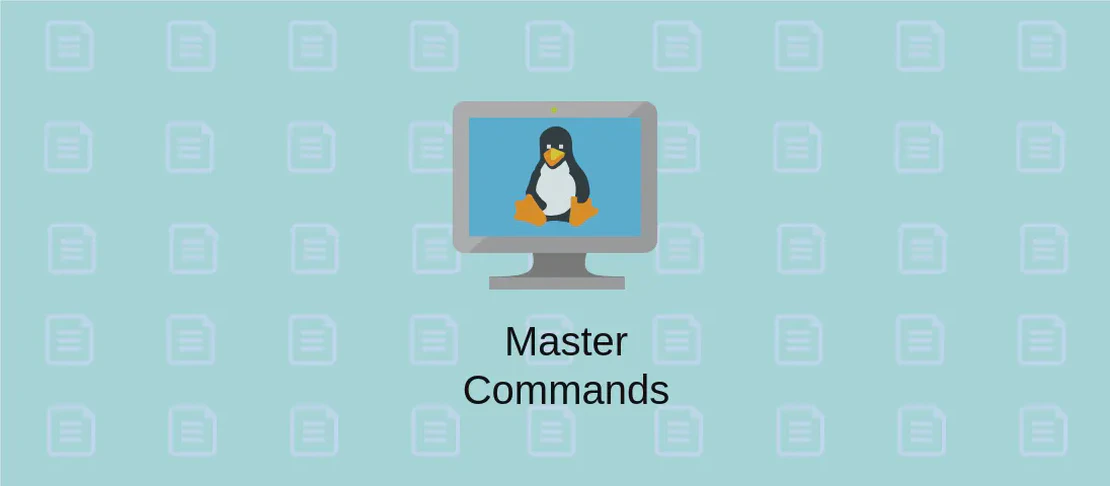
How to use the command lldb (with examples)
- Osx
- December 25, 2023
LLDB is the LLVM Low-Level Debugger, which is used for debugging programs written in C, C++, Objective-C, and Swift. It provides a command-line interface to examine and manipulate the state of programs while they are running.
Use case 1: Debug an executable
Code:
lldb "executable"
Motivation: This use case is helpful when you want to debug an executable file to identify and fix issues in the code. By running the executable in LLDB, you can step through the code, set breakpoints, inspect variables, and analyze the program’s behavior.
Explanation:
lldb
: The command to start the LLDB debugger."executable"
: The path to the executable file that you want to debug.
Example output:
(lldb) target create "executable"
Current executable set to 'executable' (x86_64).
(lldb) b main
Breakpoint 1: where = executable`main, address = 0x0000000100000f80
(lldb) run
Process 1234 stopped
* thread #1, queue = 'com.apple.main-thread', stop reason = breakpoint 1.1
[...]
Use case 2: Attach lldb
to a running process with a given PID
Code:
lldb -p pid
Motivation: This use case is helpful when you want to attach LLDB to an already running process. It allows you to inspect the state of the program, set breakpoints, and analyze its execution.
Explanation:
lldb
: The command to start the LLDB debugger.-p pid
: The-p
option is used to specify the process ID (PID) of the running process that you want to attach to.
Example output:
(lldb) attach -p 1234
Process 1234 stopped
* thread #1, queue = 'com.apple.main-thread', stop reason = signal SIGSTOP
[...]
Use case 3: Wait for a new process to launch with a given name, and attach to it
Code:
lldb -w -n "process_name"
Motivation: This use case is useful when you want to wait for a specific process to launch and attach to it for debugging. It is commonly used when you need to debug a process that is launched by another program or script, and you want to start debugging as soon as the process becomes available.
Explanation:
lldb
: The command to start the LLDB debugger.-w
: The-w
option makes LLDB wait for the process to launch before attaching to it.-n "process_name"
: The-n
option is used to specify the name of the process that you want to wait for and attach to.
Example output:
Waiting for process "process_name"...
Process "process_name" has launched!
Process 5678 stopped
* thread #1, queue = 'com.apple.main-thread', stop reason = breakpoint 1.1
[...]
Conclusion:
In this article, we explored three different use cases of the LLDB command. We learned how to debug an executable file, attach LLDB to a running process with a given PID, and wait for a new process to launch with a given name. LLDB is a powerful debugger that provides various features and options to help developers identify and fix issues in their programs.