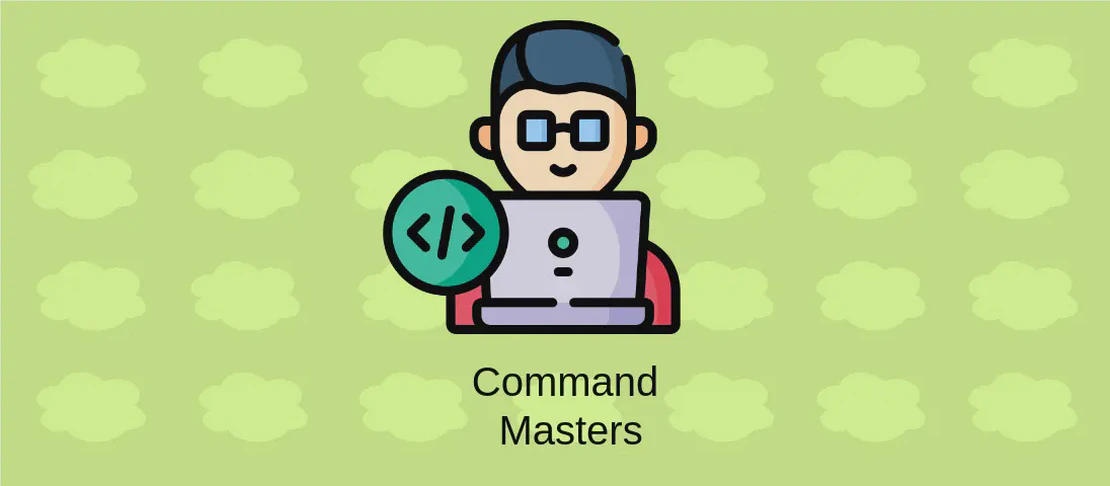
How to Use the Command 'lli' (with Examples)
The lli
command is a part of the LLVM infrastructure, which allows you to directly execute programs from LLVM bitcode. This command serves as an interpreter for LLVM bytecode, enabling developers to test and run their code efficiently without needing to compile it to machine code first. By utilizing lli
, you can execute LLVM intermediate representations and test your applications with various options to optimize performance.
Use case 1: Execute a Bitcode or IR File
Code:
lli path/to/file.ll
Motivation:
Executing a bitcode file or intermediate representation (IR) file directly helps developers quickly test that the code behaves as expected. This is particularly useful during the development and debugging phase, where you want to see the output of your code without incurring the overhead of compiling it down to machine code.
Explanation:
lli
: The command used to invoke the LLVM interpreter.path/to/file.ll
: The path to the LLVM bitcode or IR file you wish to execute..ll
is the file extension typically used for LLVM IR files.
Example Output:
Upon executing this command, you will observe the standard output or results of the bitcode file. If there are print statements or specific functions called within the code, they will display their outputs in your terminal window.
Use case 2: Execute with Command-line Arguments
Code:
lli path/to/file.ll argument1 argument2 ...
Motivation:
In many scenarios, testing code involves passing arguments to simulate various states or inputs the code will deal with in a real-world application. This command allows you to develop and test how your bitcode will handle different inputs by passing them directly through the command line.
Explanation:
lli
: The interpreter for executing LLVM bitcode.path/to/file.ll
: The LLVM bitcode or IR file to be executed.argument1
,argument2
, …: These are the positional command-line arguments you provide to the code. The code executed bylli
may read and use these arguments.
Example Output:
The output will reflect variations based on the passed arguments. For example, if the program processes input and returns results, such as calculating the sum of numbers, providing different arguments will yield corresponding results.
Use case 3: Enable All Optimizations
Code:
lli -O3 path/to/file.ll
Motivation:
Optimization is a significant step in the development process to ensure that programs run efficiently. By using this command, you can enable all optimizations at level 3 (-O3
), which is typically the most aggressive optimization level. This helps in testing the maximum potential efficiency of your program in terms of speed and performance.
Explanation:
lli
: The LLVM interpreter command.-O3
: This flag enables the highest level of optimization, enhancing the performance by improving the speed at which the code executes. It optimizes code execution and may make significant changes to code behavior to streamline performance.path/to/file.ll
: The path to the LLVM bitcode or IR file that you are optimizing and executing.
Example Output:
The output should ideally remain the same in terms of the data produced by the program. However, under the hood, the file has been executed using optimizations that may reduce execution time or improve performance metrics without altering the logic output.
Use case 4: Load a Dynamic Library Before Linking
Code:
lli --dlopen=path/to/library.dll path/to/file.ll
Motivation:
Applications often rely on external libraries for additional functionality. Sometimes, during the execution of bitcode, a required external library must be loaded. This command allows you to specify that a dynamic library should be loaded beforehand, enabling correct execution especially for code that relies on shared library functions.
Explanation:
lli
: The interpreter for executing LLVM code.--dlopen=path/to/library.dll
: This specifies that a custom shared library, identified here bylibrary.dll
, should be dynamically loaded before the bitcode execution. This is critical for the execution of bitcode that depends on external functions defined in the shared library.path/to/file.ll
: The LLVM bitcode you intend to run with the help of the specified dynamic library.
Example Output:
Utilizing an external library, the output may involve function calls that are reliant on the dynamic library. If, for example, the library processes or transforms data, the bitcode results will reflect this, offering extended or modified functionality compared to executing without the library.
Conclusion
Using the lli
command allows developers to seamlessly execute and test their LLVM bitcode in various scenarios. Whether you want to quickly run a program, pass different inputs, apply optimizations, or utilize dynamic libraries, lli
provides the flexibility needed to evaluate your code thoroughly. These use cases demonstrate lli
as a powerful tool for working within the LLVM ecosystem.