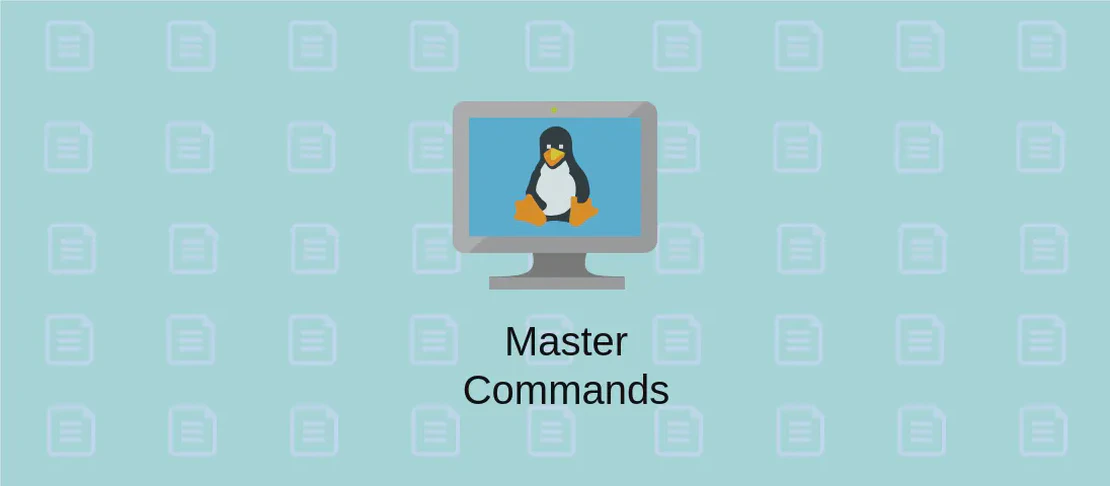
How to use the command 'lldb' (with examples)
The LLVM Low-Level Debugger, commonly known as LLDB, is a debugger for the C, C++, and Objective-C programming languages. Part of the LLVM project, LLDB serves as a powerful tool for developers who need to analyze and resolve issues in their code. It offers essential functionalities like setting breakpoints, inspecting memory and variables, and controlling execution to assist in identifying bugs and performance bottlenecks effectively. With an easy-to-use interface and extensive documentation, LLDB is widely regarded as a reliable, robust debugger that integrates seamlessly with various development environments.
Debug an executable
Code:
lldb executable
Motivation for using this example:
Debugging an executable is one of the most fundamental tasks when developing or testing software. When a program doesn’t work as expected, stepping through its execution can give insights into the cause. This is especially useful for identifying runtime errors, incorrect logic, or memory issues. By using LLDB to debug an executable, developers can observe how their program behaves, examine its state at various execution points, and make necessary corrections.
Explanation of the command:
lldb
: This is the command to invoke the LLVM Low-Level Debugger. Here, it starts a new LLDB session.executable
: This argument is the path to the compiled executable file you wish to debug. By providing this argument, LLDB launches and prepares to debug the specified program.
Example output:
When you run lldb executable
, you might see output similar to the following:
(lldb) target create "executable"
Current executable set to 'executable' (x86_64).
(lldb)
This indicates that LLDB has successfully loaded the specified executable and is ready to accept debugging commands.
Attach lldb
to a running process with a given PID
Code:
lldb -p pid
Motivation for using this example:
Attaching LLDB to a running process is beneficial when a problem only occurs in a specific environment, making it difficult to replicate through normal debugging methods. By connecting to an active process, developers can inspect the state of a live application, evaluate memory utilization, variable states, and even alter the program’s flow without the need for recompilation or restarting.
Explanation of the command:
lldb
: Launches the debugger.-p pid
: This option tells LLDB to attach to a running process identified by its Process ID (PID). Thepid
here should be replaced by the actual numeric identifier of the process you want to debug.
Example output:
Running lldb -p 1234
(assuming 1234 is the PID) gives:
(lldb) attach to process 1234
Process 1234 stopped
* thread #1: tid = 1234, 0x00007fff8e2e257a libsystem_kernel.dylib`mach_msg_trap + 10, stop reason = signal SIGSTOP
frame #0: 0x00007fff8e2e257a libsystem_kernel.dylib`mach_msg_trap + 10
(lldb)
This output shows that LLDB has successfully attached to the process with PID 1234 and halted execution, allowing the developer to begin debugging commands.
Wait for a new process to launch with a given name, and attach to it
Code:
lldb -w -n process_name
Motivation for using this example:
In some situations, developers might need to debug a process that has yet to start, particularly during the startup phase of an application. By instructing LLDB to wait for a new process with a specific name to launch, developers can automatically attach the debugger as soon as the process begins execution. This can be critical for diagnosing issues that occur only at startup or for processes that are not easily triggered manually.
Explanation of the command:
lldb
: Initiates the LLVM Low-Level Debugger.-w
: The wait option causes LLDB to wait for a specified process.-n process_name
: Specifies the name of the executable you expect to run. LLDB waits until a process matching this name launches and then attaches to it automatically.
Example output:
Executing lldb -w -n myapp
results in:
(lldb) process attach --name "myapp" --waitfor
Process 5678 stopped
* thread #1: tid = 5678, 0x00007fff8e2e257a libsystem_kernel.dylib`mach_msg_trap + 10, stop reason = signal SIGSTOP
frame #0: 0x00007fff8e2e257a libsystem_kernel.dylib`mach_msg_trap + 10
This output shows that LLDB successfully waited for and attached to the process named “myapp” once it started, halting execution for debugging.
Conclusion:
Using LLDB offers developers a powerful way to inspect, diagnose, and correct issues within their applications by providing tools to debug executables, attach to running processes, and anticipate process launches. Through these illustrative use cases, users can harness LLDB’s capabilities to improve development efficiency and software robustness effectively.