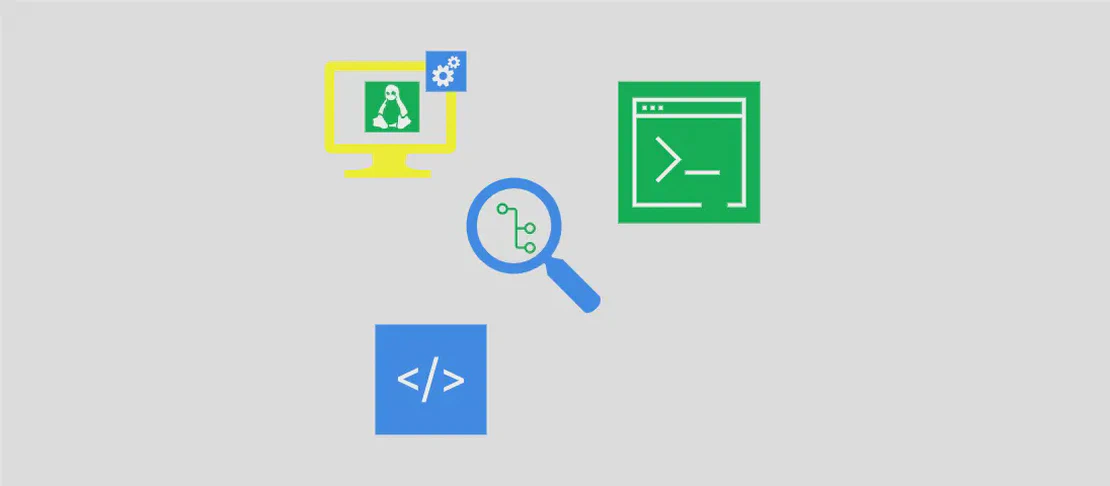
How to use the command 'local' (with examples)
The local
command is primarily used within function blocks in Bash to declare variables that have a local scope. This means they are confined to the function in which they are declared, preventing their values from interfering with or being overridden by variables with the same name outside of the function. It enables programmers to manage the data within specific function calls effectively, ensuring clean and conflict-free code practices. The local command can be used to declare strings, integers, arrays, and associative arrays among other variable types, each with specific flags or attributes that define their type or behavior within the function’s scope.
Use case 1: Declare a string variable with the specified value
Code:
local variable="value"
Motivation for using the example:
The motivation behind declaring a string variable as local is to ensure that the variable is unique to the function’s context. When you place code within a function, you might need to reuse variable names or ensure that the variable used doesn’t affect or isn’t affected by the same name variable in a larger script. Using local
helps manage this scope, retaining variable usage to very specific parts of the codebase which reduces errors and logical overlap.
Explanation:
local
: Indicates the variable is confined to the function’s scope.variable
: Represents the variable name being declared."value"
: The string assigned to the declared variable. This can be any text you wish to store within the variable during its lifecycle in the function.
Example output:
Within a function, when echo $variable
is executed, it would output value
if this line was used in setting that variable.
Use case 2: Declare an integer variable with the specified value
Code:
local -i variable="value"
Motivation for using the example:
When handling numerical operations, it can be beneficial to declare a variable as an integer explicitly. This separation not only helps in ensuring that the operations performed are numerical (preventing the string type operations by mistake), but it also inherently makes any assignment or operational flaw more evident in your code as it will adhere strictly to integer characteristics.
Explanation:
local
: Restricts the visibility of the variable to the local scope of a function.-i
: Specifies that the variable is an integer, ensuring that arithmetic operations can be performed directly and string assignments are flagged.variable
: The name for the integer variable being declared."value"
: The numerical value assigned to that variable.
Example output:
If a function tries to set variable="foo"
after this declaration, it will cause an error since “foo” is not an integer.
Use case 3: Declare an array variable with the specified value
Code:
local variable=(item_a item_b item_c)
Motivation for using the example:
Arrays are essential in handling collections of items, whether they be pieces of data or other values that need to be coordinated together. Keeping an array local ensures a controlled and isolated environment, even when elements may share common names or use similar data types with other arrays outside that function.
Explanation:
local
: Limits variable scope to the local function.variable
: The identifier for the array being defined.(item_a item_b item_c)
: The elements stored within this array; it initializes the array with specific items at declaration.
Example output:
When executing echo ${variable[0]}
within the function, it would output item_a
.
Use case 4: Declare an associative array variable with the specified value
Code:
local -A variable=([key_a]=item_a [key_b]=item_b [key_c]=item_c)
Motivation for using the example:
An associative array allows for more advanced data correlation within a script, like a dictionary in other programming languages, connecting keys to values. This means if you have meaningful keys and need precise storage or retrieval, an associative array is integral. Keeping this layout local can avoid conflicts, particularly when handling multiple datasets that might otherwise clash in naming or structure.
Explanation:
local
: Ensures the associative array’s scope is maintained within the function.-A
: This flag sets the variable as an associative array which allows for key-value pairs.variable
: The name of the associative array.([key_a]=item_a [key_b]=item_b [key_c]=item_c)
: Represents the key-value pairs initialized with different data elements tied to specific keys.
Example output:
Using echo ${variable[key_a]}
inside the function returns item_a
.
Use case 5: Declare a readonly variable with the specified value
Code:
local -r variable="value"
Motivation for using the example:
Readonly variables are perfect when you want to ensure the integrity of a constant inside your function. This characteristic prevents changes to the variable once it is assigned, providing security for values that should not be altered once defined, ensuring operational integrity and reducing accidental errors.
Explanation:
local
: Constrains the variable to be functional only within the block’s scope.-r
: Specifies that the variable is readonly, thus immutable once initialized with a value.variable
: The name of the readonly variable."value"
: The initial (and only) value that will be assigned given its readonly status.
Example output:
Attempting to re-assign the variable within the function will result in an error, as it’s protected by readonly restrictions.
Conclusion:
The local
command is a robust toolkit for managing variable scope inside Bash functions. By using it effectively, developers can create more modular, maintainable, and error-free shell scripts. By covering use cases from simple string declarations to more intricate associative arrays and readonly constants, the local command allows for efficient variable management that aligns with shell scripting best practices.