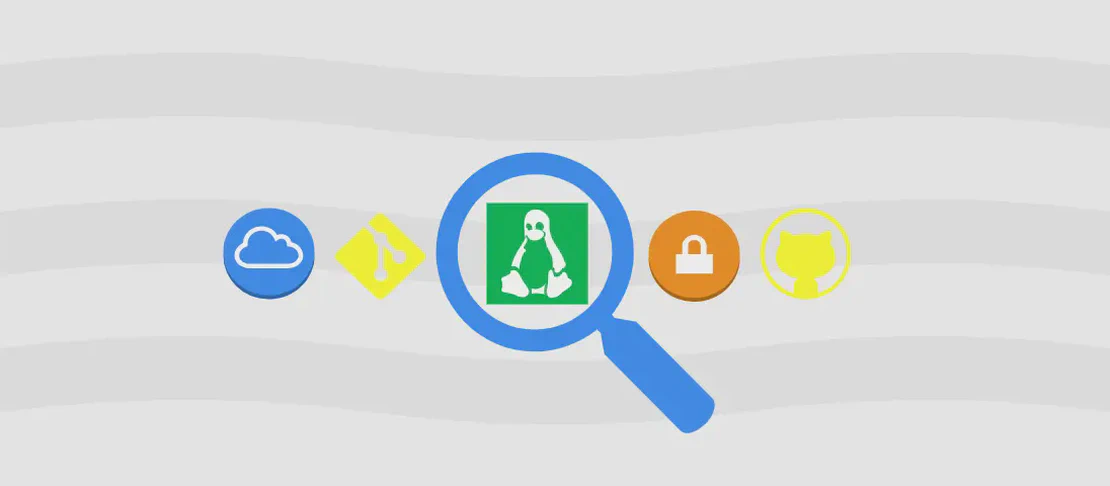
How to use the command 'llm' (with examples)
The ’llm’ command allows users to interact with Large Language Models (LLMs) through remote APIs and models that can be installed and run on their local machine. It provides a range of functionalities for running prompts, system prompts, installing packages, and downloading and running prompts against specific models.
Use case 1: Set up an OpenAI API Key
Code:
llm keys set openai
Motivation: Setting up an OpenAI API Key allows users to authenticate and access the OpenAI API, which is required to use the ’llm’ command with OpenAI models.
Explanation:
llm
: The command itself.keys set openai
: This subcommand sets up an OpenAI API Key for authentication.
Example output:
Successfully set up OpenAI API Key.
Use case 2: Run a prompt
Code:
llm "Ten fun names for a pet pelican"
Motivation: Running a prompt allows users to generate text based on the given input. It can be useful for brainstorming ideas or generating creative content.
Explanation:
llm
: The command itself."Ten fun names for a pet pelican"
: The prompt text enclosed in double quotes.
Example output:
1. Percy the Pelican
2. Swoop
3. Pippin
4. Feathers
5. Splash
6. Pelecanus
7. Breeze
8. Fisherman
9. Skye
10. Psyche
Use case 3: Run a [s]ystem prompt against a file
Code:
cat path/to/file.py | llm --system "Explain this code"
Motivation: Running a system prompt against a file allows users to get explanations or descriptions of code snippets. It can be useful for understanding unfamiliar code or debugging.
Explanation:
cat path/to/file.py
: Reads the contents of the specified file and pipes it to the ’llm’ command.llm
: The command itself.--system
: Specifies that the prompt is a system prompt."Explain this code"
: The prompt text enclosed in double quotes.
Example output:
This code is implementing a bubble sort algorithm to sort an array of integers. The input array is iterated multiple times, comparing adjacent elements and swapping them if they are in the wrong order. This process is repeated until the array is sorted in ascending order.
Use case 4: Install packages from PyPI into the same environment as LLM
Code:
llm install package1 package2 ...
Motivation: Installing packages from PyPI allows users to extend the functionality of LLM and access additional libraries or modules.
Explanation:
llm
: The command itself.install
: Specifies that packages need to be installed.package1 package2 ...
: The names of the packages to install, separated by spaces.
Example output:
Successfully installed package1 version x.x.x.
Successfully installed package2 version y.y.y.
Use case 5: Download and run a prompt against a [m]odel
Code:
llm --model orca-mini-3b-gguf2-q4_0 "What is the capital of France?"
Motivation: Downloading and running a prompt against a specific model allows users to utilize the capabilities of that model for generating responses to prompts or questions.
Explanation:
llm
: The command itself.--model orca-mini-3b-gguf2-q4_0
: Specifies the model to be used, which is the ‘orca-mini-3b-gguf2-q4_0’ in this example."What is the capital of France?"
: The prompt text enclosed in double quotes.
Example output:
The capital of France is Paris.
Use case 6: Have an interactive chat with a specific [m]odel
Code:
llm chat --model chatgpt
Motivation: Having an interactive chat with a specific model allows users to engage in natural language conversations and obtain responses to their queries or statements.
Explanation:
llm
: The command itself.chat
: Specifies the chat mode.--model chatgpt
: Specifies the model to be used, which is the ‘chatgpt’ in this example.
Example output:
User: What is the weather like today?
LLM: The weather is sunny and warm with a slight breeze.
User: Any chances of rain?
LLM: No, there is no rain expected today.
User: Thanks for the information!
LLM: You're welcome! Let me know if there's anything else I can help with.
Conclusion:
The ’llm’ command provides a versatile set of functions for interacting with Large Language Models. Whether it’s generating text, explaining code, installing packages, or engaging in conversations, the ’llm’ command offers a convenient way to harness the power of language models for various tasks.