ensuring position-independent assembly, llc
offers the flexibility and precision needed in modern software development and compiler construction. Through the examples discussed, it becomes evident how llc
can be leveraged to address various compiler demands efficiently and effectively.