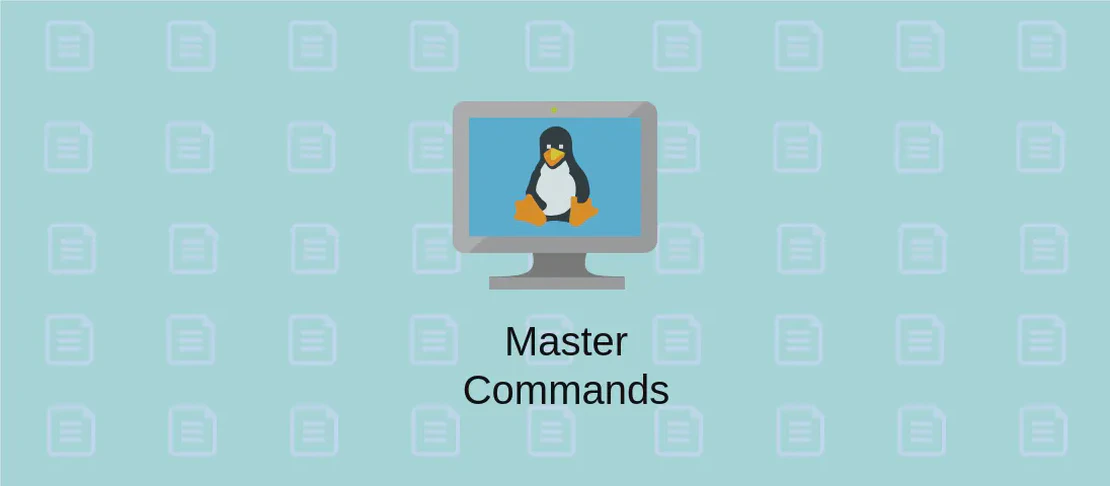
How to use the command llvm-as (with examples)
The llvm-as
command is used to assemble LLVM Intermediate Representation (.ll
) files into LLVM Bitcode (.bc
) files. It takes an IR file as input and produces a corresponding Bitcode file.
Use case 1: Assemble an IR file
Code:
llvm-as -o path/to/out.bc path/to/source.ll
Motivation: This use case is suitable when you have an IR file and you want to convert it into a Bitcode file. The Bitcode file can then be used as input for other LLVM tools or in LLVM-based compilers.
Explanation:
llvm-as
: The command name.-o path/to/out.bc
: This option specifies the output file path and name for the generated Bitcode file.path/to/source.ll
: This is the input file, which is the IR file to be assembled into Bitcode.
Example output:
The Bitcode file out.bc
will be generated at the specified output path path/to/out.bc
.
Use case 2: Assemble an IR file and include a module hash
Code:
llvm-as --module-hash -o path/to/out.bc path/to/source.ll
Motivation: Including a module hash in the Bitcode file can provide additional verification and security when using the Bitcode in subsequent stages of software development or analysis. The module hash is a unique identifier of the module contents.
Explanation:
--module-hash
: This option instructsllvm-as
to include a module hash in the produced Bitcode file.-o path/to/out.bc
: This option specifies the output file path and name for the generated Bitcode file.path/to/source.ll
: This is the input file, which is the IR file to be assembled into Bitcode.
Example output:
The Bitcode file out.bc
, including the module hash, will be generated at the specified output path path/to/out.bc
.
Use case 3: Read an IR file from stdin
Code:
cat path/to/source.ll | llvm-as -o path/to/out.bc
Motivation:
This use case is useful when you want to assemble an IR file without creating an intermediate file on disk. It allows you to read the IR file from stdin
and directly produce the corresponding Bitcode.
Explanation:
cat path/to/source.ll
: Thecat
command reads the content of the IR file from the specified path and outputs it tostdout
.|
: This pipe character redirects thestdout
of the previous command (cat
) as thestdin
for thellvm-as
command.-o path/to/out.bc
: This option specifies the output file path and name for the generated Bitcode file.
Example output:
The Bitcode file out.bc
will be generated at the specified output path path/to/out.bc
.