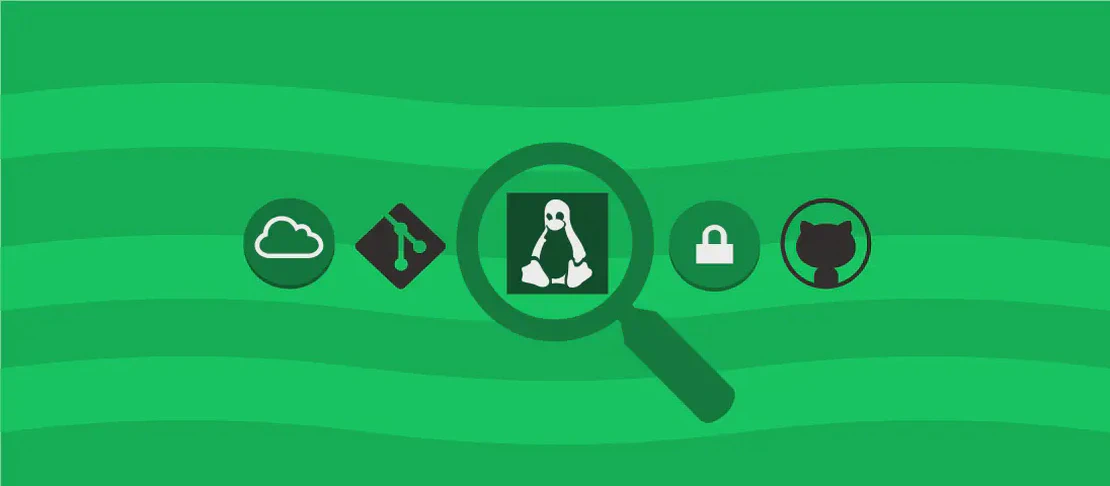
How to Use the Command 'llvm-dis' (with Examples)
The llvm-dis
command is a tool from the LLVM (Low-Level Virtual Machine) suite that allows developers to convert LLVM bitcode files into a human-readable format known as LLVM Intermediate Representation (IR). This conversion is especially useful for those who wish to analyze, understand, or debug compiled code by examining its intermediate representation. This command facilitates a deeper understanding of what occurs between the high-level source code and the final machine code.
Use Case 1: Convert a Bitcode File as LLVM IR and Write the Result to stdout
Code:
llvm-dis path/to/input.bc -o -
Motivation:
This use case is perfectly suited for developers who want to quickly inspect the LLVM IR generated from a bitcode file without any intermediate storage. By writing the output to stdout
, developers can immediately view the IR in the terminal, enabling rapid analysis or pipelining the output to other tools. Such a requirement arises frequently during development cycles where quick feedback is essential for debugging or optimization purposes.
Explanation:
llvm-dis
: The command used to perform the conversion process from bitcode to LLVM IR.path/to/input.bc
: This is the input bitcode file that you want to convert. The file path should point to a compiled source file saved in LLVM bitcode format.-o -
: The-o
flag specifies the output destination, and using-
as the argument tellsllvm-dis
to write the output to standard output (stdout
). This means the converted IR text will be printed directly in the terminal.
Example Output: Running the above command might output a series of LLVM IR instructions, typically consisting of textual representations of functions, control flow constructs, and typed operations. For example:
; ModuleID = 'input.bc'
source_filename = "input.bc"
define i32 @main() {
entry:
ret i32 0
}
Use Case 2: Convert a Bitcode File to an LLVM IR File with the Same Filename
Code:
llvm-dis path/to/file.bc
Motivation:
This example is used when a developer prefers a straightforward solution to convert a bitcode file and examine its associated IR without specifying additional output filenames. By default, llvm-dis
appends a .ll
extension to the original file’s name, storing the IR in a parallel file. This approach minimizes command overhead and maintains a consistent and predictable naming pattern for converted files, which is beneficial for systematic code analysis or backup.
Explanation:
llvm-dis
: Again, this is the command to initiate the conversion from the bitcode file.path/to/file.bc
: This points to the bitcode file you wish to convert. Without a specified output file, the command generates an LLVM IR file namedfile.ll
in the same directory.
Example Output:
Upon execution, a file named file.ll
will appear in the specified directory, containing the IR representation of the original bitcode. Checking the file content using a text editor will reveal LLVM IR, similar to the following:
; ModuleID = 'file.bc'
source_filename = "file.bc"
define i32 @main() {
entry:
ret i32 0
}
Use Case 3: Convert a Bitcode File to LLVM IR, Writing the Result to the Specified File
Code:
llvm-dis path/to/input.bc -o path/to/output.ll
Motivation: This use case is essential for scenarios where specific output management or categorization is needed. By defining a specific output file, developers can organize IR files into directories or give them descriptive names aligning with project documentation or internal conventions. Such an approach is advantageous in large projects requiring strict version control or when preparing IR files for further automated processing.
Explanation:
llvm-dis
: The transformation tool converting bitcode into LLVM IR.path/to/input.bc
: The location of the input file holding the bitcode that you’re targeting for conversion.-o
: This flag explicitly sets the output destination.path/to/output.ll
: The specified file path where the IR output will be written. This provides flexibility in naming and directory structure.
Example Output:
Execution of this command will create or overwrite the specified output.ll
file with the IR content. When opened, the content format will resemble standard LLVM IR:
; ModuleID = 'input.bc'
source_filename = "input.bc"
define i32 @main() {
entry:
ret i32 0
}
Conclusion:
The llvm-dis
command is a versatile and robust tool for any developer working with LLVM-based projects. By understanding its various use cases, users can tailor it to fit different needs, from quick viewing in the terminal to managing precise output files for further use or analysis. Its ability to convert binary LLVM bitcode into a more human-readable format significantly aids in both debugging and educational contexts.