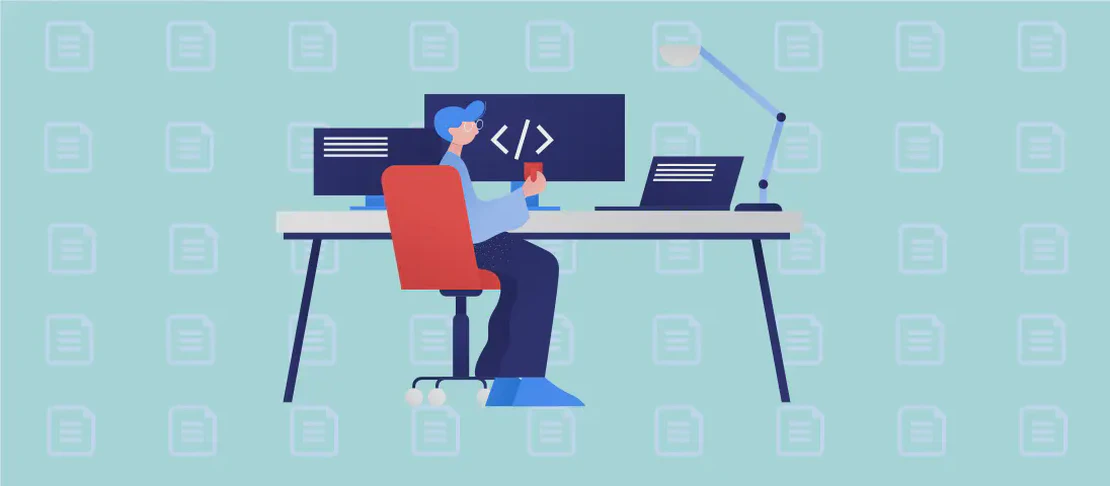
How to Use the Command 'logcat' (with Examples)
- Android
- December 17, 2024
The logcat
command is a versatile tool used primarily in the Android development environment. It is designed to stream logs of system messages, which can include everything from system stack traces during error events to information messages logged by various applications. The tool is essential for developers looking to debug applications and understand system behavior in-depth. By harnessing its power, one can sift through extensive logs to pinpoint issues or glean insights from application behavior.
Use case 1: Display System Logs
Code:
logcat
Motivation:
The simplest and most direct use of the logcat
command is to display system logs in real-time. As a developer or system administrator, you might want to inspect the live flow of system logs to monitor application behavior, detect bugs, or identify possible security issues.
Explanation:
logcat
: Invokinglogcat
without additional arguments streams all system logs to your terminal. This includes a plethora of information ranging from verbose notifications to critical error logs, providing a comprehensive look at what’s occurring on the device at any given moment.
Example Output:
I/exampleapp( 1234): Example information message
E/exampleapp( 1234): Example error message
D/exampleapp( 1234): Example debug message
Use case 2: Write System Logs to a File
Code:
logcat -f path/to/file
Motivation:
When needing to review logs later or to share them with a colleague or support team, writing them to a file is invaluable. This method ensures that logs are preserved even after a session ends, making it easier to perform more detailed analysis offline or on another device.
Explanation:
-f path/to/file
: This option tellslogcat
to write the logs to a specified file. By specifying the file path, you ensure that all logs captured during the session are saved directly to your file system for long-term storage and later use.
Example Output:
Logs will be written to path/to/file
and can be viewed using any text editor.
Use case 3: Display Lines that Match a Regular Expression
Code:
logcat --regex regular_expression
Motivation:
Sometimes, you’re only interested in logs that match specific patterns. Using regular expressions allows for fine-tuned filtering, enabling developers to identify messages of interest efficiently, such as tracking down specific error messages or monitoring lines that contain particular identifiers.
Explanation:
--regex regular_expression
: This flag allows you to specify a regular expression thatlogcat
uses to filter log lines. Only logs matching the pattern will be displayed. It serves to cut through the noise and focus exclusively on the entries that matter most to your current task.
Example Output:
Assuming a regular expression to match error logs, you’ll only see output like:
E/exampleapp( 1234): Error caused by null pointer exception
Use case 4: Display Logs for a Specific PID
Code:
logcat --pid pid
Motivation:
When diagnosing a problem related to a specific process, isolating its logs from the rest can save a significant amount of time. Monitoring logs for a particular process ID (PID) ensures that you only see relevant information from a particular application or service, simplifying troubleshooting and providing clarity on process-specific actions.
Explanation:
--pid pid
: This option enables filtering logs by the specified process ID (PID). By narrowing the logs down to a specific PID, it’s easier to focus on the behavior and interactions of one process without interference from the logs of others.
Example Output:
Assuming the PID of interest is 1234
, the logs related to this PID would look like:
I/exampleapp( 1234): Process started successfully
D/exampleapp( 1234): Processing user request
Use case 5: Display Logs for the Process of a Specific Package
Code:
logcat --pid $(pidof -s package)
Motivation:
When working with applications identified by their package name rather than PID, this method provides a mechanism to filter logs specifically for a package. It is particularly beneficial when you know the application’s package name but not its current process ID, which allows live tracing of application logs.
Explanation:
--pid $(pidof -s package)
: Here, the command uses the output frompidof -s package
to dynamically find the current PID of the running package and use it to filter logs. This combination streamlines the process of identifying logs corresponding to a specific application by leveraging the package name as an identifier.
Example Output:
Assuming the package name is com.example.app
, and its PID is determined to be 1234
, you’ll receive an output similar to:
I/com.example.app( 1234): Application initialized
E/com.example.app( 1234): Unexpected error occurred
Conclusion:
The logcat
command is an indispensable tool within the Android development sphere, offering comprehensive logging capabilities. By mastering its different use cases, including live monitoring, writing to files, regular expression filtering, and PID/package-specific logging, developers can gain detailed insights into system and application behaviors, significantly enhancing the debugging and development process.