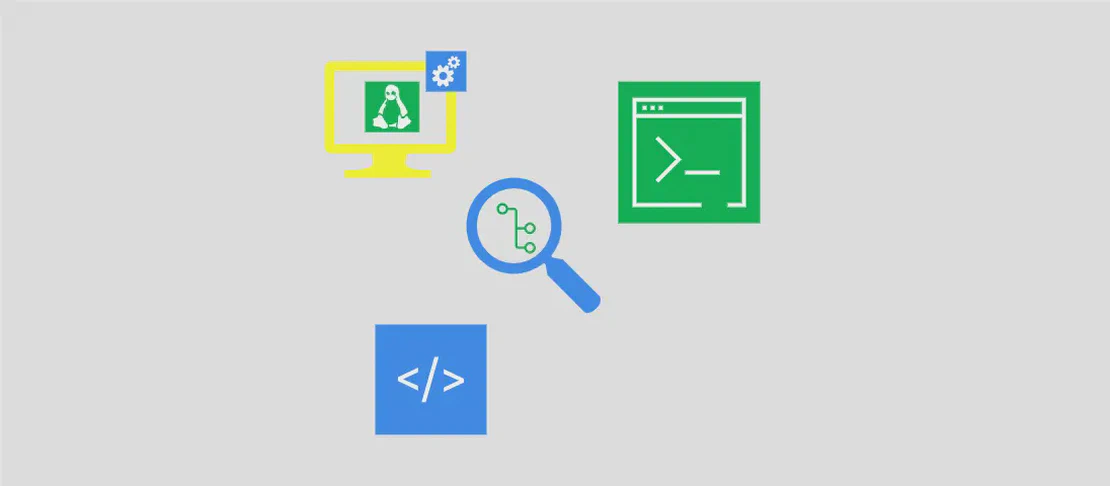
Using the llvm-config Command (with examples)
1: Compiling and Linking an LLVM Based Program
To compile and link an LLVM based program, you can use the clang++
command in combination with llvm-config
. The llvm-config
command is used to retrieve various configuration information needed to compile programs that utilize LLVM.
The following code snippet demonstrates how to compile and link an LLVM based program:
clang++ $(llvm-config --cxxflags --ldflags --libs) --output path/to/output_executable path/to/source.cc
Motivation:
Compiling and linking an LLVM based program can be a complex task, especially when dealing with dependencies and different configurations. By using llvm-config
, you can easily retrieve the necessary configuration flags and libraries needed to correctly compile and link your program.
Explanation:
$(llvm-config --cxxflags)
retrieves the necessary C++ compiler flags for compiling with LLVM, such as include directories and additional preprocessor definitions.$(llvm-config --ldflags)
retrieves the necessary linker flags for linking with LLVM, such as library directories.$(llvm-config --libs)
retrieves the necessary LLVM libraries to link against.
Example Output:
Suppose you have an LLVM based program located at path/to/source.cc
and you want to compile and link it into an executable file called output_executable
. Running the example code snippet would generate the executable file in the specified output path.
2: Printing the PREFIX of your LLVM Installation
You can use the llvm-config
command to print the PREFIX
of your LLVM installation. The PREFIX
refers to the directory where your LLVM installation is located.
The following code demonstrates how to print the PREFIX
of your LLVM installation:
llvm-config --prefix
Motivation:
Knowing the PREFIX
of your LLVM installation can be useful in various scenarios, such as when configuring other tools or when referencing LLVM components in build scripts.
Example Output:
Running the code snippet will display the PREFIX
of your LLVM installation, providing you with the directory path where LLVM is installed.
3: Printing all Targets Supported by your LLVM Build
The llvm-config
command can be used to retrieve a list of all targets supported by your LLVM build. The targets refer to the different architectures or platforms that LLVM has been built to target.
To print all the targets supported by your LLVM build, use the following code:
llvm-config --targets-built
Motivation:
Knowing the targets supported by your LLVM build is important when developing software that targets specific architectures or platforms. This information helps ensure that your code is compatible with the intended target.
Example Output:
Executing the code snippet will display a list of all the supported targets in your LLVM build. This can include architectures like x86, ARM, MIPS, or platforms like Linux, macOS, or Windows.